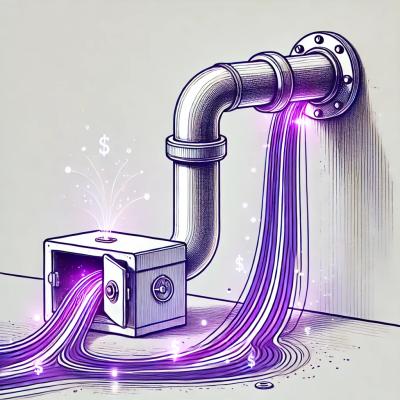
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
fast-sha256
Advanced tools
SHA-256, HMAC and PBKDF2 implementation with typed arrays for modern browsers and Node.js
The fast-sha256 npm package is a JavaScript implementation of the SHA-256 hash function. It is designed to be fast and efficient, making it suitable for use in environments where performance is critical.
Hashing a string
This feature allows you to hash a string using the SHA-256 algorithm. The code sample demonstrates how to encode a string into a Uint8Array, hash it, and then convert the hash value to a hexadecimal string.
const { hash } = require('fast-sha256');
const encoder = new TextEncoder();
const data = encoder.encode('Hello, world!');
const hashValue = hash(data);
console.log(Buffer.from(hashValue).toString('hex'));
Hashing a file
This feature allows you to hash the contents of a file using the SHA-256 algorithm. The code sample demonstrates how to read a file into a buffer, hash it, and then convert the hash value to a hexadecimal string.
const { hash } = require('fast-sha256');
const fs = require('fs');
const data = fs.readFileSync('path/to/file');
const hashValue = hash(data);
console.log(Buffer.from(hashValue).toString('hex'));
Incremental hashing
This feature allows you to perform incremental hashing, which is useful for hashing data that is received in chunks. The code sample demonstrates how to create a Hash instance, update it with multiple chunks of data, and then obtain the final hash value.
const { Hash } = require('fast-sha256');
const hash = new Hash();
hash.update(new TextEncoder().encode('Hello, '));
hash.update(new TextEncoder().encode('world!'));
const hashValue = hash.digest();
console.log(Buffer.from(hashValue).toString('hex'));
The 'crypto' module is a built-in Node.js module that provides cryptographic functionality, including the SHA-256 hash function. It is highly optimized and widely used. Compared to fast-sha256, the 'crypto' module is more versatile and includes a broader range of cryptographic functions.
The 'js-sha256' package is a JavaScript implementation of the SHA-256 hash function. It is designed to be fast and efficient, similar to fast-sha256. However, 'js-sha256' is more focused on providing a simple API for hashing strings and buffers, whereas fast-sha256 also supports incremental hashing.
The 'sha.js' package is a JavaScript implementation of the SHA family of hash functions, including SHA-256. It is designed to be fast and compatible with both Node.js and browser environments. Compared to fast-sha256, 'sha.js' offers a wider range of hash functions but may not be as optimized for performance.
SHA-256 implementation for JavaScript/TypeScript with typed arrays that works in modern browsers and Node.js. Implements the hash function, HMAC, and PBKDF2.
Public domain. No warranty.
You can install fast-sha256-js via NPM:
$ npm install fast-sha256
Functions accept and return Uint8Array
s.
To convert strings, use external library (for example,
nacl.util).
Returns a SHA-256 hash of the message.
Returns an HMAC-SHA-256 of the message for the key.
Returns a key of length dkLen derived using PBKDF2-HMAC-SHA256 from the given password, salt, and the number of rounds.
Returns a key of the given length derived using HKDF as described in RFC 5869.
There are also classes Hash
and HMAC
:
Constructor for hash instance. Should be used with new
.
Available methods: update()
, digest()
, reset()
, etc.
Constructor for HMAC instance. Should be used with new
.
Available methods: update()
, digest()
, reset()
, etc.
See comments in src/sha256.ts
for details.
import sha256, { Hash, HMAC } from "fast-sha256";
sha256(data) // default export is hash
const h = new HMAC(key); // also Hash and HMAC classes
const mac = h.update(data).digest();
// alternatively:
import * as sha256 from "fast-sha256";
sha256.pbkdf2(password, salt, iterations, dkLen); // returns derived key
sha256.hash(data)
const hasher = new sha256.Hash();
hasher.update(data1);
hasher.update(data2);
const result = hasher.digest();
Install development dependencies:
$ npm install
Build JavaScript, minified version, and typings:
$ npm run build
Run tests:
$ npm test
Run tests on a different source file:
$ SHA256_SRC=sha256.min.js npm test
Run benchmark:
$ npm run bench
(or in a browser, open tests/bench.html
).
Lint:
$ npm run lint
While this implementation is pretty fast compared to previous generation implementations, if you need an even faster one, check out asmCrypto.
FAQs
SHA-256, HMAC and PBKDF2 implementation with typed arrays for modern browsers and Node.js
The npm package fast-sha256 receives a total of 238,636 weekly downloads. As such, fast-sha256 popularity was classified as popular.
We found that fast-sha256 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.