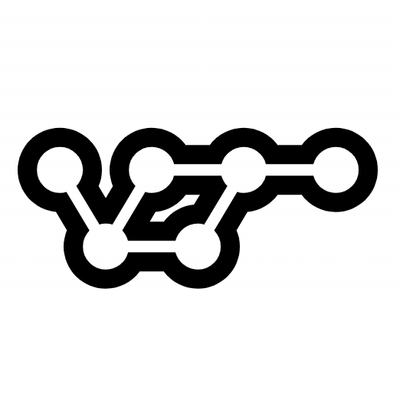
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Simple grid for flexible layouts.
Uses flexbox to produce flexible easy to use classes for rows and columns.
There are 2 versions of Grid. A modern version and one with -prefixes- for better browser support.
bower install wiz-grid
or
npm install grid-css --save
.grid {
display: flex;
}
.cell {
flex: 1;
box-sizing: border-box;
}
<div class="grid">
<div class="cell">first</div>
<div class="cell">second</div>
<div class="cell">third</div>
</div>
This will create a simple flexible row of cells.
-------------------------------------
| first | second | third |
-------------------------------------
You can nest grids inside cells with relative ease...
<div class="grid">
<div class="cell">
<div class="grid">
<div class="cell">first</div>
<div class="cell">second</div>
</div>
<div class="grid">
<div class="cell">third</div>
<div class="cell">fourth</div>
</div>
</div>
<div class="cell">fifth</div>
</div>
Little complicated to explain. This is what it should produce:
-------------------------------------
| first | second | fifth |
--------------------------| |
| third | fourth | |
-------------------------------------
.cell-width-20 {
flex: 0 0 20%;
}
<div class="grid">
<div class="cell cell-width-20">first</div>
<div class="cell">second</div>
<div class="cell">third</div>
</div>
In this example the first cell is 20% the width of the grid, the other two share the remaining space equally.
-------------------------------------
| first | second | third |
-------------------------------------
.cell-offset-20 {
margin-left: 20%;
}
<div class="grid">
<div class="cell cell-width-10">first</div>
<div class="cell cell-width-10 cell-offset-80">second</div>
</div>
Adding cell-offset-80
will add a margin-left: 80%
to the cell, pushing it right.
--------- ----------
| first | | second |
--------- ----------
.grid-wrap {
flex-wrap: wrap;
}
Here we have 2 cells, 50% and 66.6666% wide, but the grid is only 100% wide...
<div class="grid">
<div class="cell cell-width-50">first</div>
<div class="cell cell-width-66">second</div>
</div>
this means the cells are now in control and have broken out of their confinment!
<div class="grid grid-wrap">
<div class="cell cell-width-50">first</div>
<div class="cell cell-width-66">second</div>
</div>
Adding grid-wrap
will push the second cell down under the first cell.
-------------------
| first |
-------------------
-----------------------
| second |
-----------------------
Why not just make this default behaviour? Leaving this option to the developer provides more flexibility.
/* All cells */
.grid-top {
align-items: flex-start;
}
.grid-center {
align-items: center;
}
.grid-bottom {
align-items: flex-end;
}
/* Individual cells */
.cell-top {
align-self: flex-start;
}
.cell-center {
align-self: center;
}
.cell-bottom {
align-self: flex-end;
}
<div class="grid grid-bottom">
<div class="cell cell-top">first</div>
<div class="cell cell-center">second</div>
<div class="cell">third</div> <!-- grid-bottom pushes this to the bottom -->
<div class="cell">fourth
<br>fourth
<br>fourth
<br>fourth
<br>fourth
<br>
</div>
</div>
Notice there are two vertical aligment rules being applied to this grid. A grid wide rule of grid-bottom
that pushes everything to the bottom, and per cell alignment rules of cell-top
and cell-center
that vertically align the cells to the top and center of the grid respectively.
------------ -------------
| first | | fourth |
------------ | |
| fourth |
------------- | |
| second | | fourth |
------------- | |
| fourth |
------------- |
| third | fourth |
-------------------------
@media (min-width: 24em) and (max-width: 48em) {
.grid-medium-fit > .cell:not([class*="cell-width"]) {
flex: 1;
}
.grid-medium-full {
flex-wrap: wrap;
}
.grid-medium-full > .cell {
flex: 0 0 100%;
margin-left: 0;
}
}
Let's make this grid thing respond to different screen sizes...
<div class="grid grid-small-full grid-medium-fit grid-large-full">
<div class="cell cell-top">first</div>
<div class="cell cell-center">second</div>
<div class="cell">third</div> <!-- grid-bottom pushes this to bottom -->
<div class="cell">fourth
<br>fourth
<br>fourth
<br>fourth
<br>fourth
<br>
</div>
</div>
.grid-small-full
this rule makes the cells full width but only when the screen is small.grid-medium-fit
makes the cells share the space, but only for medium screens.grid-large-full
turns it all into columns againThere are some handy "make visible when small" and "hide when large" rules for you to play with.
.visible-small {
display: none;
}
.hidden-medium {
display: none;
}
.hidden-large {
display: none;
}
FAQs
Simple grid for flexible layouts
The npm package grid-css receives a total of 8 weekly downloads. As such, grid-css popularity was classified as not popular.
We found that grid-css demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.