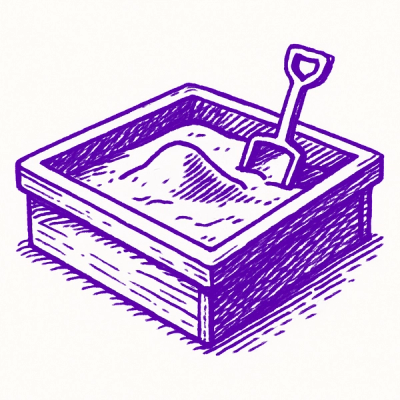
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
export-to-csv
Advanced tools
The export-to-csv npm package is a utility that allows you to easily export data to CSV format. It is particularly useful for converting JSON data into CSV files, which can then be downloaded or used in other applications.
Basic CSV Export
This feature allows you to export a basic JSON array to a CSV file. The code sample demonstrates how to configure the export options and generate the CSV data.
const { ExportToCsv } = require('export-to-csv');
const data = [
{ name: 'John', age: 30, city: 'New York' },
{ name: 'Jane', age: 25, city: 'San Francisco' }
];
const options = {
fieldSeparator: ',',
quoteStrings: '"',
decimalSeparator: '.',
showLabels: true,
showTitle: true,
title: 'My CSV File',
useTextFile: false,
useBom: true,
useKeysAsHeaders: true
};
const csvExporter = new ExportToCsv(options);
const csvData = csvExporter.generateCsv(data);
console.log(csvData);
Custom Headers
This feature allows you to specify custom headers for the CSV file. The code sample shows how to set the headers option to define the column names in the CSV output.
const { ExportToCsv } = require('export-to-csv');
const data = [
{ name: 'John', age: 30, city: 'New York' },
{ name: 'Jane', age: 25, city: 'San Francisco' }
];
const options = {
fieldSeparator: ',',
quoteStrings: '"',
decimalSeparator: '.',
showLabels: true,
showTitle: true,
title: 'My CSV File',
useTextFile: false,
useBom: true,
headers: ['Name', 'Age', 'City']
};
const csvExporter = new ExportToCsv(options);
const csvData = csvExporter.generateCsv(data);
console.log(csvData);
Download CSV File
This feature allows you to download the generated CSV data as a file. The code sample demonstrates how to use the 'fs' module to write the CSV data to a file.
const { ExportToCsv } = require('export-to-csv');
const fs = require('fs');
const data = [
{ name: 'John', age: 30, city: 'New York' },
{ name: 'Jane', age: 25, city: 'San Francisco' }
];
const options = {
fieldSeparator: ',',
quoteStrings: '"',
decimalSeparator: '.',
showLabels: true,
showTitle: true,
title: 'My CSV File',
useTextFile: false,
useBom: true,
useKeysAsHeaders: true
};
const csvExporter = new ExportToCsv(options);
const csvData = csvExporter.generateCsv(data);
fs.writeFileSync('my_csv_file.csv', csvData);
The json2csv package is another popular library for converting JSON data to CSV format. It offers a wide range of customization options and supports both synchronous and asynchronous operations. Compared to export-to-csv, json2csv provides more advanced features such as custom field transformations and multi-level JSON support.
The csv-writer package is a simple and easy-to-use library for writing CSV files. It allows you to define the structure of the CSV file and write data to it in a straightforward manner. While it may not offer as many customization options as export-to-csv, it is a good choice for basic CSV writing tasks.
The fast-csv package is a fast and efficient library for parsing and formatting CSV data. It supports both reading and writing CSV files and offers a range of configuration options. Compared to export-to-csv, fast-csv is more focused on performance and is suitable for handling large datasets.
Like this library and want to support active development?
Small, simple, and single purpose. Zero dependencies, functionally inspired, and fairly well-typed.
If you're looking for a fully CSV-compliant, consistently maintained, whole-package library, I'd recommend looking elsewhere! (see alternatives section below)
If you want a lightweight, stable, easy-to-use basic CSV generation and download library, feel free to install.
npm install --save export-to-csv
This library was written with TypeScript in mind, so the examples will be in TS.
You can easily use this library in JavaScript as well. The bundle uses ES modules, which all modern browsers support.
You can also look at the integration tests for browser/JS use, and the unit tests to understand how the library functions.
import { mkConfig, generateCsv, download } from "export-to-csv";
// mkConfig merges your options with the defaults
// and returns WithDefaults<ConfigOptions>
const csvConfig = mkConfig({ useKeysAsHeaders: true });
const mockData = [
{
name: "Rouky",
date: "2023-09-01",
percentage: 0.4,
quoted: '"Pickles"',
},
{
name: "Keiko",
date: "2023-09-01",
percentage: 0.9,
quoted: '"Cactus"',
},
];
// Converts your Array<Object> to a CsvOutput string based on the configs
const csv = generateCsv(csvConfig)(mockData);
// Get the button in your HTML
const csvBtn = document.querySelector("#csv");
// Add a click handler that will run the `download` function.
