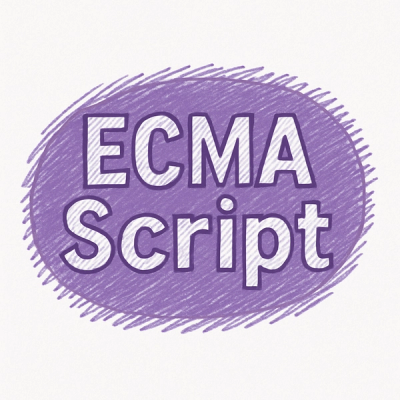
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
fb-messenger-sdk
Advanced tools
NodeJS Facebook Messenger API for bots to send messages and setup events to Facebook.
npm install fb-messenger-sdk
Import
const facebook = require('fb-messenger-sdk');
or
import { FacebookMessagingAPIClient, etc... } from 'fb-messenger-sdk';
Initialize
const messageClient = new facebook.FacebookMessagingAPIClient(process.env.PAGE_ACCESS_TOKEN);
or
const messageClient = new FacebookMessagingAPIClient(process.env.PAGE_ACCESS_TOKEN);
Using proxy
const messageClient = new facebook.FacebookMessagingAPIClient(process.env.PAGE_ACCESS_TOKEN, { hostname:process.env.PROXY_HOST, port: process.env.PROXY_PORT });
or
const messageClient = new FacebookMessagingAPIClient(process.env.PAGE_ACCESS_TOKEN, { hostname:process.env.PROXY_HOST, port: process.env.PROXY_PORT });
Defaults to http
if no protocol provided
messageClient.sendTextMessage(senderId, <MESSAGE>)
.then((result) => ...)
or
await messageClient.sentTextMessage(senderId, <MESSAGE>);
messageClient.sendImageMessage(senderId, <IMAGE_URL>)
.then((result) => ...)
or
const result = await messageClient.sendImageMessage(senderId, <IMAGE_URL>)
This method will have the image cached by facebook so every receiver after the first will get it quickly.
messageClient.sendButtonsMessage(senderId, <BUTTONS TEXT> [<ARRAY OF BUTTONS>])
.then((result) => ...)
or
const result = await messageClient.sendButtonsMessage(senderId, <BUTTONS TEXT> [<ARRAY OF BUTTONS>])
messageClient.sendQuickReplyMessage(senderId, <TEXT>, [<QUICK_REPLIES>])
.then((result) => ...)
or
const result = await messageClient.sendQuickReplyMessage(senderId, <TEXT>, [<QUICK_REPLIES>])
messageClient.sendGenericTemplate(senderId, [ELEMENTS])
.then((result) => ...)
or
const result = await messageClient.sendGenericTemplate(senderId, [ELEMENTS])
Generic Template element format
messageClient.sendListMessage(senderId, [ELEMENTS], <firstElementStyle>, [FINAL_BUTTONS])
.then((result) => ...)
or
const result = await messageClient.sendListMessage(senderId, [ELEMENTS], <firstElementStyle>, [FINAL_BUTTONS])
firstElementStyle
is optional. If not provided defaults to large
messageClient.markSeen(senderId);
As per all methods, callback can be provided. If no callback provided returns promise. Recommended to send and continue processing without waiting for reply.
messageClient.toggleTyping(senderId, <true/false>);
As per all methods, callback can be provided. If no callback provided returns promise. Recommended to send and continue processing without waiting for reply.
Defaults to false
if no boolean parameter provided.
messageClient.getUserProfile(senderId,[<PROPERTIES>])
.then((result) => ...)
or
const result = await messageClient.getUserProfile(senderId,[<PROPERTIES>])
Valid properties: first_name
,last_name
,profile_pic
,locale
,timezone
,gender
,is_payment_enabled
,last_ad_referral
If none are given defaults to first_name
only.
Extracts all relevant & known message types that can be found here. Returns array with all objects of interest. Left flexibility to user to filter out message types of interest per use case instead of returning dictionary object with each message type as a separate list for optional performance saving in case of usage on time sensitive platforms (AWS Lambda, AF, GCF, etc).
import {FacebookMessageParser} from 'fb-messenger-sdk';
const messages = FacebookMessageParser.parsePayload(incomingPayload);
Initialize
const profileClient = new facebook.FacebookProfileAPIClient(process.env.PAGE_ACCESS_TOKEN);
or
import {Profile} from 'fb-messenger-sdk';
const profileClient = new FacebookProfileAPIClient(process.env.PAGE_ACCESS_TOKEN);
Using proxy
const profileClient = new facebook.FacebookProfileAPIClient(process.env.PAGE_ACCESS_TOKEN, { hostname:process.env.PROXY_HOST, port: process.env.PROXY_PORT });
profileClient.setGreetingMessage('Message that will be visible first thing when opening chat window with your bot/page')
.then((result) => ...)
or
const result = await profileClient.setGreetingMessage('Message that will be visible first thing when opening chat window with your bot/page');
profileClient.setGetStartedAction(senderId, payload)
.then((result) => ...)
or
const result = await profileClient.setGetStartedAction(senderId, payload)
payload
is the value that will be first sent when new user sends first message, once per user interaction
profileClient.setPersistentMenu(senderId, [<MENU_ENTRIES>])
.then((result) => ...)
