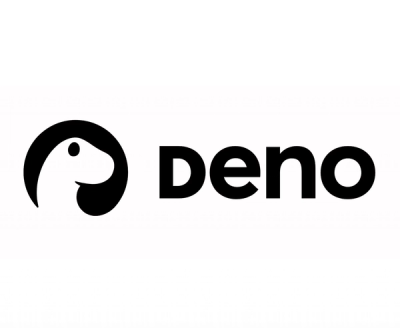
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
A small and pluggable lib to fetch a resource and cache the result.
Fetch will parse the cache-control
header. I it encounters private
, no-cache
, max-age=0
or must-revalidate
it wont cache. Otherwise
it will respect the max-age
header.
var behavior = {};
var fetch = fetchBuilder(behavior);
fetch("http://example.com/resouce.json", function (err, content) {
// Do something with the result
});
var behavior = {};
var fetch = fetchBuilder(behavior);
fetch("http://example.com/resouce.json").then(function (content) {
// Do something with the result
});
freeze
: (default:false
). When this option is set it will freeze the response so it can't be modified.cache
: (default: an instance of AsyncCache
(https://github.com/ExpressenAB/exp-asynccache). To disable caching set {cache: null}
cacheKeyFn
: (default: caches on the url) An optional formatting function for finding the cache-key. One might, for example, want to cache on an url with the get params stripped.maxAgeFn
: (default: respects the cache-control
header)onNotFound
: If given a function, it will be called each time fetch encounters a 404onError
: If given a function, it will be called each time fetch encounters a non 200 nor 404 responseonSuccess
: If given a function, it will be called each time fetch encounters a 200logger
: A logger object implementing error
, warning
, info
, debug
for example https://github.com/tj/log.jsvar keyFinder = function (url) {
return url.replace(/\//g, "");
}
var fetch = fetchBuilder({cacheKeyFn: keyFinder});
Promise.all([
fetch("http://example.com/foo/bar")
fetch("http://example.com/foobar")
]).then(function (result) {
result[0] === result[1];
});
function cacheNothing(maxAge, key, headers, content) {
return -1;
}
var fetch = fetchBuilder({maxAgeFn: cacheNothing});
FAQs
A small pluggable fetch lib
The npm package exp-fetch receives a total of 293 weekly downloads. As such, exp-fetch popularity was classified as not popular.
We found that exp-fetch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 20 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.