// `download` takes `csvConfig` and the generated `CsvOutput`
// from `generateCsv`.
csvBtn.addEventListener("click", () => download(csvConfig)(csv));
import { mkConfig, generateCsv, asString } from "export-to-csv";
import { writeFile } from "node:fs";
import { Buffer } from "node:buffer";
// mkConfig merges your options with the defaults
// and returns WithDefaults<ConfigOptions>
const csvConfig = mkConfig({ useKeysAsHeaders: true });
const mockData = [
{
name: "Rouky",
date: "2023-09-01",
percentage: 0.4,
quoted: '"Pickles"',
},
{
name: "Keiko",
date: "2023-09-01",
percentage: 0.9,
quoted: '"Cactus"',
},
];
// Converts your Array<Object> to a CsvOutput string based on the configs
const csv = generateCsv(csvConfig)(mockData);
const filename = `${csvConfig.filename}.csv`;
const csvBuffer = new Uint8Array(Buffer.from(asString(csv)));
// Write the csv file to disk
writeFile(filename, csvBuffer, (err) => {
if (err) throw err;
console.log("file saved: ", filename);
});
generateCsv
output as a string
Note: this is only applicable to projects using Typescript. If you're using this library with Javascript, you might not run into this issue.
There might be instances where you want to use the result from generateCsv
as a string
instead of a CsvOutput
type. To do that, you can use asString
, which is exported from this library.
import { mkConfig, generateCsv, asString } from "export-to-csv";
const csvConfig = mkConfig({ useKeysAsHeaders: true });
const addNewLine = (s: string): string => s + "\n";
const mockData = [
{
name: "Rouky",
date: "2023-09-01",
percentage: 0.4,
quoted: '"Pickles"',
},
{
name: "Keiko",
date: "2023-09-01",
percentage: 0.9,
quoted: '"Cactus"',
},
];
// Converts your Array<Object> to a CsvOutput string based on the configs
const csvOutput = generateCsv(csvConfig)(mockData);
// This would result in a type error
// const csvOutputWithNewLine = addNewLine(csvOutput);
// ❌ => CsvOutput is not assignable to type string.
// This unpacks CsvOutput which turns it into a string before use
const csvOutputWithNewLine = addNewLine(asString(csvOutput));
The reason the CsvOutput
type exists is to prevent accidentally passing in a string which wasn't formatted by generateCsv
to the download
function.
generateCsv
output as a Blob
A case for this would be using browser extension download methods instead of the supplied download
function. There may be scenarios where using a Blob
might be more ergonomic.
import { mkConfig, generateCsv, asBlob } from "export-to-csv";
// mkConfig merges your options with the defaults
// and returns WithDefaults<ConfigOptions>
const csvConfig = mkConfig({ useKeysAsHeaders: true });
const mockData = [
{
name: "Rouky",
date: "2023-09-01",
percentage: 0.4,
quoted: '"Pickles"',
},
{
name: "Keiko",
date: "2023-09-01",
percentage: 0.9,
quoted: '"Cactus"',
},
];
// Converts your Array<Object> to a CsvOutput string based on the configs
const csv = generateCsv(csvConfig)(mockData);
// Generate the Blob from the CsvOutput
const blob = asBlob(csvConfig)(csv);
// Requires URL to be available (web workers or client scripts only)
const url = URL.createObjectURL(blob);
// Assuming there's a button with an id of csv in the DOM
const csvBtn = document.querySelector("#csv");
csvBtn.addEventListener("click", () => {
// Use Chrome's downloads API for extensions
chrome.downloads.download({
url,
body: csv,
filename: "chrome-extension-output.csv",
});
});
Option | Default | Type | Description |
---|---|---|---|
fieldSeparator | "," | string | Defines the field separator character |
filename | "generated" | string | Sets the name of the file created from the download function |
quoteStrings | false | boolean | Determines whether or not to quote strings (using quoteCharacter 's value). Whether or not this is set, \r , \n , and fieldSeparator will be quoted. |
quoteCharacter | '"' | string | Sets the quote character to use. |
decimalSeparator | "." | string | Defines the decimal separator character (default is .). If set to "locale", it uses the language-sensitive representation of the number. |
showTitle | false | boolean | Sets whether or not to add the value of title to the start of the CSV. (This is not supported by all CSV readers) |
title | "My Generated Report" | string | The title to display as the first line of the CSV file. (This is not the name of the file [see filename ]) |
showColumnHeaders | true | boolean | Determines if columns should have headers. When set to false , the first row of the CSV will be data. |
columnHeaders | [] | Array<string | {key: string, displayLabel: string}> | Use this option if column/header order is important! Determines the headers to use as the first line of the CSV data. If the item is a string , it will be used for lookup in your collection AND as the header label. If the item is an object, key will be used for lookup, and displayLabel will be used as the header label. |
useKeysAsHeaders | false | boolean | If set, the CSV will use the key names in your collection as headers. Warning: headers recommended for large collections. If set, it'll override the headers option. Column/header order also not guaranteed. Use headers only if order is important! |
boolDisplay | {true: "TRUE", false: "FALSE"} | {true: string, false: string} | Determines how to display boolean values in the CSV. This only works for true and false . 1 and 0 will not be coerced and will display as 1 and 0 . |
useBom | true | boolean | Adds a byte order mark which is required by Excel to display CSVs, despite it not being necessary with UTF-8 🤷♂️ |
useTextFile | false | boolean | Deprecation warning. This will be removed in the next major version. Will download the file as text/plain instead of text/csv and use a .txt vs .csv file extension. |
fileExtension | csv | string | File extension to use. Currently, this only applies if useTextFile is false . |
As mentioned above, this library is intentionally small and was designed to solve a very simple need. It was not originally designed to be fully CSV compliant, so many things you need might be missing. I'm also not the most active on it (~7 year gap between updates). So, here are some alternatives with more support and that might be more fully featured.
This library was originally based on this library by Javier Telio
Credits and Original Authors |
---|
javiertelioz |
sn123 |
arf1980 |
FAQs
Easily create CSV data from json collection
The npm package export-to-csv receives a total of 204,124 weekly downloads. As such, export-to-csv popularity was classified as popular.
We found that export-to-csv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.