or
const result = await profileClient.setPersistentMenu(senderId, [<MENU_ENTRIES>])
This is a burger menu appearing next to the chat input field where users can click and get direct interaction shortcuts to specific functionality of the bot. Persistent menu format
Initialize
const pageClient = new facebook.FacebookPageAPIClient(process.env.PAGE_ID, process.env.PAGE_ACCESS_TOKEN);
or
import {FacebookPageAPIClient} from 'fb-messenger-sdk';
const pageClient = new FacebookPageAPIClient(process.env.PAGE_ID, process.env.PAGE_ACCESS_TOKEN)
Using proxy
const pageClient = new facebook.FacebookPageAPIClient(process.env.PAGE_ID, process.env.PAGE_ACCESS_TOKEN, { hostname:process.env.PROXY_HOST, port: process.env.PROXY_PORT });
Defaults to http
if no protocol provided
Requires a never expiring publishing_actions
token that can be obtained by following this guide.
pageClient.imageUrl(`<URL>`).imageCaption(`<CAPTION>`).sendImage(`<CALLBACK>`);
<URL>
is the url of the image being posted
<CAPTION>
is the text you want on top of the image
<CALLBACK>
is optional callback otherwise promise is returned
pageClient.postUrl(`<URL>`).postMessage(`<MESSAGE>`).sendPost(`<CALLBACK>`);
<URL>
is the url of the link being posted
<MESSAGE>
is the text you want on top of the link
<CALLBACK>
is optional callback otherwise promise is returned
const facebook = require('fb-messenger-sdk');
const router = require('express').Router();
router.get('/api/webhook',(req, res) => facebook.ValidateWebhook.validateServer(req,res));
Example based on usage with Express Router, can use any other middleware which passes in the req and response objects.
Assumes verification token set under process.env.FB_VERIFICATION_TOKEN
.
Alternatively, if you want to pass in your set token in a different manner or under different name you can use
ValidateWebhook.validateServer(req, res, <TOKEN>);
This allows you to obtain the value as you wish and still use it as above with the help of currying.
...
const validateWebhook = function validateWebhook(token) {
return (req, res) => facebook.ValidateWebhook.validateServer(req, res, token);
}
const validator = validateWebhook(<TOKEN>);
router.get('/api/webhook/',validator);
Alternatively, you can use this when running on AWS Lambda to take advantage of the serverless paradigm as follows:
import {ValidateWebhook} from 'fb-messenger-sdk';
const handler = (event, context, callback: Function) => {
...
if(event.httpMethod === 'GET') {
ValidateWebhook.validateLambda(event, callback);
}
...
}
Both validateLambda
and validateServer
support passing in verification token as third parameter. Otherwise will check process.env.FB_VERIFICATION_TOKEN
for value.
Validates the integrity of the message received from Facebook platform using the provided signature signed with Facebook Application Secret.
The Facebook application secret can be provided either as second optional parameter to ValidateWebhook.validateMessageIntegrity(<X-HUB-SIGNATURE>, <FB_APPLICATION_SECRET>)
or by setting process.env.FB_APPLICATION_SECRET
.
Compatible with both server/less paradigms as part of single line middleware function to Express or as Lambda first check before callback or remainder or programme.
import {ValidateWebhook} from 'fb-messenger-sdk';
const messageIntegrityChecker = (req, res) => {
const validMessage = ValidateWebhook.validateMessageIntegrity(req.headers["x-hub-signature"]);
...
}
router.post('/api/webhook/',messageIntegrityChecker);
const router = require('express').Router();
const facebook = require('fb-messenger-sdk');
const messagingClient = new facebook.FacebookMessagingAPIClient(process.env.PAGE_ACCESS_TOKEN);
const messageParser = facebook.FacebookMessageParser;
...
router.get('/api/webhook',facebook.ValidateWebhook.validateServer);
router.post('/api/webhook', (req, res) => {
const incomingMessages = messageParser.parsePayload(req.body);
...
messagingClient.markSeen(senderId)
.then(() => client.toggleTyping(senderId,true))
.catch((err) => console.log(error));
...
//promise based reaction on message send confirmation
messagingClient.sendTextMessage(senderId, 'Hello')
.then((result) => console.log(`Result sent with: ${result}`));
...
//callback based reaction on message confirmation
messagingClient.sendTextMessage(senderId, 'Hello',(result) => console.log(`Result sent with: ${result}`));
...
//silent message sending
messagingClient.sendTextMessage(senderId,'Hello');
})
or
import {FacebookMessagingAPIClient, ValidateWebhook, FacebookMessageParser} from 'fb-messenger-sdk';
import {Router} from 'express';
...
router.get('/api/webhook',facebook.ValidateWebhook.validateServer);
router.post('/api/webhook', (req, res) => {
try {
const incomingMessages = messageParser.parsePayload(req.body);
...
await messagingClient.markSeen(senderId);
await messagingClient.toggleTyping(senderId,true));
...
//promise based reaction on message send confirmation
const result = await messagingClient.sendTextMessage(senderId, 'Hello');
console.log(`Result sent with: ${result}`));
...
} catch(e){...}
//callback based reaction on message confirmation
messagingClient.sendTextMessage(senderId, 'Hello',(result) => console.log(`Result sent with: ${result}`));
...
//silent message sending
messagingClient.sendTextMessage(senderId,'Hello');
});
FAQs
NodeJS Facebook Messenger API for bots to send messages and setup events to Facebook.
The npm package fb-messenger-sdk receives a total of 15 weekly downloads. As such, fb-messenger-sdk popularity was classified as not popular.
We found that fb-messenger-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.