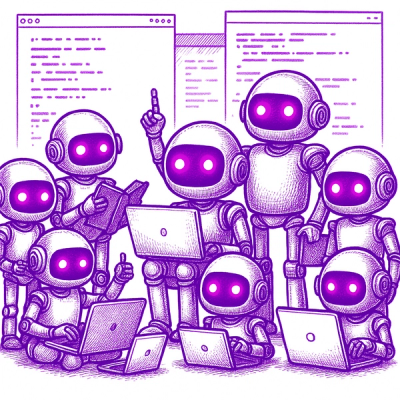
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
error-ninja
Advanced tools
Backend error handling can be a pain, it's tempting to just pass strings to callbacks so it's easier to handle, but using a proper Error object is a better way and Error Ninja can help with this.
First, define a hash of error IDs and error messages.
const ErrorNinja = require('error-ninja').define({
'invalid-blog-id': 'The given blog ID does not exist!',
'attachment-too-big': 'You cannot upload an attachment that big!',
'some-error': 'Woah, this code is buggy!',
});
Now you can create an error. You can throw this error just like any other.
const err = new ErrorNinja('invalid-blog-id');
throw err;
/*
Outputs...
Error [invalid-blog-id]: The given blog ID does not exist!
at new ErrorNinja (/path/to/Error-Ninja/errorNinja.js:36:19)
at Object.<anonymous> (/path/to/myScript.js:59:13)
at Module._compile (module.js:456:26)
at Object.Module._extensions..js (module.js:474:10)
at Module.load (module.js:356:32)
at Function.Module._load (module.js:312:12)
at Function.Module.runMain (module.js:497:10)
at startup (node.js:119:16)
at node.js:906:3
*/
If you need to include some extra properties in the error you can specify the second parameter. By default this data will be output to the console if the error is thrown, and you'll also be able to access it when handling your error.
const err = new ErrorNinja('attachment-too-big', { fileSize: 17483, maxSize: 1024, name: 'crash.log' });
throw err;
/*
Outputs...
Error [attachment-too-big]: You cannot upload an attachment that big!
----------
{"fileSize":17483,"maxSize":1024,"name":"crash.log"}
----------
at new ErrorNinja (/path/to/Error-Ninja/errorNinja.js:36:19)
at Object.<anonymous> (/path/to/myScript.js:59:13)
at Module._compile (module.js:456:26)
at Object.Module._extensions..js (module.js:474:10)
at Module.load (module.js:356:32)
at Function.Module._load (module.js:312:12)
at Function.Module.runMain (module.js:497:10)
at startup (node.js:119:16)
at node.js:906:3
*/
To prevent the extra error data being output in the console you can do one of two things:
const err = new ErrorNinja('some-error', { abc: 'do-not-output' }, false);
const ErrorNinja = require('error-ninja').define({ ... }, { outputData: false });
Apart from the usual error properties you can also access the following additional properties that should help with handling your errors.
const err = new ErrorNinja('attachment-too-big', { fileSize: 17483, maxSize: 1024, name: 'crash.log' });
err.id; // "attachment-too-big"
err.isError; // True
err.isNinja; // True
err.human; // "You cannot upload an attachment that big!"
err.data; // (mixed) e.g. { fileSize: 17483, maxSize: 1024, name: 'crash.log' }
// data defaults to {}
doSomethingAsync((err, result) => {
err = ErrorNinja.convert(err, 'my-error-id'/* , data, outputData */);
ErrorNinja.isNinja(err); // true.
});
ErrorNinja provides two methods to detect if a variable is an error, or more specifically an ErrorNinja error.
// Ordinary JavaScript errors.
const err1 = new Error();
if (ErrorNinja.isError(err1)) { ... }
// ErrorNinja errors.
const err2 = new ErrorNinja('...');
if (ErrorNinja.isNinja(err2)) { ... }
FAQs
A handy library for creating custom error constructors that work across async boundaries in Node.js.
The npm package error-ninja receives a total of 8 weekly downloads. As such, error-ninja popularity was classified as not popular.
We found that error-ninja demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.