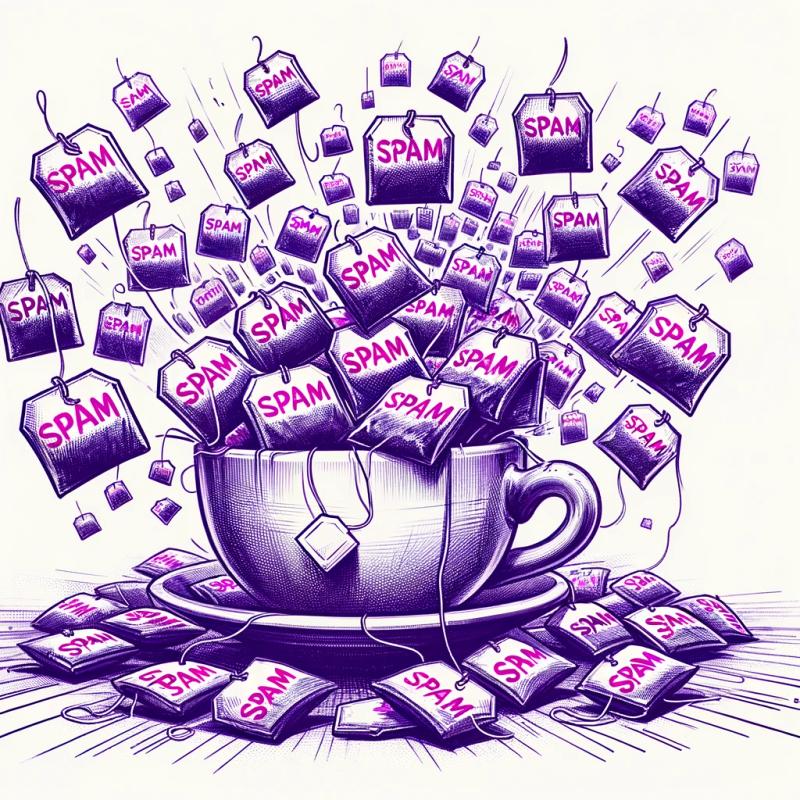
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
handlebars-i18n
Advanced tools
Readme
handlebars-i18n
adds the internationalization features of i18next to handlebars.js. It also provides date, number, and currency formatting via Intl. Use as node module or in the web browser. Supports Typescript.
Handlebars-i18n is listed amongst i18next’s framework helpers.
Copyright (c) 2020–24 Florian Walzel,
MIT License
If you use handlebars-i18n in a professional context, you could
If you use version npm >= 7:
$ npm i handlebars-i18n
For older versions do:
$ npm i handlebars-i18n handlebars@4.7.6 i18next@20.2.1 intl@1.2.5
Usage within node environment:
const HandlebarsI18n = require("handlebars-i18n");
HandlebarsI18n.init();
Usage in web browser:
<script src="handlebars.js"></script>
<script src="i18next.js"></script>
<script src="handlebars-i18n.js"></script>
<script>
HandlebarsI18n.init()
</script>
With ES6 import syntax:
import * as HandlebarsI18n from "handlebars-i18n";
HandlebarsI18n.init();
Initialize i18next with your language strings and default settings:
const i18next = require("i18next");
i18next.init({
resources : {
"en" : {
translation : {
"phrase1": "What is good?",
"phrase2": "{{thing}} is good."
}
},
"de" : {
translation: {
"phrase1": "Was ist gut?",
"phrase2": "{{thing}} ist gut."
}
}
},
lng : "en"
});
Set your Handlebars.js data object:
let data = {
myItem: "handlebars-i18n",
myPrice: 1200.99,
myDate: "2020-03-11T03:24:00"
}
Initialize handlebars-i18n:
HandlebarsI18n.init();
Optionally configure your language specific number, currency, and date-time defaults:
HandlebarsI18n.configure([
["en", "PriceFormat", {currency: "USD"}],
["de", "PriceFormat", {currency: "EUR"}]
]);
Finally use in template:
<p> {{__ "phrase1"}} </p>
<p> {{__ "phrase2" thing=myItem}} </p>
<p> {{_date myDate}} </p>
<p> {{_price myPrice}} </p>
:point_right: See the examples folder in the repo for more use cases and details.
examples/browser-example/index.html
in your Web browser to see an implementation with a simple UI$ npm run example:js
in the console to get a very basic node example logged$ npm run example:ts
to compile and log a typescript exampleHandlebars-i18n has its own command line interface handlebars-i18n-cli.
$ npm i handlebars-i18n-cli --save-dev
Automatically extract translation strings from handlebars templates and generate i18next conform json files from it. Handlebars-i18n-cli also helps to keep your translations up to date when changes are made in the templates over time.
Returns the phrase associated with the given key for the selected language. __ will take all options i18next’s t-function would take. The primary key can be passed hard encoded in the template when written in quotes:
{{__ "keyToTranslationPhrase"}}
… or it can be referenced via a handlebars variable:
{{__ keyFromHandlebarsData}}
Variable Replacement
Template usage:
{{__ "whatIsWhat" a="Everything" b="fine"}}
The i18next resource:
"en" : {
translation : {
"whatIsWhat": "{{a}} is {{b}}."
