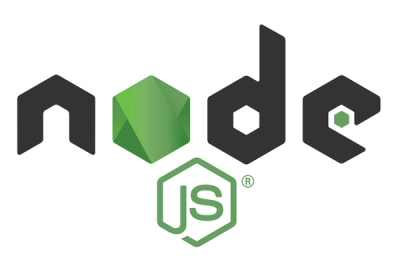
Security News
Node.js Homepage Adds Paid Support Link, Prompting Contributor Pushback
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
graphql-yoga
Advanced tools
graphql-yoga is a fully-featured GraphQL server that is easy to set up and use. It is built on top of GraphQL.js and provides a simple yet powerful API for building GraphQL servers. It comes with out-of-the-box support for features like subscriptions, file uploads, and more.
Basic Server Setup
This code sets up a basic GraphQL server with a single query 'hello' that returns a string. The server is started and listens on port 4000.
const { createServer } = require('graphql-yoga');
const typeDefs = `
type Query {
hello: String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
Subscriptions
This code demonstrates how to set up a GraphQL server with subscriptions. It uses the 'graphql-subscriptions' package to handle real-time updates. A new message is published every second.
const { createServer } = require('graphql-yoga');
const { PubSub } = require('graphql-subscriptions');
const pubsub = new PubSub();
const typeDefs = `
type Query {
hello: String!
}
type Subscription {
newMessage: String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
Subscription: {
newMessage: {
subscribe: () => pubsub.asyncIterator(['NEW_MESSAGE']),
},
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
setInterval(() => {
pubsub.publish('NEW_MESSAGE', { newMessage: 'Hello, world!' });
}, 1000);
File Uploads
This code sets up a GraphQL server that supports file uploads. It defines a custom scalar 'Upload' and a mutation 'singleUpload' that handles the file upload process.
const { createServer } = require('graphql-yoga');
const typeDefs = `
scalar Upload
type Query {
hello: String!
}
type Mutation {
singleUpload(file: Upload!): String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
Mutation: {
singleUpload: async (parent, { file }) => {
const { createReadStream, filename } = await file;
createReadStream().pipe(fs.createWriteStream(path.join(__dirname, filename)));
return filename;
},
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
Apollo Server is a community-driven, open-source GraphQL server that works with any GraphQL schema. It provides a robust set of features, including caching, subscriptions, and more. Compared to graphql-yoga, Apollo Server offers more advanced features and integrations but may require more configuration.
express-graphql is a minimalistic GraphQL HTTP server middleware for Express. It is easy to set up and use, making it a good choice for simple applications. However, it lacks some of the advanced features provided by graphql-yoga, such as built-in subscriptions and file uploads.
graphql-koa is a GraphQL server middleware for Koa. It provides a simple way to integrate GraphQL into a Koa application. While it is similar to express-graphql in terms of simplicity, it does not offer the same level of built-in features as graphql-yoga.
pnpm add graphql-yoga graphql
Make a schema, create Yoga and start a Node server:
import { createServer } from 'node:http'
import { createSchema, createYoga } from 'graphql-yoga'
const yoga = createYoga({
schema: createSchema({
typeDefs: /* GraphQL */ `
type Query {
hello: String
}
`,
resolvers: {
Query: {
hello: () => 'Hello from Yoga!'
}
}
})
})
const server = createServer(yoga)
server.listen(4000, () => {
console.info('Server is running on http://localhost:4000/graphql')
})
envelop
plugins.Our documentation website will help you get started.
We've made sure developers can quickly start with GraphQL Yoga by providing a comprehensive set of
examples.
See all of them in the examples/
folder.
Read more about how GraphQL Yoga compares to other servers in the ecosystem here.
If this is your first time contributing to this project, please do read our Contributor Workflow Guide before you get started off.
For this project in particular, to get started on stage/2-failing-test
:
npm i -g pnpm@8 && pnpm install && pnpm build
packages/graphql-yoga/__tests__
using Jest APIspnpm test
Feel free to open issues and pull requests. We're always welcome support from the community.
Help us keep Yoga open and inclusive. Please read and follow our Code of Conduct as adopted from Contributor Covenant.
MIT
FAQs
Unknown package
The npm package graphql-yoga receives a total of 444,985 weekly downloads. As such, graphql-yoga popularity was classified as popular.
We found that graphql-yoga demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.
Research
Security News
The Socket Research Team investigates a malicious Python typosquat of a popular password library that forces Windows shutdowns when input is incorrect.