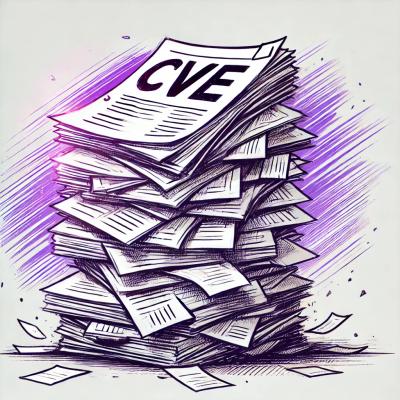
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
express-validator
Advanced tools
express-validator is a set of express.js middlewares that wraps validator.js, a library for string validation and sanitization. It provides a comprehensive set of validation and sanitization middlewares for handling user input in express applications.
Validation
This feature allows you to validate user input. In this example, the 'username' field must be alphanumeric and the 'password' field must be at least 5 characters long. If the validation fails, a 400 status code with the validation errors is returned.
const { body, validationResult } = require('express-validator');
app.post('/user', [
body('username').isAlphanumeric(),
body('password').isLength({ min: 5 })
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
res.send('User is valid');
});
Sanitization
This feature allows you to sanitize user input. In this example, the 'email' field is normalized to a standard email format and the 'username' field is trimmed of whitespace and escaped to prevent HTML injection.
const { body } = require('express-validator');
app.post('/user', [
body('email').normalizeEmail(),
body('username').trim().escape()
], (req, res) => {
res.send('User input is sanitized');
});
Custom Validators
This feature allows you to create custom validation logic. In this example, the 'age' field must be at least 18. If the validation fails, a 400 status code with the validation errors is returned.
const { body, validationResult } = require('express-validator');
app.post('/user', [
body('age').custom(value => {
if (value < 18) {
throw new Error('Age must be at least 18');
}
return true;
})
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
res.send('User is valid');
});
Joi is a powerful schema description language and data validator for JavaScript. It allows you to create blueprints or schemas for JavaScript objects to ensure validation of key information. Compared to express-validator, Joi is more focused on schema-based validation and is not tied to express.js.
Yup is a JavaScript schema builder for value parsing and validation. It is similar to Joi but is more lightweight and has a more modern API. Like Joi, Yup is not tied to express.js and can be used in various JavaScript environments.
Validator is a library of string validators and sanitizers. It is the underlying library used by express-validator for its validation and sanitization functions. While it provides a comprehensive set of validation and sanitization functions, it does not provide middleware for express.js out of the box.
An express.js middleware for validator.
npm install express-validator
Also make sure that you have Node.js 14 or newer in order to use it.
Please refer to the documentation website on https://express-validator.github.io.
Check the GitHub Releases page.
MIT License
FAQs
Express middleware for the validator module.
The npm package express-validator receives a total of 461,644 weekly downloads. As such, express-validator popularity was classified as popular.
We found that express-validator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.