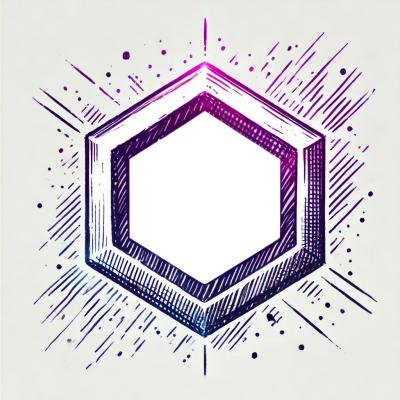
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
This package name is not currently in use, but was formerly occupied by another package. To avoid malicious use, npm is hanging on to the package name, but loosely, and we'll probably give it to you if you want it.
The 'fs' package in Node.js is a core module providing file system related functionality. It allows for interacting with the file system in a way similar to standard POSIX functions.
Reading files
This feature allows you to read the contents of a file asynchronously. The example shows reading a text file and logging its contents.
const fs = require('fs');
fs.readFile('/path/to/file', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Writing files
This feature enables writing data to a file. In the example, a new file is created with the text 'Hello World!'
const fs = require('fs');
fs.writeFile('/path/to/file', 'Hello World!', (err) => {
if (err) throw err;
console.log('File has been saved!');
});
Watching files
This feature allows you to watch for changes in a file or directory. The example logs the type of event and the filename involved.
const fs = require('fs');
fs.watch('/path/to/file', (eventType, filename) => {
console.log(`Event type is: ${eventType}`);
if (filename) {
console.log(`Filename provided: ${filename}`);
} else {
console.log('Filename not provided');
}
});
Enhances the fs module, mainly by making it more robust to various types of errors and by adding queueing to operations. It is a drop-in replacement that handles errors more gracefully and avoids EMFILE errors by queuing.
Builds upon the fs module by adding file system methods that aren't included in the native fs module, such as copying directories and files, and removing directories. It offers more comprehensive file handling capabilities.
A more powerful alternative for watching files and directories in Node.js. It provides a high-level API over node.js fs.watch/fs.watchFile and adds more features and reliability, particularly in scenarios involving complex file watching needs.
This package name is not currently in use, but was formerly occupied by another package. To avoid malicious use, npm is hanging on to the package name, but loosely, and we'll probably give it to you if you want it.
You may adopt this package by contacting support@npmjs.com and requesting the name.
FAQs
This package name is not currently in use, but was formerly occupied by another package. To avoid malicious use, npm is hanging on to the package name, but loosely, and we'll probably give it to you if you want it.
The npm package fs receives a total of 1,224,739 weekly downloads. As such, fs popularity was classified as popular.
We found that fs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.