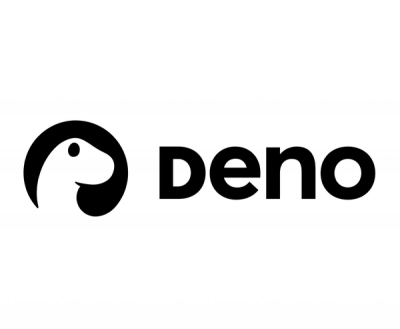
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
github-api
Advanced tools
Github.js provides a minimal higher-level wrapper around Github's API. It was concieved in the context of Prose, a content editor for GitHub.
Github.js is available from npm
or npmcdn.
npm install github-api
<!-- just github-api source (5.3kb) -->
<script src="//npmcdn.com/github-api/dist/GitHub.min.js"></script>
<!-- standalone (20.3kb) -->
<script src="//npmcdn.com/github-api/dist/GitHub.bundle.min.js"></script>
## Compatibility Github.js is tested on Node:
The team behind Github.js has created a whole organization, called GitHub Tools, dedicated to GitHub and its API. In the near future this repository could be moved under the GitHub Tools organization as well. In the meantime, we recommend you to take a look at other projects of the organization.
/*
Data can be retrieved from the API either using callbacks (as in versions < 1.0)
or using a new promise-based API. For now the promise-based API just returns the
raw HTTP request promise; this might change in the next version.
*/
var GitHub = require('github-api');
// unauthenticated client
var gh = new GitHub();
var gist = gh.getGist(); // not a gist yet
gist.create({
public: true,
description: 'My first gist',
files: {
"file1.txt": {
contents: "Aren't gists great!"
}
}
}).then(function(httpResponse) {
// Promises!
var gist = httpResponse.data;
gist.read(function(err, gist, xhr) {
// if no error occurred then err == null
// gist == httpResponse.data
// xhr == httpResponse
});
});
var GitHub = require('github-api');
// basic auth
var gh = new GitHub({
username: 'FOO',
password: 'NotFoo'
});
var me = gh.getUser();
me.getNotification(function(err, notifcations) {
// do some stuff
});
var clayreimann = gh.getUser('clayreimann');
clayreimann.getStarredRepos()
.then(function(httpPromise) {
var repos = httpPromise.data;
});
var GitHub = require('github-api');
// token auth
var gh = new GitHub({
token: 'MY_OAUTH_TOKEN'
});
var yahoo = gh.getOrganization('yahoo');
yahoo.getRepos(function(err, repos) {
// look at all the repos!
})
1.2.1
Repository
: Replace invalid references to postTree
with createTree
FAQs
A higher-level wrapper around the Github API.
The npm package github-api receives a total of 22,754 weekly downloads. As such, github-api popularity was classified as popular.
We found that github-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.