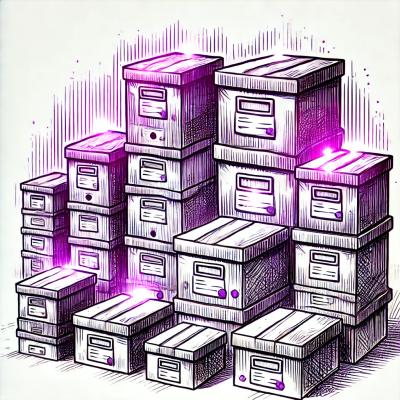
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
expo-linear-gradient
Advanced tools
The expo-linear-gradient package is a part of the Expo ecosystem and provides a way to create linear gradients in React Native applications. It is particularly useful for creating visually appealing backgrounds, buttons, and other UI elements with gradient effects.
Basic Linear Gradient
This feature allows you to create a basic linear gradient with multiple colors. The gradient is applied to a View component, and you can customize the colors and style as needed.
import { LinearGradient } from 'expo-linear-gradient';
import { View, Text } from 'react-native';
const BasicGradient = () => (
<LinearGradient
colors={['#4c669f', '#3b5998', '#192f6a']}
style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}
>
<Text style={{ color: 'white' }}>Basic Gradient</Text>
</LinearGradient>
);
Gradient with Custom Start and End Points
This feature allows you to define custom start and end points for the gradient. This can be useful for creating diagonal or other non-standard gradient directions.
import { LinearGradient } from 'expo-linear-gradient';
import { View, Text } from 'react-native';
const CustomGradient = () => (
<LinearGradient
colors={['#ff7e5f', '#feb47b']}
start={[0, 0]}
end={[1, 1]}
style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}
>
<Text style={{ color: 'white' }}>Custom Gradient</Text>
</LinearGradient>
);
Gradient with Locations
This feature allows you to specify the locations of each color in the gradient. This can be useful for creating more complex gradient effects where colors change at specific points.
import { LinearGradient } from 'expo-linear-gradient';
import { View, Text } from 'react-native';
const GradientWithLocations = () => (
<LinearGradient
colors={['#e96443', '#904e95']}
locations={[0.3, 0.7]}
style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}
>
<Text style={{ color: 'white' }}>Gradient with Locations</Text>
</LinearGradient>
);
The react-native-linear-gradient package provides similar functionality to expo-linear-gradient, allowing you to create linear gradients in React Native applications. It offers more flexibility and is not tied to the Expo ecosystem, making it a good choice for projects that do not use Expo.
The react-native-svg package allows you to create complex vector graphics, including gradients, in React Native applications. While it offers more advanced features and flexibility compared to expo-linear-gradient, it also has a steeper learning curve and is more complex to use.
Provides a React component that renders a gradient view.
For managed Expo projects, please follow the installation instructions in the API documentation for the latest stable release.
For bare React Native projects, you must ensure that you have installed and configured the expo
package before continuing.
npx expo install expo-linear-gradient
No additional set up necessary.
Run npx pod-install
after installing the npm package.
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
FAQs
Provides a React component that renders a gradient view.
The npm package expo-linear-gradient receives a total of 210,900 weekly downloads. As such, expo-linear-gradient popularity was classified as popular.
We found that expo-linear-gradient demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.