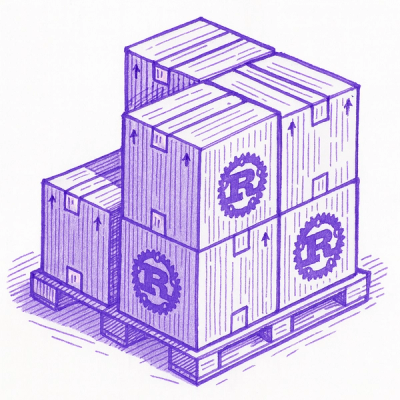
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
express-authenticators
Advanced tools
Third party authenticators in nodejs. Support various providers. Zero heavy dependencies.
Third party authenticator module re-written in Typescript
yarn
: yarn add express-authenticators
.npm
: npm install --save express-authenticators
.
const {
FacebookAuthenticator,
FoursquareAuthenticator,
GithubAuthenticator,
GoogleAuthenticator,
InstagramAuthenticator,
LinkedInAuthenticator,
PinterestAuthenticator,
TumblrAuthenticator,
TwitterAuthenticator,
OAuth2,
OAuth
} = require('express-authenticators')
const express = require('express')
const session = require('express-session')
const asyncMiddleware = require('middleware-async')
const app = express()
app.use(session())
const facebookAuth = new FacebookAuthenticator({
clientID: 'facebook app id',
clientSecret: 'facebook app secret',
redirectUri: `https://example.com/auth/facebook/callback`,
})
app.get(
'/auth/facebook',
(req, res, next) => {
req.session!.someInfo = 'my info' // store the user credential
facebookAuth.authenticate(req, res, next)
}
/*
or
app.get('/auth/facebook', facebookAuth.authenticate)
*/
)
app.get(
`/auth/facebook/callback`,
asyncMiddleware(async (req, res) => {
const token = await facebookAuth.callback(req)
const profile = await facebookAuth.fetchProfile(token)
console.log('got profile', profile)
res.send(JSON.stringify(profile))
})
)
This module requires express-session
middleware to be applied before.
Exported classes:
FacebookAuthenticator
, FoursquareAuthenticator
, GithubAuthenticator
, GoogleAuthenticator
, InstagramAuthenticator
, LinkedInAuthenticator
, PinterestAuthenticator
, TumblrAuthenticator
, TwitterAuthenticator
.
All these classes have a same interface, they all inherit OAuth2 or OAuth classes
constructor(option: {
clientID: 'app id',
clientSecret: 'app secret',
redirectUri: `https://example.com/auth/<provider>/callback`
})
authenticate(req: Request, res: Response): Promise<void> | void // async middleware
callback(req: Request): Promise<TokenType> | TokenType
fetchProfile(tokenSet: TokenType): Promise<{
id?: string
email?: string
emailVerified?: boolean
first?: string
last?: string
avatar?: string
raw: any
}>
authenticate
: redirect middleware
If there is no error, this middleware will redirect the user to the provider website.
Eventually, redirect back to our pre-configured redirectUri
with appropriate user privilege.
callback
: take the request and return a promise which returns a token for profile fetching.
This function should be called in the callback url handler. Check example at the end of this readme.
fetchProfile
: provider-specific profile fetcher using the token returned by callback
.
OAuth2
, OAuth
In principle these classes have authenticate
and callback
methods.
However, I recommend you use the provider-specific classes described above.
If you need an additional provider, clone the other providers' implementations then make your own.
Here are two sample implementations of FacebookAuthenticator
(OAuth2
), and TwitterAuthenticator
(OAuth
)
class FacebookAuthenticator extends OAuth2 implements IOAuthProfileFetcher<string> {
fetchProfile = fetchFacebookProfile //make your own profile fetcher
constructor(options: {
clientID: string
clientSecret: string
redirectUri: string
scope?: string
}) {
super({
consentURL: 'https://www.facebook.com/v6.0/dialog/oauth',
tokenURL: 'https://graph.facebook.com/v6.0/oauth/access_token',
scope: ['email'].join(','),
...options,
}, {
ignoreGrantType: true,
tokenRequestMethod: TokenRequestMethod.GET,
includeStateInAccessToken: false
})
}
}
class TwitterAuthenticator extends OAuth implements IOAuthProfileFetcher<IOAuthTokenSet> {
constructor(config: {
clientID: string
clientSecret: string
redirectUri: string
}) {
super({
consumerKey: config.clientID,
consumerSecret: config.clientSecret,
callbackUrl: config.redirectUri,
requestTokenUrl: 'https://api.twitter.com/oauth/request_token',
accessTokenUrl: 'https://api.twitter.com/oauth/access_token',
authorizeUrl: 'https://api.twitter.com/oauth/authorize',
signingMethod: OAuthSigningMethod.Hmac,
})
}
async fetchProfile(tokenSet: IOAuthTokenSet){
//make use of this.signAndFetch and make your own profile fetcher
const response = await this.signAndFetch(
'https://api.twitter.com/1.1/account/verify_credentials.json',
{
qs: { include_email: true},
},
tokenSet
)
if (!response.ok) throw new OAuthProfileError(await response.text())
const profile = await response.json()
if (!profile.id_str) throw new OAuthProfileError('Invalid Twitter profile ID')
return {
id: profile.id_str,
raw: profile,
avatar: profile.profile_image_url_https
|| profile.profile_image_url
|| profile.profile_background_image_url_https
|| profile.profile_background_image_url,
first: profile.name || profile.screen_name,
email: profile.email,
emailVerified: !!profile.email,
/**
* from twitter docs
* https://developer.twitter.com/en/docs/accounts-and-users/manage-account-settings/api-reference/get-account-verify_credentials
* When set to true email will be returned in the user objects as a string. If the user does not have an email address on their account, or if the email address is not verified, null will be returned.
*/
}
}
}
FAQs
Third party authenticators in nodejs. Support various providers. Almost zero dependencies.
The npm package express-authenticators receives a total of 10 weekly downloads. As such, express-authenticators popularity was classified as not popular.
We found that express-authenticators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.