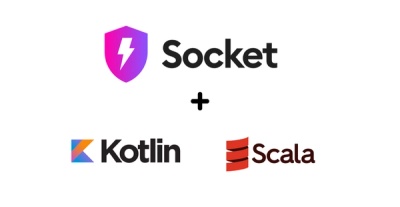
Product
Introducing Scala and Kotlin Support in Socket
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
facebook-bot-messenger
Advanced tools
==
SDK of the Facebook Messenger Platform for Node.js
The Facebook Messenger Platform SDK can be installed with NPM.
$ npm install facebook-bot-messenger
var MessengerPlatform = require('facebook-bot-messenger');
sample is following
var bot = MessengerPlatform.create({
pageID: '<your page id>',
appID: '<your app id>',
appSecret: '<your app secret>',
validationToken: '<your validation token>',
pageToken: '<your page token>'
});
bot.webhook('/webhook');
bot.on(MessengerPlatform.Events.MESSAGE, function(userId, message) {
// add code below.
});
bot.listen(8080);
var server = require('http').createServer(handler);
var bot = MessengerPlatform.create({
pageID: '<your page id>',
appID: '<your app id>',
appSecret: '<your app secret>',
validationToken: '<your validation token>',
pageToken: '<your page token>'
}, server);
bot.webhook('/webhook');
bot.on(MessengerPlatform.Events.MESSAGE, function(userId, message) {
// add code below.
});
server.listen(8080);
var app = require('express')();
var server = require('http').Server(app);
var bot = MessengerPlatform.create({
pageID: '<your page id>',
appID: '<your app id>',
appSecret: '<your app secret>',
validationToken: '<your validation token>',
pageToken: '<your page token>'
}, server);
app.use(bot.webhook('/webhook'));
bot.on(MessengerPlatform.Events.MESSAGE, function(userId, message) {
// add code below.
});
server.listen(8080);
Instance of bot client is a handler of the Messenger Platform.
var bot = MessengerPlatform.create({
pageID: '<your page id>',
appID: '<your app id>',
appSecret: '<your app secret>',
validationToken: '<your validation token>',
pageToken: '<your page token>'
});
You can call API through the bot client instance.
Get detail information of user.
bot.getProfile('<user id>').then(function(data) {
// add your code when success.
}).catch(function(error) {
// add your code when error.
});
When MessengerPlatform#getProfile() success return JSON object.
Send readed message
bot.sendReadedAction('<user id>');
Send typing on
bot.sendTypingAction('<user id>');
Send typing off
bot.sendClearTypingAction('<user id>');
sample is following
bot.sendTextMessage('<user id>', '<message>');
This procedure sends a message to the destination that is associated with .
More advanced sample is below;
var textMessageBuilder = new MessengerPlatform.TextMessageBuilder('<message>');
bot.sendMessage('<user id>', textMessageBuilder);
And other send method;
Send image attachment message
bot.sendImageMessage('<user id>', '<url>', '<is reusable');
Send audio attachment message
bot.sendAudioMessage('<user id>', '<url>', '<is reusable');
Send video attachment message
bot.sendVideoMessage('<user id>', '<url>', '<is reusable');
Send file attachment message
bot.sendFileMessage('<user id>', '<url>', '<is reusable');
Type of message depends on the type of instance of MessageBuilder. That means this method sends text message if you pass TextMessageBuilder
The type of instance of MessageBuilder
TextMessageBuilder
var builder = new MessengerPlatform.TextMessageBuilder('<message>');
QuickRepliesMessageBuilder
var builder = new MessengerPlatform.QuickRepliesMessageBuilder('Pick a color:');
builder.addImageOption('Red', 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_RED', 'http://petersfantastichats.com/img/red.png')
