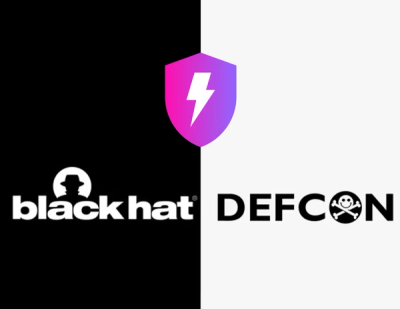
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
github-gist-database
Advanced tools
Unlimited GitHub Gist DB is a lightweight NoSQL database package for Node.js, designed to store JSON data in GitHub Gists, providing CRUD operations and more. Because it depends on the gists and Github says that you can create an unlimted gists and unlimi
Unlimited GitHub Gist DB is a lightweight NoSQL database package for Node.js, designed to store JSON data in GitHub Gists, providing CRUD operations and more. Because it depends on the gists and Github says that you can create an unlimted gists and unlimited requests š¤ for free.
npm install github-gist-database
import { DB } from "github-gist-database";
// Define your Schema
const productSchema = new DB(
{
name: "String",
price: "Number",
},
{
githubToken: process.env.GITHUB_ACCESS_TOKEN!,
schemaName: "productSchema",
projectName: "test",
gistId: "48ec463b54be5973729a108297860555",
timeStamps: true,
}
);
// Example usage
(async () => {
const product = await productSchema.create({
name: "laptop lenovo",
price: 500,
});
console.log(product);
const updatedProduct = await productSchema.findOneAndUpdate(
{ name: "iphone 15 pro max" },
{ name: "laptop Dell", price: 800 }
);
console.log(updatedProduct);
const deletionStatus = await productSchema.findByIdAndDelete(
"33a66454-fc9a-4016-bc01-45731fc16be3"
);
console.log(deletionStatus);
})();
First you have to define the Schema (the body of your database)
import { DB } from "github-gist-database";
// Define your schema
interface Product {
name: string;
price: number;
}
// Initialize the database
const productSchema = new DB<Product>(
{
name: "String",
price: "Number",
},
{
githubToken: process.env.GITHUB_ACCESS_TOKEN!,
schemaName: "productSchema",
projectName: "test",
gistId: "48ec463b54be5973729a108297860555",
timeStamps: true,
}
);
1`
{
name: "String",
price: "Number",
},
this is the fields and its types of your database
you can define many fields as you want.
And here is the types you can create with
| "String"
| "Number"
| "Boolean"
| "Object"
| "Array"
| "Undefined"
| "Null"
| "Symbol"
| "BigInt"
`;
githubToken: process.env.GITHUB_ACCESS_TOKEN!. your github access token you can create it in you Developer Settings
Only Check Gist
And then click Generate Token
This is your github Token
timeStamps: true 3.
timeStamps: true, will add CreatedAt and updatedAT in your schema. whenever you create a new Document Example (new product)
ExamplecreatedAt: '2024-03-04T12:35:52.641Z', updatedAt: '2024-03-04T12:35:52.643Z'
const product = await productSchema.create({
name: "laptop lenovo",
price: 500,
});
console.log(product);
/*
{
name: 'laptop lenovo',
price: 500,
id: '16f1a00f-5527-4783-a966-29355aa5a9de',
createdAt: '2024-03-04T12:35:52.641Z',
updatedAt: '2024-03-04T12:35:52.643Z'
}
*/
console.log(await productSchema.findMany());
/*
[
{
name: 'laptop lenovo',
price: 500,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-04T13:30:25.986Z'
},
{
name: 'laptop lenovo',
price: 500,
id: '479a474b-a668-4407-8543-adcae24d9f91',
createdAt: '2024-03-04T13:31:08.447Z',
updatedAt: '2024-03-04T13:31:08.449Z'
}
]
*/
console.log(await productSchema.findMany());
/*
[
{
name: 'laptop lenovo',
price: 500,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-04T13:30:25.986Z'
},
{
name: 'laptop lenovo',
price: 500,
id: '479a474b-a668-4407-8543-adcae24d9f91',
createdAt: '2024-03-04T13:31:08.447Z',
updatedAt: '2024-03-04T13:31:08.449Z'
}
]
*/
console.log(
await productSchema.findByIdAndUpdate(
"29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7",
{ name: "laptop Dell", price: 800 }
)
);
/*
Before:
{
"name": "laptop lenovo",
"price": 500,
"id": "29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7",
"createdAt": "2024-03-04T13:30:25.984Z",
"updatedAt": "2024-03-04T13:30:25.986Z"
}
After:
{
name: 'laptop Dell',
price: 800,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-05T21:54:15.440Z'
}
*/
console.log(
await productSchema.findByIdAndUpdate(
"29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7",
{ name: "laptop Dell", price: 800 }
)
);
/*
Before:
{
name: 'laptop Dell',
price: 800,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-05T21:54:15.440Z'
}
After:
{
name: 'laptop Dell 2',
price: 800,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-05T21:58:24.302Z'
}
*/
console.log(
await productSchema.findByIdAndUpdate(
"29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7",
{ name: "laptop Dell", price: 800 }
)
);
/*
Before:
{
name: 'laptop Dell 2',
price: 800,
id: '29ad41de-b015-4d96-a9d4-1a5c5a4a4ec7',
createdAt: '2024-03-04T13:30:25.984Z',
updatedAt: '2024-03-05T21:58:24.302Z'
}
After:
Response "Ok"
*/
console.log(
await productSchema.findOneAndDelete({id:"479a474b-a668-4407-8543-adcae24d9f91"})
);
/*
Before:
{
"name": "laptop lenovo",
"price": 500,
"id": "479a474b-a668-4407-8543-adcae24d9f91",
"createdAt": "2024-03-04T13:31:08.447Z",
"updatedAt": "2024-03-04T13:31:08.449Z"
}
After:
Response "Ok"
*/
š¤ ElTahawy
Contributions, issues and feature requests are welcome!
Feel free to check issues page.
Give a āļø if this project helped you!
Copyright Ā© 2024 Amer Eltahawy.
This project is MIT licensed.
FAQs
Unlimited GitHub Gist DB is a lightweight NoSQL database package for Node.js, designed to store JSON data in GitHub Gists, providing CRUD operations and more. Because it depends on the gists and Github says that you can create an unlimted gists and unlimi
The npm package github-gist-database receives a total of 1 weekly downloads. As such, github-gist-database popularity was classified as not popular.
We found that github-gist-database demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.