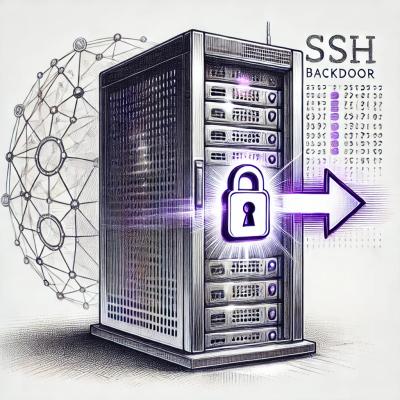
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
eventsource
Advanced tools
The eventsource npm package is a Node.js implementation of the EventSource client, which is used for receiving server-sent events (SSE). It allows clients to subscribe to a stream of updates from a server over an HTTP connection. The package is designed to be compliant with the W3C EventSource specification and is typically used for real-time data feeds, such as live notifications, stock prices updates, or any other continuous data stream.
Connecting to an SSE server
This code demonstrates how to connect to an SSE server and listen for messages. The 'onmessage' handler is called whenever a new message is received, and the 'onerror' handler is called if there's an error with the connection.
const EventSource = require('eventsource');
const es = new EventSource('http://example.com/events');
es.onmessage = function(event) {
console.log(event.data);
};
es.onerror = function(err) {
console.error('EventSource failed:', err);
};
Listening for specific event types
This code snippet shows how to listen for specific event types using the 'addEventListener' method. In this example, the client listens for 'userupdate' events and processes the received data.
const EventSource = require('eventsource');
const es = new EventSource('http://example.com/events');
es.addEventListener('userupdate', function(event) {
const userData = JSON.parse(event.data);
console.log('User update:', userData);
});
Reconnecting after connection loss
This example illustrates the automatic reconnection feature of the EventSource API. The 'onopen' handler is called when the connection is successfully established, and the 'onerror' handler indicates that the connection has been lost and a reconnection attempt will be made.
const EventSource = require('eventsource');
const es = new EventSource('http://example.com/events');
es.onopen = function() {
console.log('Connection to server opened.');
};
es.onerror = function() {
console.log('Connection lost, the browser will automatically attempt to reconnect.');
};
The sse-client package is another implementation of the EventSource API for Node.js. It provides similar functionality for connecting to SSE servers and handling server-sent events. Compared to eventsource, it may have different API nuances or additional features, but the core functionality remains the same.
Oceanwind is not a direct alternative to eventsource, but it is an example of a package that uses server-sent events to provide real-time updates to clients. It is a library for managing real-time communication between servers and clients, and it may include an SSE implementation as part of its feature set.
This library is a pure JavaScript implementation of the EventSource client. The API aims to be W3C compatible.
You can use it with Node.js or as a browser polyfill for
browsers that don't have native EventSource
support.
npm install eventsource
npm install
node ./example/sse-server.js
node ./example/sse-client.js # Node.js client
open http://localhost:8080 # Browser client - both native and polyfill
curl http://localhost:8080/sse # Enjoy the simplicity of SSE
Just add example/eventsource-polyfill.js
file to your web page:
<script src=/eventsource-polyfill.js></script>
Now you will have two global constructors:
window.EventSourcePolyfill
window.EventSource // Unchanged if browser has defined it. Otherwise, same as window.EventSourcePolyfill
If you're using webpack or browserify
you can of course build your own. (The example/eventsource-polyfill.js
is built with webpack).
You can define custom HTTP headers for the initial HTTP request. This can be useful for e.g. sending cookies
or to specify an initial Last-Event-ID
value.
HTTP headers are defined by assigning a headers
attribute to the optional eventSourceInitDict
argument:
var eventSourceInitDict = {headers: {'Cookie': 'test=test'}};
var es = new EventSource(url, eventSourceInitDict);
By default, https requests that cannot be authorized will cause the connection to fail and an exception to be emitted. You can override this behaviour, along with other https options:
var eventSourceInitDict = {https: {rejectUnauthorized: false}};
var es = new EventSource(url, eventSourceInitDict);
Note that for Node.js < v0.10.x this option has no effect - unauthorized HTTPS requests are always allowed.
Unauthorized and redirect error status codes (for example 401, 403, 301, 307) are available in the status
property in the error event.
es.onerror = function (err) {
if (err) {
if (err.status === 401 || err.status === 403) {
console.log('not authorized');
}
}
};
You can define a proxy
option for the HTTP request to be used. This is typically useful if you are behind a corporate firewall.
var es = new EventSource(url, {proxy: 'http://your.proxy.com'});
MIT-licensed. See LICENSE
FAQs
W3C compliant EventSource client for Node.js and browser (polyfill)
We found that eventsource demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.