.addImageOption('Green', 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_GREEN', 'http://petersfantastichats.com/img/green.png');
AttachmentMessageBuilder
var builder = new MessengerPlatform.AttachmentMessageBuilder();
builder.addImageAttachment('<url>', '<is reusable>');
Template attachment message:
var element = new MessengerPlatform.GenericElementTemplateBuilder('Welcome to Peter\'s Hats', 'https://petersfancybrownhats.com', 'https://petersfancybrownhats.com/company_image.png', 'We\'ve got the right hat for everyone.');
element1.addButton(new MessengerPlatform.URLButtonBuilder('View Website', 'https://petersfancybrownhats.com'));
var template = new MessengerPlatform.GenericTemplateBuilder();
template.addElement(element);
var builder = new MessengerPlatform.AttachmentMessageBuilder(template);
var btnUrl = new MessengerPlatform.URLButtonBuilder('Show Website', 'https://petersapparel.parseapp.com');
var btnPostback = new MessengerPlatform.PostbackButtonBuilder('Start Chatting', 'DEVELOPER_DEFINED_PAYLOAD');
var template = new MessengerPlatform.ButtonTemplateBuilder('What do you want to do next?', [btnUrl, btnPostback]);
var builder = new MessengerPlatform.AttachmentMessageBuilder(template);
var element1 = new MessengerPlatform.ListElementTemplateBuilder('Classic Black T-Shirt', 'https://peterssendreceiveapp.ngrok.io/img/black-t-shirt.png', '100% Cotton, 200% Comfortable');
element1.setDefaultAction('https://peterssendreceiveapp.ngrok.io/view?item=102', 'https://peterssendreceiveapp.ngrok.io/', true, MessengerPlatform.WebviewHeight.TALL)
.addURLButton('Buy', 'https://peterssendreceiveapp.ngrok.io/view?item=102');
var element2 = new MessengerPlatform.ListElementTemplateBuilder('Classic Gray T-Shirt', 'https://peterssendreceiveapp.ngrok.io/img/gray-t-shirt.png', '100% Cotton, 200% Comfortable');
element2.setDefaultAction('https://peterssendreceiveapp.ngrok.io/view?item=103', 'https://peterssendreceiveapp.ngrok.io/', true, MessengerPlatform.WebviewHeight.TALL)
.addURLButton('Buy', 'https://peterssendreceiveapp.ngrok.io/shop?item=103');
var template = new MessengerPlatform.ListTemplateBuilder();
template.isLargeTopElement(true)
.addElement(element1)
.addElement(element2)
.addPostbackButton('View More', 'USER_DEFINED_PAYLOAD');
var builder = new MessengerPlatform.AttachmentMessageBuilder(template);
var template = new MessengerPlatform.ReceiptTemplateBuilder('Stephane Crozatier', '12345678902', 'USD', 'Visa 2345');
template.setOrderUrl('http://petersapparel.parseapp.com/order?order_id=123456')
.setTimestamp('1428444852')
.addElement('Classic White T-Shirt', 50, 'http://petersapparel.parseapp.com/img/whiteshirt.png', 2, '100% Soft and Luxurious Cotton', 'USD')
.addElement('Classic Gray T-Shirt', 25, 'http://petersapparel.parseapp.com/img/grayshirt.png', 1, '100% Soft and Luxurious Cotton', 'USD')
.setAddress(['1 Hacker Way'], 'Menlo Park', 'CA', '94025', 'US')
.setSummary('56.14', '75.00', '4.95', '6.19')
.addAdjustment('New Customer Discount', 20)
.addAdjustment('$10 Off Coupon', 10);
var builder = new MessengerPlatform.AttachmentMessageBuilder(template);
Facebook's server sends user action (message, message delivered, message read and etc.) to your bot server. Request of that contains event(s); event is action of the user.
Webhook events:
FAQs
SDK of the Facebook messenger platform for Node.js
The npm package facebook-bot-messenger receives a total of 2 weekly downloads. As such, facebook-bot-messenger popularity was classified as not popular.
We found that facebook-bot-messenger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.