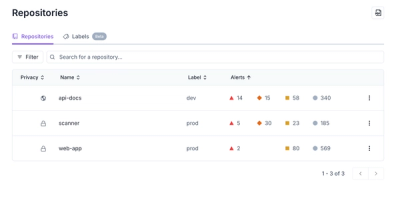
Product
Redesigned Repositories Page: A Faster Way to Prioritize Security Risk
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
google-search-results-nodejs-modified
Advanced tools
This NodeJS module is designed to scrape and parse Google, Bing and Baidu results using SerpApi. This Ruby Gem is meant to scrape and parse Google results using SerpApi. The following services are provided:
SerpApi provides a script builder to get you started quickly.
This Ruby Gem is meant to scrape and parse Google results using SerpApi. The following services are provided:
SerpApi provides a script builder to get you started quickly.
The full documentation is available here.
NPM 7+
$ npm install google-search-results-nodejs
const SerpApi = require('google-search-results-nodejs')
const search = new SerpApi.GoogleSearch("Your Private Key")
search.json({
q: "Coffee",
location: "Austin, TX"
}, (result) => {
console.log(result)
})
This example runs a search about "coffee" using your secret api key.
The SerpApi service (backend)
Alternatively, you can search:
See the playground to generate your code.
The SerpApi api_key can be set globally using a singleton pattern.
const SerpApi = require('google-search-results-nodejs')
let search = new SerpApi.GoogleSearch("Your Private Key")
The SerpApi api_key can be provided for each request
const SerpApi = require('google-search-results-nodejs')
let search = new SerpApi.GoogleSearch()
let result = search.json({
api_key: "Your private key",
q: "Coffee", // search query
location: "Austin, TX", // location
}, (data) => {
console.log(data)
})
query_params = {
q: "query",
google_domain: "Google Domain",
location: "Location Requested",
device: device,
hl: "Google UI Language",
gl: "Google Country",
safe: "Safe Search Flag",
num: "Number of Results",
start: "Pagination Offset",
api_key: "Your SERP API Key", // https://serpapi.com/dashboard
tbm: "nws|isch|shop",
tbs: "custom to be search criteria",
async: true|false, // allow async query
output: "json|html", // output format
}
const SerpApi = require('google-search-results-nodejs')
const search = new SerpApi.GoogleSearch()
// create a callback
callback = (data) => {
console.log(data)
}
// Show result as JSON
search.json(query_params, callback)
// Show result as HTML file
search.html(query_params, callback)
(the full documentation)[https://serpapi.com/search-api]
see below for more hands on examples.
We love true open source, continuous integration and Test Drive Development (TDD). We are using RSpec to test our infrastructure around the clock to achieve the best QoS (Quality Of Service).
The directory test/ includes specification/examples.
Set your api key.
export API_KEY="your secret key"
Run all tests
npm test
const search = new SerpApi.GoogleSearch(api_key)
search.location("Austin", 3, (data) => {
console.log(data)
})
it prints the first 3 location matching Austin (Texas, Texas, Rochester)
[ { id: '585069bdee19ad271e9bc072',
google_id: 200635,
google_parent_id: 21176,
name: 'Austin, TX',
canonical_name: 'Austin,TX,Texas,United States',
country_code: 'US',
target_type: 'DMA Region',
reach: 5560000,
gps: [ -97.7430608, 30.267153 ],
keys: [ 'austin', 'tx', 'texas', 'united', 'states' ] },
...]
The first search result returns a search_id which can be provided to get the search result from the archive.
var search = new SerpApi.GoogleSearch(api_key)
search.json({q: "Coffee", location: "Portland" }, (search_result) => {
// search in archive for the search just returned
search.search_archive(search_result.search_metadata.id, (archived_search) => {
console.log(archived_search)
})
})
it prints the search from the archive.
const search = new SerpApi.GoogleSearch(api_key)
search.account((data) => {
console.log(data)
})
it prints your account information.
This API was developped using basic callback to handle response. And exception are just throw away with interceptor.
if you want to take advantage of the promise to block the request. here is how I will do.
const util = require('util')
function getJson(parameter, resolve, reject) {
const search = new SerpApi.GoogleSearch(api_key)
try {
search.json(parameter, resolve)
} catch (e) {
reject(e)
}
}
const blockFn = util.promisify(getJson)
blockFn[util.promisify.custom](parameter).then((data) => {
expect(data.local_results[0].title.length).toBeGreaterThan(5)
done()
}).catch((error) => {
console.error(error)
done()
})
Reference:
This API is using callback to run in non-blocking code. Here we are trying to follow the spirit of NodeJS. For reference you can read this article:
For pratical example, you can see the test located under test/.
To run the regression suite.
export API_KEY="your api key"
make test
SerpApi supports Google Images, News, Shopping and more.. To enable a type of search, the field tbm (to be matched) must be set to:
The field tbs
allows to customize the search even more.
FAQs
Google Search Node JS API via SerpApi.com
The npm package google-search-results-nodejs-modified receives a total of 2 weekly downloads. As such, google-search-results-nodejs-modified popularity was classified as not popular.
We found that google-search-results-nodejs-modified demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Multiple deserialization flaws in PyTorch Lightning could allow remote code execution when loading untrusted model files, affecting versions up to 2.4.0.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.