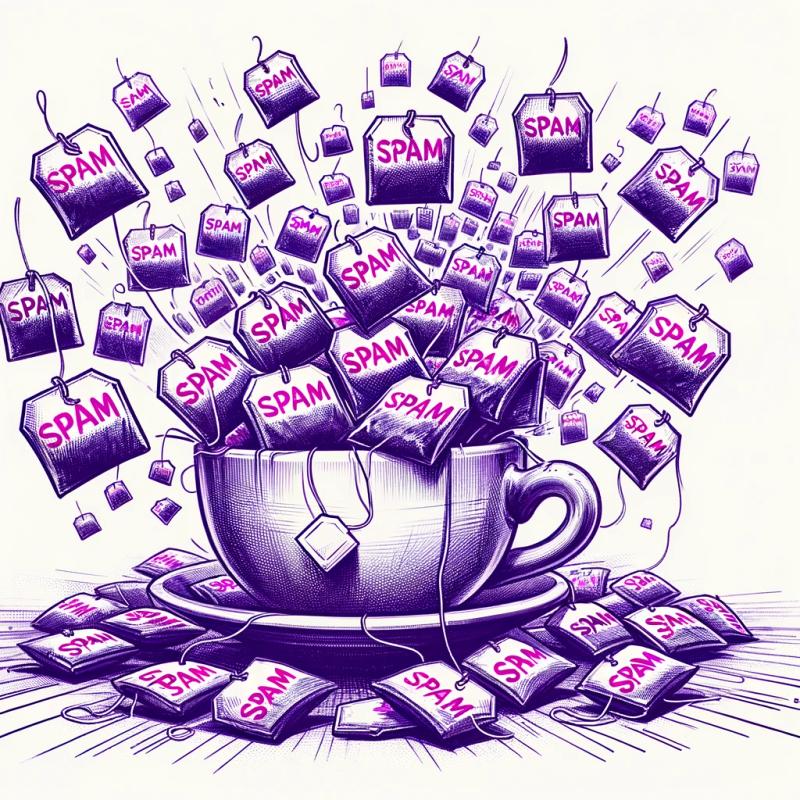
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
fiql-query-builder
Advanced tools
Readme
Feed Item Query Language (FIQL) is a simple, URI-friendly query language for filtering entries of web feeds.
This module provides the utility to generate valid FIQL query strings by using a JSON objects or the custom classes provided.
$ npm install fiql-query-builder
FIQL query strings can be produced by supplying a JSON object, or using the Node classes provided.
// var json = ...;
// Using require()
var fiqlQueryBuilder = require('fiql-query-builder');
fiqlQueryBuilder.convertFromJson(json);
// Using ES6 import
import { convertFromJson } from 'fiql-query-builder';
convertFromJson(json);
Object Key | Children | Description |
---|---|---|
custom_operator |
| Define a custom basic operator |
equals |
| Produces an equality operator (== ) |
not_equals |
| Produces an inequality operator (!= ) |
less_than |
| Produces an less than operator (=lt= ) |
less_than_or_equal |
| Produces an less than or equal operator (=le= ) |
greater_than |
| Produces an greater operator (=gt= ) |
greater_than_or_equal |
| Produces an greater than or equal operator (=ge= ) |
in |
| Produces an in operator (in ) |
out |
| Produces an out operator (out ) |
Object Key | Children | Description |
---|---|---|
custom_expression |
| Define a custom boolean expression |
and |
| Combines child array with an and operator (; ) |
or |
| Combines child array with an or operator (, ) |
Object Key | Children | Description |
---|---|---|
group |
| Wraps an expression in parentheses |
// var node = ...
// Using require()
var fiqlQueryBuilder = require('fiql-query-builder');
fiqlQueryBuilder.convertFromNode(node);
// Using ES6 import
import { convertFromNode, EqNode, AndNode, OpNode } from 'fiql-query-builder';
convertFromNode(node);
LeafNode(value)
The query param is built by traversing the object tree recursively, so a LeafNode
is used to represent a primitive value.
