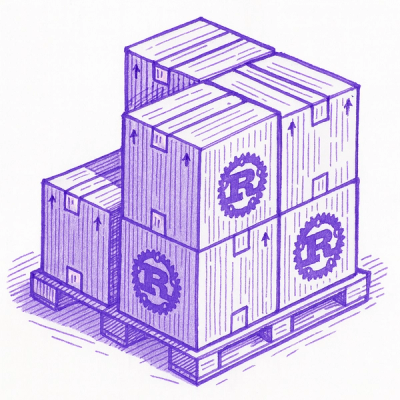
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
fluid-js is a library that generates audio data (frames). fluid-js uses wasm version of FluidSynth.
npm install --save fluid-js
Copies dist/fluid.js
(or dist/fluid.min.js
) and externals/libfluidsynth-2.0.1.js
(libfluidsynth JS file) to your project, and writes <script>
tags as following order:
<script src="libfluidsynth-2.0.1.js"></script>
<script src="fluid.js"></script>
When scripts are available, you can use APIs via Fluid
namespace object.
// Prepare the AudioContext instance
var context = new AudioContext();
var synth = new Fluid.Synthesizer();
synth.init(context.sampleRate);
// Create AudioNode (ScriptProcessorNode) to output audio data
var node = synth.createAudioNode(context, 8192); // 8192 is the frame count of buffer
node.connect(context.destination);
// Load your SoundFont data (sfontBuffer: ArrayBuffer)
synth.loadSFont(sfontBuffer).then(function () {
// Load your SMF file data (smfBuffer: ArrayBuffer)
return synth.addSMFDataToPlayer(smfBuffer);
}).then(function () {
// Play the loaded SMF data
return synth.playPlayer();
}).then(function () {
// Wait for finishing playing
return synth.waitForPlayerStopped();
}).then(function () {
// Wait for all voices stopped
return synth.waitForVoicesStopped();
}).then(function () {
// Releases the synthesizer
synth.close();
}, function (err) {
console.log('Failed:', err);
// Releases the synthesizer
synth.close();
});
(Above example uses Web Audio API, but you can use Synthesizer
without Web Audio, by using render()
method.)
If you prefer to load fluid-js as an ES module, you can use import
statement such as import * as Fluid from 'fluid-js'
.
Notes:
fluid.js
intends the ES2015-supported environment. If you need to run the script without errors on non-ES2015 environment such as IE11 (to notify 'unsupported'), you should load those scripts dynamically, or use transpiler such as babel.import
-able and its license (LGPL v2.1) is differ from fluid-js's (BSD-3-Clause).fluid-js supports AudioWorklet process via dist/fluid.worklet.js
(or dist/fluid.worklet.min.js
). You can load fluid-js on the AudioWorklet as the following code:
var context = new AudioContext();
context.audioWorklet.addModule('libfluidsynth-2.0.1.js')
.then(function () {
return context.audioWorklet.addModule('fluid.worklet.js');
})
.then(function () {
// Create the synthesizer instance for AudioWorkletNode
var synth = new Fluid.AudioWorkletNodeSynthesizer();
synth.init(context.sampleRate);
// You must create AudioWorkletNode before using other methods
// (This is because the message port is not available until the
// AudioWorkletNode is created)
audioNode = synth.createAudioNode(context);
audioNode.connect(context.destination); // or another node...
// After node creation, you can use Synthesizer methods
return synth.loadSFont(sfontBuffer).then(function () {
return synth.addSMFDataToPlayer(smfBuffer);
}).then(function () {
return synth.playPlayer();
}).then(function () {
...
});
});
These classes implement the interface named Fluid.ISynthesizer
.
Fluid.Synthesizer
(construct: new Fluid.Synthesizer()
)
Fluid.AudioWorkletNodeSynthesizer
(construct: new Fluid.AudioWorkletNodeSynthesizer()
)
Fluid.ISynthesizer
methods(Not documented yet. Please see dist/lib/ISynthesizer.d.ts
.)
fluid-js is licensed under BSD 3-Clause License except for the files in externals
directory.
For licenses of the files in externals
directory, please read externals/README.md
.
FAQs
Wrapper JS library of fluidsynth for using with Web Audio or etc.
The npm package fluid-js receives a total of 6 weekly downloads. As such, fluid-js popularity was classified as not popular.
We found that fluid-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.