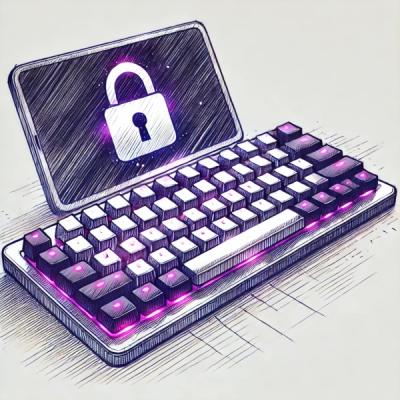
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
formdata-node
Advanced tools
Package description
The formdata-node package is a Node.js module that allows you to create, manipulate, and encode multipart/form-data streams. It can be used to submit forms and upload files via HTTP requests in a way that is compatible with browser FormData API.
Creating FormData
This feature allows you to create a new FormData instance and append fields to it, similar to how you would in a browser environment.
const { FormData } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
Appending Files
This feature enables appending files to the FormData instance, which can then be used to upload files to a server.
const { FormData, fileFromPath } = require('formdata-node');
const formData = new FormData();
formData.append('file', fileFromPath('./path/to/file.txt'));
Retrieving FormData Content
This feature allows you to iterate over the entries in the FormData object, enabling you to access the keys and values that have been appended.
const { FormData } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
for (const [key, value] of formData) {
console.log(key, value);
}
Encoding FormData
This feature is used to encode the FormData into a Blob, which can then be used to send the data over an HTTP request.
const { FormData, formDataToBlob } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
const blob = formDataToBlob(formData);
The 'form-data' package is similar to 'formdata-node' and is used to create readable 'multipart/form-data' streams. It can be used in the Node.js environment and is compatible with 'request' library. It differs in API and internal implementation but serves a similar purpose.
Busboy is a Node.js module for parsing incoming HTML form data. It's different from 'formdata-node' as it focuses on parsing form data rather than creating it, but it can handle multipart/form-data, which is commonly used for file uploads.
Multiparty is a Node.js module for parsing multipart/form-data requests, which is used for uploading files. Unlike 'formdata-node', which is for creating and encoding form data, 'multiparty' is designed for the server-side to parse incoming data.
Readme
FormData implementation for Node.js. Built over Readable stream and async generators. Can be used to communicate between servers with multipart/form-data format.
You can install this package from npm:
npm install --save formdata-node
Or with yarn:
yarn add formdata-node
constructor FormData([fields])
Initialize new FormData instance
get boundary() -> {string}
Returns a boundary string of the current FormData instance.
get stream() -> {stream.Readable}
Returns an internal Readable stream.
set(name, value[, filename]) -> {void}
Set a new value for an existing key inside FormData, or add the new field if it does not already exist.
append(name, value[, filename]) -> {void}
Appends a new value onto an existing key inside a FormData object, or adds the key if it does not already exist.
get(name) -> {string | Buffer | stream.Readable}
Returns the first value associated with the given name. Buffer and Readable values will be returned as-is.
getAll(name) -> {string[] | Buffer[] | stream.Readable[]}
Returns all the values associated with a given key from within a FormData object.
has(name) -> {boolean}
Check if a field with the given name exists inside FormData.
delete(name) -> {void}
Deletes a key and its value(s) from a FormData object.
forEach(callback[, ctx]) -> {void}
Executes a given callback for each field of the FormData instance
keys() -> {iterator}
Returns an iterator allowing to go through the FormData keys
values() -> {iterator}
Returns an iterator allowing to go through the FormData values
entries() -> {iterator}
Returns an iterator allowing to go through the FormData key/value pairs
[Symbol.iterator]() -> {iterator}
An alias of FormData#entries
[Symbol.asyncIterator]() -> {asyncIterator}
Returns an async iterator which allows to read the data from internal Readable stream using for-await syntax. Read the async iteration proposal for more info about async generator functions.
FAQs
Unknown package
We found that formdata-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.