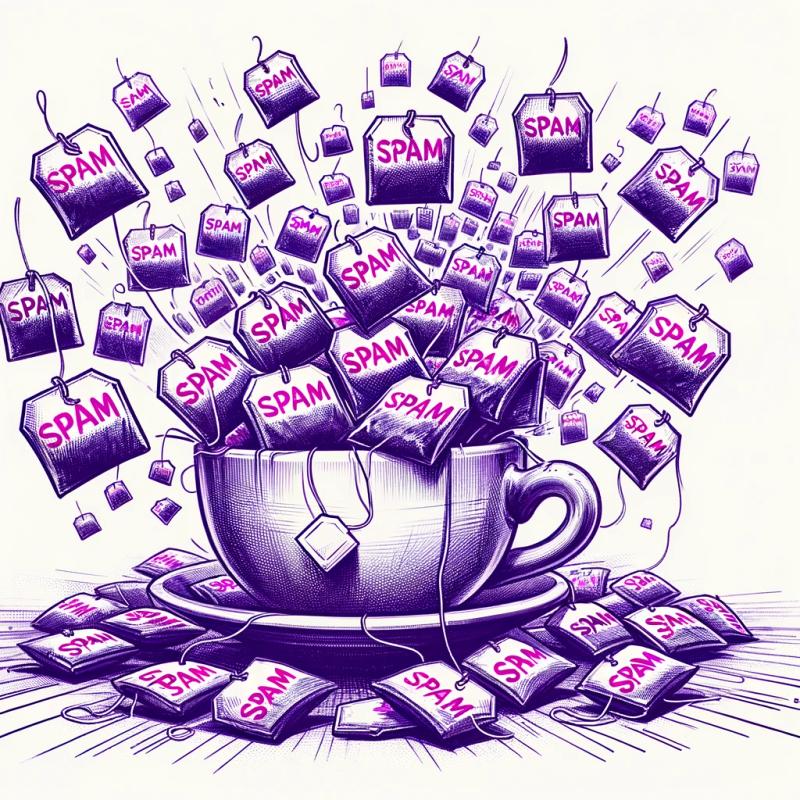
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
gl-vec2
Advanced tools
Readme
Part of a fork of @toji's
gl-matrix split into smaller pieces: this
package contains glMatrix.vec2
.
vec2 = require('gl-vec2')
Will load all of the module's functionality and expose it on a single object. Note that any of the methods may also be required directly from their files.
For example, the following are equivalent:
var scale = require('gl-vec2').scale
var scale = require('gl-vec2/scale')
Adds two vec2's
Math.ceil
the components of a vec2
Creates a new vec2 initialized with values from an existing vector
Copy the values from one vec2 to another
Creates a new, empty vec2
Computes the cross product of two vec2's Note that the cross product must by definition produce a 3D vector
Calculates the euclidian distance between two vec2's. Aliased as dist
.
Divides two vec2's. Aliased as div
.
Calculates the dot product of two vec2's
Returns whether or not the vectors have approximately the same elements in the same position.
Returns whether or not the vectors exactly have the same elements in the same position (when compared with ===)
Math.floor
the components of a vec2
Perform some operation over an array of vec2s.
Creates a new vec2 initialized with the given values
Returns the inverse of the components of a vec2
Calculates the length of a vec2. Aliased as len
.
Performs a linear interpolation between two vec2's
Limit the magnitude of this vector to the value used for the max
parameter
Returns the maximum of two vec2's
Returns the minimum of two vec2's
Multiplies two vec2's. Aliased as mul
.
Negates the components of a vec2
Normalize a vec2
Generates a random vector with the given scale
Math.round
the components of a vec2
Rotates a vec2 by an angle (in radians)
Scales a vec2 by a scalar number
Adds two vec2's after scaling the second operand by a scalar value
Set the components of a vec2 to the given values
Calculates the squared euclidian distance between two vec2's. Aliased as sqrDist
.
Calculates the squared length of a vec2. Aliased as sqrLen
.
Subtracts vector b from vector a. Aliased as sub
.
Transforms the vec2 with a mat2
Transforms the vec2 with a mat2d
Transforms the vec2 with a mat3 3rd vector component is implicitly '1'
Transforms the vec2 with a mat4 3rd vector component is implicitly '0' 4th vector component is implicitly '1'
zlib. See LICENSE.md for details.
FAQs
gl-matrix's vec2, split into smaller pieces
The npm package gl-vec2 receives a total of 110,986 weekly downloads. As such, gl-vec2 popularity was classified as popular.
We found that gl-vec2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.