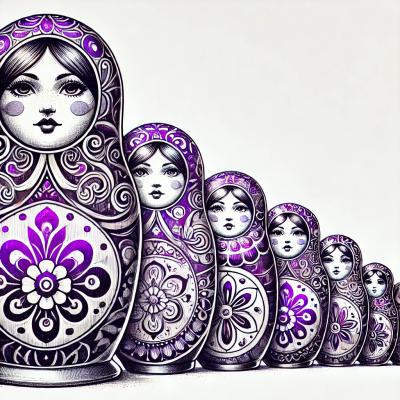
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
expo-web-browser
Advanced tools
Provides access to the system's web browser and supports handling redirects. On iOS, it uses SFSafariViewController or SFAuthenticationSession, depending on the method you call, and on Android it uses ChromeCustomTabs. As of iOS 11, SFSafariViewController
The expo-web-browser package provides an API to open web pages in a browser within an Expo app. It allows developers to open URLs in a web browser or an in-app browser, providing a seamless user experience.
Open a URL in the system's web browser
This feature allows you to open a URL in the system's default web browser. The `openBrowserAsync` function takes a URL as an argument and opens it in the browser.
import * as WebBrowser from 'expo-web-browser';
async function openBrowser() {
let result = await WebBrowser.openBrowserAsync('https://example.com');
console.log(result);
}
Open a URL in an in-app browser
This feature allows you to open a URL in an in-app browser with additional options like reader mode and bar collapsing. The `openBrowserAsync` function can take a second argument with options to customize the in-app browser.
import * as WebBrowser from 'expo-web-browser';
async function openInAppBrowser() {
let result = await WebBrowser.openBrowserAsync('https://example.com', {
readerMode: true,
enableBarCollapsing: true
});
console.log(result);
}
Dismiss the in-app browser
This feature allows you to programmatically dismiss the in-app browser. The `dismissBrowser` function can be called to close the in-app browser if it is open.
import * as WebBrowser from 'expo-web-browser';
function dismissBrowser() {
WebBrowser.dismissBrowser();
}
The react-native-inappbrowser-reborn package provides similar functionality to expo-web-browser, allowing you to open URLs in an in-app browser. It offers more customization options and supports both Android and iOS. However, it requires linking and additional setup compared to the seamless integration of expo-web-browser in Expo projects.
The react-native-webview package allows you to embed web content directly within your React Native application. Unlike expo-web-browser, which opens a separate browser window, react-native-webview provides a component to render web content inline. This package is more suitable for cases where you need to display web content as part of your app's UI.
Provides access to the system's web browser and supports handling redirects. On iOS, it uses SFSafariViewController or SFAuthenticationSession, depending on the method you call, and on Android it uses ChromeCustomTabs. As of iOS 11, SFSafariViewController no longer shares cookies with Safari, so if you are using WebBrowser for authentication you will want to use WebBrowser.openAuthSessionAsync, and if you just want to open a webpage (such as your app privacy policy), then use WebBrowser.openBrowserAsync.
For managed Expo projects, please follow the installation instructions in the API documentation for the latest stable release.
For bare React Native projects, you must ensure that you have installed and configured the expo
package before continuing.
npx expo install expo-web-browser
Run npx pod-install
after installing the npm package.
No additional set up necessary.
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
FAQs
Provides access to the system's web browser and supports handling redirects. On iOS, it uses SFSafariViewController or ASWebAuthenticationSession, depending on the method you call, and on Android it uses ChromeCustomTabs. As of iOS 11, SFSafariViewControl
The npm package expo-web-browser receives a total of 250,435 weekly downloads. As such, expo-web-browser popularity was classified as popular.
We found that expo-web-browser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.