}
}
Plurals
{{__ "keyWithCount" count=8}}
"en" : {
translation : {
"keyWithCount" : "{{count}} item",
"keyWithCount_plural" : "{{count}} items"
}
}, ...
Override globally selected language
{{__ "key1" lng="de"}}
Will output the contents for "de" even though other language is selected.
Returns the shortcode of i18next’s currently selected language such as "en", "de", "fr", "fi" … etc.
{{_locale}}
Checks a string against i18next’s currently selected language. Returns true or false.
{{#if (localeIs "en")}} ... {{/if}}
Outputs a formatted date according to the language specific conventions.
{{_date}}
If called without argument the current date is returned. Any other input date can be passed as a conventional date string, a number (timestamp in milliseconds), or a date array. _date accepts all arguments Javascript’s new Date() constructor would accept.
Date argument given as date string:
{{_date "2020-03-11T03:24:00"}}
or
{{_date "December 17, 1995 03:24:00"}}
Date argument given as number (milliseconds since begin of unix epoch):
{{_date 1583922952743}}
Date argument given as javascript date array [year, monthIndex [, day [, hour [, minutes [, seconds [, milliseconds]]]]]]:
{{_date "[2012, 11, 20, 3, 0, 0]"}}
Additional arguments for formatting
You can add multiple arguments for individual formatting. See Intl DateTimeFormat for your option arguments.
{{_date 1583922952743 year="2-digit" day="2-digit" timeZone="America/Los_Angeles"}}
Outputs a formatted number according to the language specific conventions of number representation, e.g. 4,100,000.8314 for "en", but 4.100.000,8314 for "de".
{{_num 4100000.8314 }}
Additional arguments for formatting
You can add multiple arguments for individual formatting. See Intl NumberFormat for your option arguments.
{{_num 3.14159 maximumFractionDigits=2}}
Will output 3.14 for "en", but 3,14 for "de".
Outputs a formatted currency string according to the language specific conventions of price representation, e.g. €9,999.99 for "en", but 9.999,99 € for "de".
{{_price 9999.99}}
Additional arguments for formatting
You can add multiple arguments for individual currency formatting. See Intl NumberFormat for your option arguments.
{{_price 1000 currency="JPY" minimumFractionDigits=2}}
Instead of defining the formatting options for each date, number or price anew, you can configure global settings for all languages or only for specific languages.
HandlebarsI18n.configure("all", "DateTimeFormat", {timeZone: "America/Los_Angeles"});
First argument is the language shortcode or "all" for all languages. Second is the format option you want to address (DateTimeFormat, NumberFormat, or PriceFormat). Third argument ist the options object with the specific settings.
You can define specific subsets to be used in the template, i.e. if you want the date in different formats such as:
To do this, define a 4th parameter with a custom name:
HandlebarsI18n.configure([
["en", "DateTimeFormat", {year:"numeric"}, "year-only"],
["en", "DateTimeFormat", {year:"numeric", month:"numeric", day:"numeric"}, "standard-date"],
['en', 'DateTimeFormat', {hour:"numeric", minute:"numeric", second:"numeric", hour12:false}, "time-only"]
]);
Call a subset in template with the parameter format="custom-name", like:
{{_date myDate format="year-only"}}
The general lookup cascade is:
{{_date format="my-custom-format"}}
{{_date timeZone="America/Los_Angeles" year="2-digit"}}
Example:
This defines that all prices for all languages are represented in Dollar:
HandlebarsI18n.configure("all", "PriceFormat", {currency: "USD"});
This defines that all prices for all languages are represented in Dollar, but that for the language French the currency is Euro:
HandlebarsI18n.configure([
["all", "PriceFormat", {currency: "USD"}],
["fr", "PriceFormat", {currency: "EUR"}]
]);
Dismiss all existing configurations:
HandlebarsI18n.reset();
Sometimes you may want to use a Handlebars object you have already modified before, or you may want to use multiple discrete instances of Handlebars. In this case you can pass you custom Handlebars instance to the init function to use it instead of the generic Handlebars object like so:
const HandlebarsModified = require("handlebars");
HandlebarsModified.registerHelper("foo", function() { return "what you want" });
HandlebarsI18n.init(HandlebarsModified);
HandlebarsI18n will have your previously defined method foo() by now.
The same can be done for a custom instance of i18next. Pass it as the second argument to the init function.
const i18nextCustom = require("i18next");
i18nextCustom.createInstance( /* pass some params here ... */ );
HandlebarsI18n.init(null, i18nextCustom);
$ npm test
For your contribution, I would like to thank @MickL, @dargmuesli, and @DiefBell.
There is a different package named handlebars-i18next by @jgonggrijp which might also suit your needs. Cheers!
FAQs
handlebars-i18n adds internationalization to handlebars.js using i18next and Intl
The npm package handlebars-i18n receives a total of 703 weekly downloads. As such, handlebars-i18n popularity was classified as not popular.
We found that handlebars-i18n demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.