FeathersJS Filter UI
an universal configurable filter module that will fire an event
and gives you a FeathersJS query that you should apply.

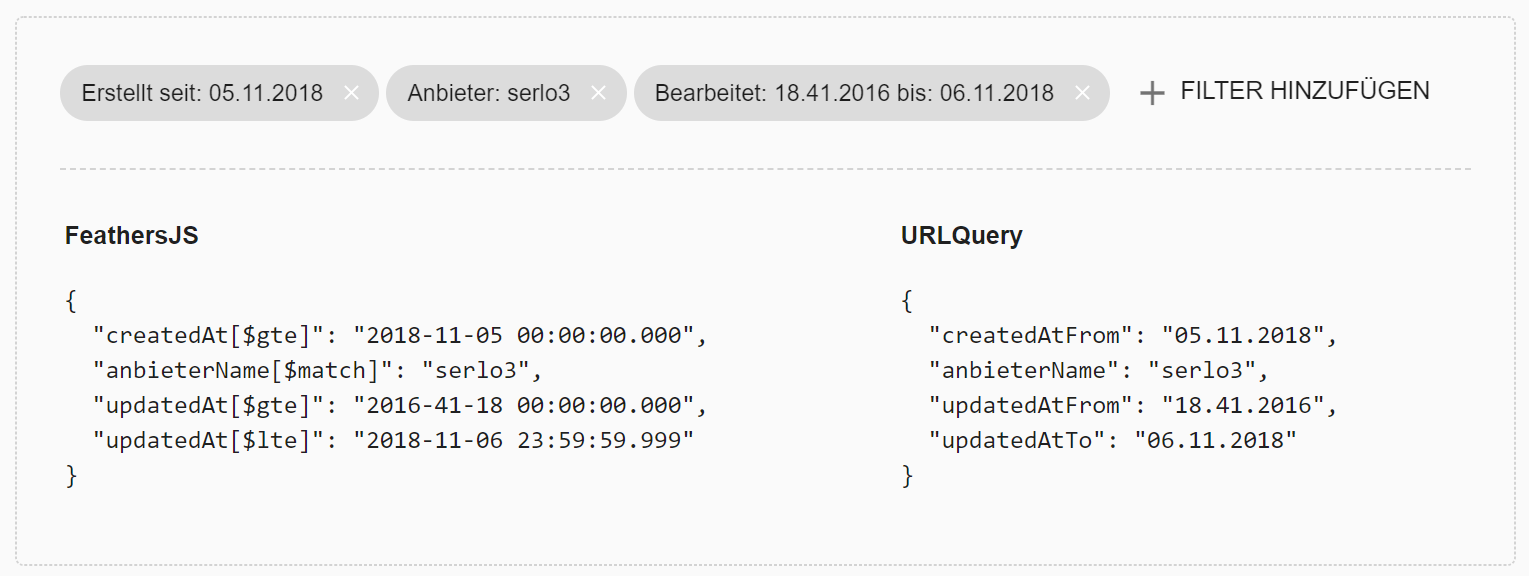
Usage
Vue
Mount globally:
import Vue from "vue";
import FeathersFilter from "feathersjs-filter-ui";
Vue.use(FeathersFilter);
or directly in the component:
import FeathersFilter from "feathersjs-filter-ui";
export default {
components: {
FeathersFilter
},
}
or on any webpage:
<script src="https://unpkg.com/vue"></script>
<script src="./dist/lib/FeathersjsFilterUi.umd.js"></script>
<div id="app">
<demo></demo>
</div>
<script>
new Vue({
components: {
demo: FeathersjsFilterUi
}
}).$mount('#app')
</script>
As a native WebComponent (WIP, currently not working)
simply include the module into your project and you can use it.
Configure it by apply some of the following html-attributes.
Then add an eventListener to watch for new querys.
<html>
<header>
<meta charset="utf-8">
<script src="https://unpkg.com/vue" defer></script>
<script src="./dist/wc/feathersjs-filter-ui.min.js" defer></script>
</header>
<body>
<feathersjs-filter-ui id="filter" add-label="more filter"/>
<script>
document.getElementById("filter").addEventListener('newFilter', (e) => {
console.log("filter:",e.detail[0]);
})
</script>
</body>
</html>
Configuration
add-label add-label="..."
the label of the "add more filter" button.
{type: String, default: "Add Filter"}
apply-label apply-label="..."
the label of the apply button of each filter dialog.
{type: String, default: "apply"}
cancle-label cancle-label="..."
the label of the cancle button of each filter dialog.
{type: String, default: "cancle"}
handleUrl handle-url="..."
should the component update the url, of the window it is mounted,
for you or do you wan't to handle it yourself?
{type: Boolean, default: false}
filter filter="[...]"
you can use the "filter" property to configure the available filter.
The property should be a stringified JSON Object.
{type: Array, default: []}
You can use as many of each type as you want, but at the moment you only have the following filter types.
Options marked with WIP
are Work in Progress
and are currently not working.
date
filter for an date range
{
type: "date",
title: 'Created at'
displayTemplate: 'created from %1 to %2',
property: 'createdAt',
mode: 'from',
autoOrder: false,
hideOnSelect: false,
minDate: (UNIX TIMESTAMP),
maxDate: (UNIX TIMESTAMP),
fromLabel: "STRING",
toLabel: "STRING",
defaultFromDate: (UNIX TIMESTAMP),
defaultToDate: (UNIX TIMESTAMP)
}
if you set minDate or maxDate to false
the related input is hidden.
select
let the user choose an value for a variable
{
type: "select",
title: 'Class'
displayTemplate: 'class: %1',
property: 'classId',
multiple: true,
expanded: true
options: [
[123, "Class A"],
[456, "Class B"],
[789, "Class C"],
],
defaultSelection: [123, 456]
}
sort
let the user order the result
{
type: "sort",
title: 'Sort'
displayTemplate: 'Sort by: %1',
options: [
['propertyA', "Sort by A"],
['propertyB', "Sort by B"],
['propertyC', "Sort by C"],
],
defaultSelection: 'propertyA'
defaultOrder: 'DESC'
}
boolean
toggle if an boolean value should be true or false
{
type: "boolean",
title: 'more'
options: {
'propertyA': "Label A",
'propertyB': "Label B",
'propertyC': "Label C"
},
defaultSelection: {
'propertyA': false,
'propertyC': true
},
applyNegated: {
'propertyA': [true, true],
'propertyB': [false, true],
'propertyC': [true, false],
}
}
applyNegated tells the filter how to query for false/true selections and negates the query according to your settings.
e.g. if the user selects true, and you set the property to [false, true]
the query is looking for not false
resulting in property[$ne]=false
instead of property=true
.
limit
limit the result to the selected amount of items
{
type: "limit",
title: 'Anzahl der Einträge'
displayTemplate: '%1',
options: [
10, 25, 50, 100
],
defaultSelection: 25
}
Theming
Theming can be achived using the following css custom properties:
:root{
--md-theme-default-primary: #009688,
--md-theme-default-primary-on-background: #009688,
}
Development Setup
> git clone https://github.com/adrianjost/feathersjs-filter-ui.git
> cd feathersjs-filter-ui
> yarn install
Build & Development
> yarn dev
> yarn build