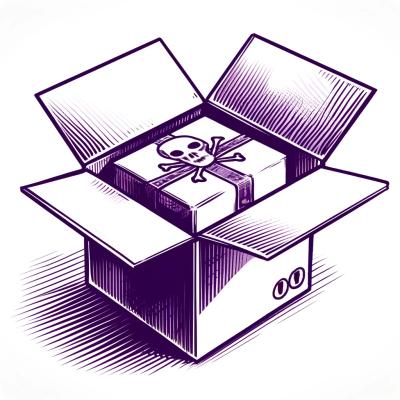
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
graphql-playground-middleware-express
Advanced tools
GraphQL IDE for better development workflows (GraphQL Subscriptions, interactive docs & collaboration).
The graphql-playground-middleware-express package provides middleware for integrating GraphQL Playground, an in-browser IDE for exploring GraphQL APIs, with Express.js applications. It allows developers to easily set up a user-friendly interface for testing and interacting with their GraphQL endpoints.
Setting up GraphQL Playground
This feature allows you to set up GraphQL Playground at a specific endpoint in your Express application. In this example, the playground is accessible at '/playground' and it points to the GraphQL endpoint '/graphql'.
const express = require('express');
const { express: playground } = require('graphql-playground-middleware-express');
const app = express();
app.get('/playground', playground({ endpoint: '/graphql' }));
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Customizing GraphQL Playground
This feature allows you to customize the settings of GraphQL Playground. In this example, the editor theme is set to 'dark' and the cursor shape is set to 'line'.
const express = require('express');
const { express: playground } = require('graphql-playground-middleware-express');
const app = express();
app.get('/playground', playground({
endpoint: '/graphql',
settings: {
'editor.theme': 'dark',
'editor.cursorShape': 'line'
}
}));
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
The graphql-playground package provides a standalone version of GraphQL Playground that can be used with various GraphQL servers. It offers similar functionalities but is not tied to any specific server framework.
GraphiQL is another in-browser IDE for exploring GraphQL APIs. It is highly customizable and can be integrated with various server frameworks. Compared to graphql-playground-middleware-express, GraphiQL offers a different user interface and customization options.
Apollo Server Express is a GraphQL server that can be integrated with Express.js. It includes a built-in GraphQL Playground for testing and interacting with GraphQL APIs. It provides a more comprehensive solution for setting up a GraphQL server with additional features like schema stitching and data sources.
Express middleware to expose an endpoint for the GraphQL Playground IDE SECURITY NOTE: All versions of
graphql-playground-express
until1.7.16
or later have a security vulnerability when unsanitized user input is used while invokingexpressPlayground()
. Read more below
Using yarn:
yarn add graphql-playground-middleware-express
Or npm:
npm install graphql-playground-middleware-express --save
See full example in examples/basic.
const express = require('express')
const expressPlayground = require('graphql-playground-middleware-express')
.default
const app = express()
app.get('/playground', expressPlayground({ endpoint: '/graphql' }))
All versions before 1.7.16
were vulnerable to user-defined input to expressPlayground()
. Read more in the security notes
To fix the issue, you can upgrade to 1.6.12
or later. If you aren't able to upgrade, see the security notes for a workaround.
yarn:
yarn add graphql-playground-express@^1.7.16
npm:
npm install --save graphql-playground-express@^1.7.16
FAQs
GraphQL IDE for better development workflows (GraphQL Subscriptions, interactive docs & collaboration).
The npm package graphql-playground-middleware-express receives a total of 158,016 weekly downloads. As such, graphql-playground-middleware-express popularity was classified as popular.
We found that graphql-playground-middleware-express demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.