Param | Type | Description |
---|---|---|
value | string | The string value |
OpNode(selector, operator, args)
A generic operator
Param | Type | Description |
---|---|---|
selector | LeafNode | The left-hand side of the operator |
operator | string | The custom operator |
args | GroupNode | LeafNode | ExpNode | OpNode | The child node for operator (right-hand side) |
Standard operator classes have been provided, and can be instantiated using ClassName(selector, args)
.
==
) : EqNode
!=
) : NeqNode
=lt=
) : LtNode
=le=
) : LeNode
=gt=
) : GtNode
=ge=
) : GeNode
=in=
) : InNode
=out=
) : NotInNode
ExpNode(operator, children)
A generic boolean expression
Param | Type | Description |
---|---|---|
operator | string | The custom operator |
children | Node[] | The child nodes for the expression |
Standard boolean expression classes have been provided, and can be instantiated using ClassName(children)
.
;
) : AndNode
,
) : OrNode
GroupNode(exp)
Used to wrap parentheses around a boolean expression.
Param | Type | Description |
---|---|---|
exp | ExpNode | The boolean expression to wrap parentheses around |
var eqJson = convertFromJson({
equals : {
selector: 'foo',
args: 'bar'
}
});
// eqJson = foo==bar
var customOperatorJson = convertFromJson({
custom_operator : {
operator: '¬',
selector: 'foo',
args: 'bar'
}
});
// customOperatorJson equals: foo¬bar
var andJson = convertFromJson({
and : [
{
equals: {
selector: 'foo',
args: 'bar'
}
},
{
not_equals: {
selector: 'baz',
args: 'qux'
}
}
]
});
// andJson equals: foo==bar;baz!=qux
var customExpressionJson = convertFromJson({
custom_expression : {
operator: '*',
children: [
{
equals: {
selector: 'foo',
args: 'bar'
}
},
{
not_equals: {
selector: 'baz',
args: 'qux'
}
}
]
}
});
// customExpressionJson equals: foo==bar*baz!=qux
var groupJson = convertFromJson({
equals : {
selector: 'k',
args: {
group : {
and : [
{
less_than: {
selector: 'foo',
args: 'bar'
}
},
{
not_equals: {
selector: 'baz',
args: 'qux'
}
}
]
}
}
}
});
// groupJson equals: k==(foo=lt=bar,baz!=qux)
var eqNode = convertFromNode(
new EqNode(
new LeafNode('foo'),
new LeafNode('bar')
)
);
// eqNode = foo==bar
var customOperatorNode = convertFromNode(
new OpNode(
new LeafNode('foo'),
'¬',
new LeafNode('bar')
)
);
// customOperatorNode equals: foo¬bar
var andNode = convertFromNode(
new AndNode([
new EqNode(
new LeafNode('foo'),
new LeafNode('bar')
),
new NeqNode(
new LeafNode('baz'),
new LeafNode('qux')
)
])
);
// andNode equals: foo==bar;baz!=qux
var customExpressionNode = convertFromNode(
new ExpNode('*', [
new EqNode('foo', 'bar'),
new NeqNode('baz', 'qux')
])
);
// customExpressionNode equals: foo==bar*baz!=qux
var groupNode = convertFromNode(
new EqNode(
new LeafNode('k'),
new GroupNode(
new AndNode([
new LtNode(
new LeafNode('foo'),
new LeafNode('bar')
),
new NeqNode(
new LeafNode('baz'),
new LeafNode('qux')
)
])
)
)
);
// groupNode equals: k==(foo=lt=bar,baz!=qux)
This project is licensed under MIT License
FAQs
This module provides the utility to generate valid FIQL query strings by using a JSON objects or the custom classes provided.
The npm package fiql-query-builder receives a total of 858 weekly downloads. As such, fiql-query-builder popularity was classified as not popular.
We found that fiql-query-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.