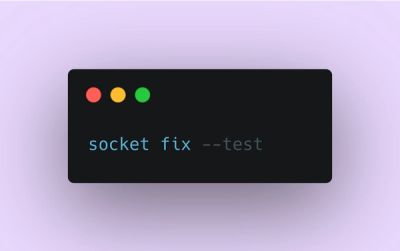
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
a Solana hooks library for React, built on top of gill - the modern JavaScript/TypeScript library for Solana
React hooks library for the Solana blockchain
Welcome to gill-react
, a React hooks library for easily interacting with the Solana blockchain.
Notice:
gill-react
is in active development. All APIs are subject to change until reaching the first major version (v1.0.0).
This React hooks library is built on top of two core libraries:
gill
- modern JavaScript/TypeScript library for interacting with the Solana
blockchain.@tanstack/react-query
- popular and powerful asynchronous
state management for React.Install gill-react
with your package manager of choice:
npm install gill gill-react @tanstack/react-query
pnpm add gill gill-react @tanstack/react-query
yarn add gill gill-react @tanstack/react-query
Note:
gill
and@tanstack/react-query
are peer dependencies ofgill-react
so you need to explicitly install them. This allows you have more/easier control over managing dependencies yourself.
Setup and configure your SolanaProvider
to use the gill hooks:
Manage and use your Solana client's connections:
useSolanaClient
- get the current Solana client (including rpc
and rpcSubscriptions
)useUpdateSolanaClient
- update the current Solana client (including rpc
and
rpcSubscriptions
)Fetch data from the Solana blockchain with the gill hooks:
useAccount
- get the account info for an addressuseBalance
- get account balance (in lamports)useLatestBlockhash
- get the latest blockhashWrap your app with the SolanaProvider
React context provider and pass your Solana client to it:
import { createSolanaClient } from "gill";
import { SolanaProvider } from "gill-react";
const client = createSolanaClient({
urlOrMoniker: "devnet",
});
function App() {
return <SolanaProvider client={client}>{/* ... */}</SolanaProvider>;
}
For application that use React server components, like NextJS, you will need to create a "client only" wrapper for the
SolanaProvider
exported from gill-react
:
"use client"; // <--- this "use client" directive is required!
import { createSolanaClient } from "gill";
import { SolanaProvider } from "gill-react";
const client = createSolanaClient({
urlOrMoniker: "devnet",
});
export function SolanaProviderClient({ children }: { children: React.ReactNode }) {
return <SolanaProvider client={client}>{children}</SolanaProvider>;
}
After creating your client-only provider, you can wrap your app with this
SolanaProviderClient
(normally inside the
root layout.tsx
):
import { SolanaProviderClient } from "@/providers/solana-provider";
export default function RootLayout({ children }: Readonly<{ children: React.ReactNode }>) {
return (
<html>
<body>
<SolanaProviderClient>{children}</SolanaProviderClient>
</body>
</html>
);
}
After you have setup your client-only provider, you must set the use client
directive in any component that uses the
gill-react
library. Signifying this component is required to be "client only".
See React's use client
directive docs.
Note: NextJs uses React server components by default. Read their docs here on the
use client
directive.
"use client"; // <--- directive required anywhere you use `gill-react`
import { useBalance, ... } from "gill-react";
// ... other imports
export function PageClient() {
const { balance } = useBalance("nicktrLHhYzLmoVbuZQzHUTicd2sfP571orwo9jfc8c");
return (
{/* ... */}
);
}
Get the current Solana client configured in the SolanaProvider
,
including the rpc
and rpcSubscriptions
connections:
"use client";
import { useSolanaClient } from "gill-react";
export function PageClient() {
const { rpc, rpcSubscriptions } = useSolanaClient();
// you can now use `rpc` to access any of the Solana JSON RPC methods
return { ... }
}
Get an account's balance (in lamports) using the Solana RPC method of
getBalance
:
"use client";
import { lamportsToSol } from "gill";
import { useBalance } from "gill-react";
export function PageClient() {
const { balance, isLoading, isError, error } = useBalance("nicktrLHhYzLmoVbuZQzHUTicd2sfP571orwo9jfc8c");
// if (isLoading) { return ... }
// if (isError) { return ... }
return (
<div className="">
<p>Balance: {lamportsToSol(balance) + " SOL"}</p>
</div>
);
}
Get the latest blockhash using the Solana RPC method of
getLatestBlockhash
"use client";
import { useLatestBlockhash } from "gill-react";
export function PageClient() {
const { latestBlockhash, isLoading, isError, error } = useLatestBlockhash();
// if (isLoading) { return ... }
// if (isError) { return ... }
return (
<div className="">
<pre>latestBlockhash: {JSON.stringify(latestBlockhash, null, "\t")}</pre>
</div>
);
}
Get the account info for an address using the Solana RPC method of
getAccountInfo
:
"use client";
import { useAccount } from "gill-react";
export function PageClient() {
const { account, isLoading, isError, error } = useAccount("nicktrLHhYzLmoVbuZQzHUTicd2sfP571orwo9jfc8c");
// if (isLoading) { return ... }
// if (isError) { return ... }
return (
<div className="">
<pre>account: {JSON.stringify(account, null, "\t")}</pre>
</div>
);
}
FAQs
a Solana hooks library for React, built on top of gill - the modern JavaScript/TypeScript library for Solana
We found that gill-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.