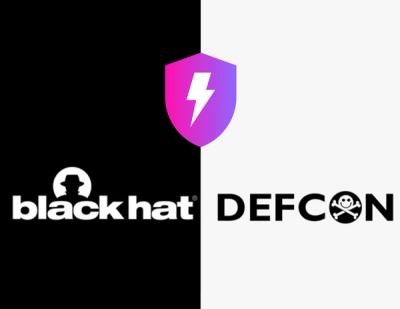
Security News
Meet Socket at BlackHat and DEF CON in Las Vegas
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
font-picker-react
Advanced tools
Readme
A simple, customizable font selector allowing users to preview, choose, and use Google Fonts on your website.
→ Demo
This is the React component for the Font Picker package.
Add the package as a dependency using NPM:
npm install font-picker-react
Then add the <FontPicker />
component somewhere in your React project:
import FontPicker from 'font-picker-react';
<FontPicker
apiKey="YOUR_API_KEY" // Google API key
defaultFont={'Open Sans'}
options={{ limit: 50 }}
/>
Add className="apply-font"
to all JSX elements you want to apply the selected font to.
When the user selects a font using the font picker, it will automatically be downloaded (added as a <link>
to the document's head) and applied to all HTML elements of the "apply-font"
class.
The following props can be passed to the FontPicker
component:
apiKey
(required): Google API key (can be generated here)defaultFont
: Font that is selected on initialization (default: 'Open Sans'
)options
: Object with additional (optional) parameters:
families
: If only specific fonts shall appear in the list, specify their names in an array (default: all font families)categories
: Array of font categories – possible values: 'sans-serif', 'serif', 'display', handwriting', 'monospace'
(default: all categories)variants
: Array of variants which the fonts must include and which will be downloaded; the first variant in the array will become the default variant (and will be used in the font picker and the .apply-font
class); e.g. ['regular', 'italic', '700', '700italic']
(default: ['regular']
)limit
: Maximum number of fonts to be displayed in the list (the least popular fonts will be omitted; default: 100
)sort
: Sorting attribute for the font list – possible values: 'alphabetical'
(default), 'popularity'
onChange
: Function which is executed whenever the user changes the active font and its stylesheet finishes downloadingFAQs
Font selector component for Google Fonts
The npm package font-picker-react receives a total of 4,041 weekly downloads. As such, font-picker-react popularity was classified as popular.
We found that font-picker-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Come meet the Socket team at BlackHat and DEF CON! We're sponsoring some fun networking events and we would love to see you there.
Security News
Learn how Socket's 'Non-Existent Author' alert helps safeguard your dependencies by identifying npm packages published by deleted accounts. This is one of the fastest ways to determine if a package may be abandoned.
Security News
In July, the Python Software Foundation mounted a quick response to address a leaked GitHub token, elected new board members, and added more members to the team supporting PSF and PyPI infrastructure.