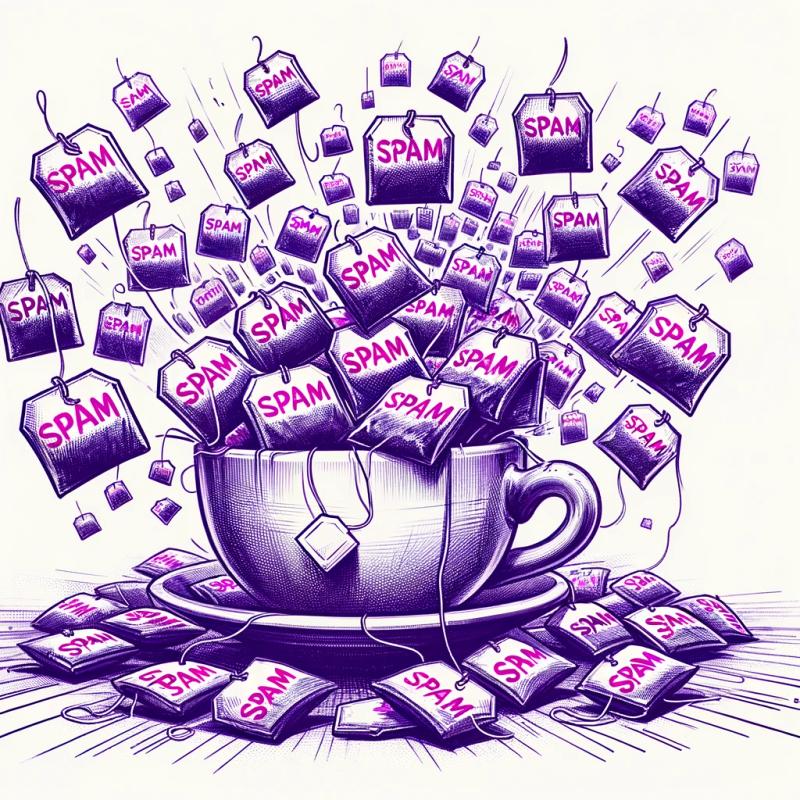
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
fireface
Advanced tools
Readme
A convenient interface for Firebase developers.
Firebase is an excellent tool to have in the developer's kit, and while its standard interface is robust, it can be helpful to have some simple abstractions for common patterns, such as fetching data one time, setting and updating records, and performing queries against your database.
Fireface is designed to work with your Node.js server, and is configured using a service account, meaning your API doesn't need any special rules to access your Firebase database. Note that this also means Fireface and read, write, and delete any data, so you'll need to manage your own access control logic on your server.
Warning: don't use this library in a client-side web application. It's too exploitable.
Install as a local dependency:
npm install -S fireface
Fireface requires you to set up a Firebase project and a service account. When setting up your service account, make sure to download the credentials as a JSON file.
Once your project and account are set up, put your credentials JSON into your project, for example config/firebase/credentials.json
. It's recommended that you add this file to your .gitignore, since this file contains sensitive account information. If you need per-environment configuration, Marshall can help.
const ff = require('fireface');
// Initialize Fireface/Firebase
ff.initializeApp({
serviceAccount: path.join(__dirname, '../config/firebase/credentials.json'),
databaseURL: 'https://my-firebase-database.firebaseio.com',
});
// Get a ref
ff.get('users/1234')
.then(user => {
console.log(user);
});
// Create a ref
// WARNING: this will overwrite the entire ref.
ff.post('users/1234', {
name: 'bob',
admin: true,
}).then(() => {
console.log('user 1234 created');
}).catch(err => {
console.log('failed to create user 1234', err);
});
// Update a ref
ff.put('users/1234', {
name: 'bobby',
}).then(() => {
console.log('user 1234 updated');
}).catch(err => {
console.log('failed to update user 1234', err);
});
// Delete a ref
ff.delete('users/1234').then(() => {
console.log('user 1234 deleted');
});
// Get a new unique ID for a ref
const newUserId = ff.getKey('users');
// Create a user with the new ID
ff.post(`users/${newUserId}`, {
id: newUserId,
name: 'mary',
admin: false,
}).then(() => {
console.log('created new user');
});
// Perform a query on a ref
ff.find('users', { name: 'mary' })
.then(result => {
console.log('found', result);
});
// Update multiple refs at one time
ff.update({
'users/1234': { admin: false },
`users/${newUserId}`: { admin: true },
}).then(() => {
console.log('updated multiple users');
});
To keep better organization of releases this project follows the Semantic Versioning 2.0.0 guidelines.
Want to contribute? Follow these recommendations.
FAQs
A convenient interface for Firebase
The npm package fireface receives a total of 8 weekly downloads. As such, fireface popularity was classified as not popular.
We found that fireface demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.