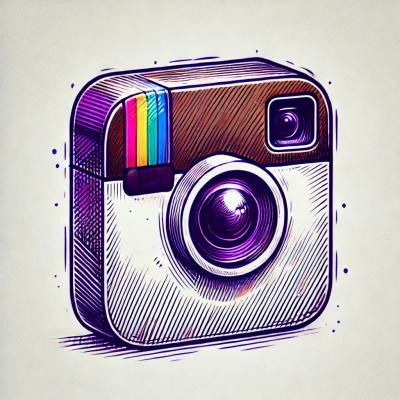
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
hapi-algolia-search
Advanced tools
Hapi plugin wrapping the JS Algolia Search client
Algolia is a "Search Engine as a Service", with high performance, great docs, and API clients available for many languages. Check it out !
$ npm install hapi-algolia-search
const HapiAlgoliaSearch = require('hapi-algolia-search');
const plugin = {
register: HapiAlgoliaSearch,
options: {
appId: 'ABCDEFGHIJ',
apiKey: 'LKHFDLSKHFLKSDFHLSKHFSLKFHLSKHDFLSKHDFLKHSDFL',
indexPrefix: 'dev_', //optional
indexes: {
Users: 'usersIndex',
Products: 'productsIndex'
},
clientOptions: {
timeout: 1000
}
}
};
server.register(plugin, (err) => {
if (err) {
console.log('Failed loading plugin');
}
});
{
"servers": [{
"port": 8080
}],
"plugins": {
"hapi-algolia-search": {
"appId": "ABCDEFGHIJ",
"apiKey": "LKHFDLSKHFLKSDFHLSKHFSLKFHLSKHDFLSKHDFLKHSDFL",
"indexPrefix": "dev_",
"indexes": {
"Users": "usersIndex",
"Products": "productsIndex"
},
"clientOptions": {
"timeout": 1000
}
}
}
}
appId
(required) your Algolia API App IDapiKey
(required) your Algolia API Api keyindexes
(optional) you can specify indexes that will be automatically initialized and exposed by the pluginindexPrefix
(optional) a prefix you want to apply to all index names, i.e to reflect your current environment (https://www.algolia.com/doc/node#different-environments)clientOptions
(optional) see https://github.com/algolia/algoliasearch-client-js#client-optionsIn your route handler :
server.route({
method: "GET",
path: "/search/{term}",
handler: function(request, reply) {
const term = request.params.term;
// search in the "product" index, initialized in the configuration example
const productIndex = request.server.plugins['hapi-algolia-search'].indexes.Products;
productsIndex.search(term, function(err, results){
if(err) { return reply(err); } //please do a better error handling than this
reply(results);
// or reply(results.hits)
})
// or access the client directly for multiple indexes queries or other advanced stuff
// see the official client docs : https://github.com/algolia/algoliasearch-client-js
const algoliaClient = request.server.plugins['hapi-algolia-search'].client
client.search(.....
}
})
Any issues or questions (no matter how basic), open an issue. Please check if it's not an issue with the Algolia API first. Also, please take the initiative to include basic debugging information like operating system and relevant version details such as:
$ npm version
#{ 'hapi-algolia-search': '1.0.0-alpha',
# npm: '3.3.12',
# ares: '1.10.1-DEV',
# http_parser: '2.6.0',
# icu: '56.1',
# modules: '47',
# node: '5.1.0',
# openssl: '1.0.2d',
# uv: '1.7.5',
# v8: '4.6.85.31',
# zlib: '1.2.8' }
Contributions are welcome. Your code should:
If you're changing something non-trivial, you may want to submit an issue first.
MIT
FAQs
Simple Hapi plugin for Algolia Search
The npm package hapi-algolia-search receives a total of 0 weekly downloads. As such, hapi-algolia-search popularity was classified as not popular.
We found that hapi-algolia-search demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.