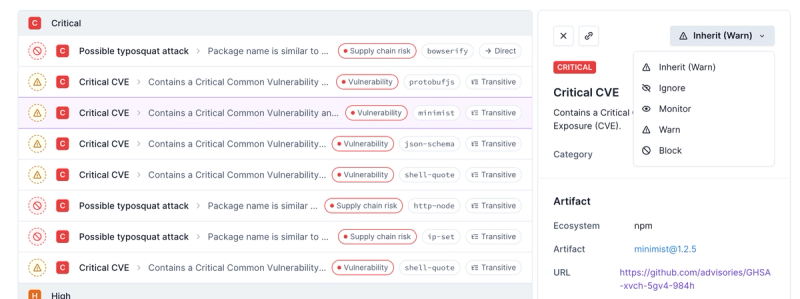
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
handlebars-layouts
Advanced tools
Package description
The handlebars-layouts npm package is an extension for Handlebars that provides layout and partial support, allowing you to create complex templates with reusable components and layouts. It simplifies the process of managing nested templates and layouts in Handlebars.
Define Layouts
This feature allows you to define layouts that can be extended by other templates. In this example, a base layout is extended, and content is inserted into the 'body' section.
const Handlebars = require('handlebars');
const layouts = require('handlebars-layouts');
Handlebars.registerHelper(layouts(Handlebars));
const source = `
{{#extend "base"}}
{{#content "body"}}
<h1>{{title}}</h1>
<p>{{message}}</p>
{{/content}}
{{/extend}}
`;
const template = Handlebars.compile(source);
const context = { title: 'Hello, World!', message: 'This is a message.' };
const result = template(context);
console.log(result);
Nested Layouts
This feature demonstrates how to use nested layouts. A base layout is extended by a child layout, which is further extended by a page template. This allows for complex and reusable template structures.
const Handlebars = require('handlebars');
const layouts = require('handlebars-layouts');
Handlebars.registerHelper(layouts(Handlebars));
const baseLayout = `
<!DOCTYPE html>
<html>
<head>
<title>{{title}}</title>
</head>
<body>
{{#block "body"}}{{/block}}
</body>
</html>
`;
const childLayout = `
{{#extend "base"}}
{{#content "body"}}
<div class="container">
{{#block "content"}}{{/block}}
</div>
{{/content}}
{{/extend}}
`;
const pageTemplate = `
{{#extend "child"}}
{{#content "content"}}
<h1>{{title}}</h1>
<p>{{message}}</p>
{{/content}}
{{/extend}}
`;
Handlebars.registerPartial('base', baseLayout);
Handlebars.registerPartial('child', childLayout);
const template = Handlebars.compile(pageTemplate);
const context = { title: 'Nested Layouts', message: 'This is a nested layout example.' };
const result = template(context);
console.log(result);
Dynamic Partials
This feature allows for dynamic partials, where the partial to be included is determined at runtime. In this example, the partial name is looked up from the context and the corresponding partial is rendered.
const Handlebars = require('handlebars');
const layouts = require('handlebars-layouts');
Handlebars.registerHelper(layouts(Handlebars));
const source = `
{{#extend "base"}}
{{#content "body"}}
{{> (lookup . 'partialName') }}
{{/content}}
{{/extend}}
`;
const baseLayout = `
<!DOCTYPE html>
<html>
<head>
<title>{{title}}</title>
</head>
<body>
{{#block "body"}}{{/block}}
</body>
</html>
`;
const partials = {
partial1: '<p>This is partial 1</p>',
partial2: '<p>This is partial 2</p>'
};
Handlebars.registerPartial('base', baseLayout);
Handlebars.registerPartial('partial1', partials.partial1);
Handlebars.registerPartial('partial2', partials.partial2);
const template = Handlebars.compile(source);
const context = { title: 'Dynamic Partials', partialName: 'partial1' };
const result = template(context);
console.log(result);
Handlebars is a popular templating engine that provides the core functionality for creating templates. While it supports partials, it does not natively support layouts and nested templates like handlebars-layouts. Handlebars-layouts extends Handlebars to provide these additional features.
Express-handlebars is a Handlebars view engine for Express. It supports layouts and partials, making it similar to handlebars-layouts. However, express-handlebars is specifically designed to work with the Express framework, whereas handlebars-layouts can be used more generally with Handlebars.
Hbs is another Express view engine for Handlebars. It provides support for partials and layouts, similar to handlebars-layouts. Like express-handlebars, hbs is tailored for use with the Express framework, while handlebars-layouts is a more general-purpose solution.
Changelog
0.3.0 - 0.3.1
Features:
Readme
Handlebars helpers which implement Jade-like layout blocks.
With Node.js:
$ npm install handlebars-layouts
With Bower:
$ bower install handlebars-layouts
With Component:
$ component install shannonmoeller/handlebars-layouts
<!doctype html>
<html lang="en-us">
<head>
{{#block "head"}}
<title>{{title}}</title>
<link rel="stylesheet" href="assets/css/screen.css" />
{{/block}}
</head>
<body>
<div class="site">
<div class="site-hd" role="banner">
{{#block "header"}}
<h1>{{title}}</h1>
{{/block}}
</div>
<div class="site-bd" role="main">
{{#block "body"}}
<h2>Hello World</h2>
{{/block}}
</div>
<div class="site-ft" role="contentinfo">
{{#block "footer"}}
<small>© 2013</small>
{{/block}}
</div>
</div>
{{#block "foot"}}
<script src="assets/js/controllers/home.js"></script>
{{/block}}
</body>
</html>
{{#extend "layout"}}
{{#append "head"}}
<link rel="stylesheet" href="assets/css/home.css" />
{{/append}}
{{#replace "body"}}
<h2>Welcome Home</h2>
<ul>
{{#items}}
<li>{{.}}</li>
{{/items}}
</ul>
{{/replace}}
{{#prepend "foot"}}
<script src="assets/js/analytics.js"></script>
{{/prepend}}
{{/extend}}
// Load Handlebars
var Handlebars = require('handlebars');
// Register helpers
require('handlebars-layouts')(Handlebars);
// Register partials
Handlebars.registerPartial('layout', fs.readFileSync('layout.html', 'utf8'));
// Compile template
var template = Handlebars.compile(fs.readFileSync('template.html', 'uft8'));
// Render template
var output = template({
title: 'Layout Test',
items: [
'apple',
'orange',
'banana'
]
});
console.log(output);
<!doctype html>
<html lang="en-us">
<head>
<title>Layout Test</title>
<link rel="stylesheet" href="assets/css/screen.css" />
<link rel="stylesheet" href="assets/css/home.css" />
</head>
<body>
<div class="site">
<div class="site-hd" role="banner">
<h1>Layout Test</h1>
</div>
<div class="site-bd" role="main">
<h2>Welcome Home</h2>
<ul>
<li>apple</li>
<li>orange</li>
<li>banana</li>
</ul>
</div>
<div class="site-ft" role="contentinfo">
<small>© 2013</small>
</div>
</div>
<script src="assets/js/analytics.js"></script>
<script src="assets/js/controllers/home.js"></script>
</body>
</html>
$ npm test
MIT
FAQs
Handlebars helpers which implement layout blocks similar to Jade, Jinja, Nunjucks, Swig, and Twig.
The npm package handlebars-layouts receives a total of 90,739 weekly downloads. As such, handlebars-layouts popularity was classified as popular.
We found that handlebars-layouts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.