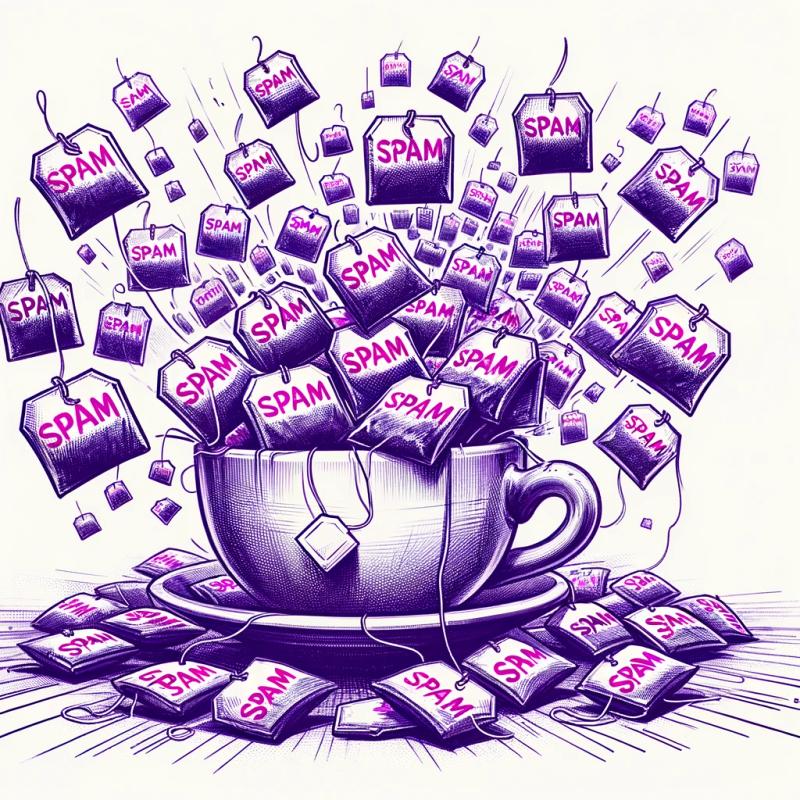
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
hapi-sequelize-dynamic-fields
Advanced tools
Readme
Sometimes we return values at a given endpoint that not always we need all the information, with the plugin you can go through the header which fields you want to return.
For example, we have a model of Tasks (sequelize) that has a relationship for the user. In this way, we could structurer the following query sequelize:
const options = {
attributes: ['id', 'descriptions', 'observation'],
include: [{
model: request.database.User,
attributes: ['id', 'username', 'lastname', 'email']
}]
};
In this case, all the fields entered in the attributes properties will be returned, including to the related table. But, will all this information always be used?
We can do in the following manner:
model.findAndCountAll(request.fieldsAll(options));
The plugin provide a call , request.fieldsAll
, in which checks if there a property in the header called fields
, if there is, the query will be mounted according to the fields informed, in case the field there isn’t, an exception will be thrown AttributesInvalidError
.
In the return of our request we can inform which fields are permitted, by the function request.fieldsHeaders
, for example:
return reply(values).header('allowing-fields', request.fieldsHeaders(options));
Response Headers:
"allowing-fields": "id,descriptions,observation,User.id,User.username,User.firstName,User.lastName,User.email",
##Example:
const Hapi = require('hapi');
const path = require('path');
let server = new Hapi.Server();
const dir = path.join(__dirname, '/models/**.js');
const register = [{
register: require('k7'),
options: {
models: dir,
adapter: require('k7-sequelize'),
connectionOptions: {
options: {
dialect: 'sqlite'
}
}
}
}, {
register: require('hapi-sequelize-dynamic-fields')
}];
server.register(register, (err) => {
if (err) throw err;
});
server.connection();
const User = sequelize.define('User', {
username: {
type: DataType.STRING(40),
allowNull: false,
unique: true
},
firstName: {
type: DataType.STRING(100),
allowNull: false,
field: 'first_name'
},
lastName: {
type: DataType.STRING(50),
allowNull: false,
field: 'last_name'
},
email: {
type: DataType.STRING(120),
allowNull: false,
unique: true,
validate: {
isEmail: true
}
},
password: {
type: DataType.STRING(200),
allowNull: false
}
}, {
createdAt: 'created_at',
updatedAt: 'update_at',
tableName: 'users'
});
const Tasks = sequelize.define('Tasks', {
descriptions: {
type: DataType.STRING(200),
allowNull: false,
unique: true
},
observation: {
type: DataType.STRING(100),
allowNull: false
},
userId: {
type: DataType.INTEGER,
allowNull: false,
references: {
model: 'users',
key: 'id'
},
field: 'user_id'
}
}, {
createdAt: 'created_at',
updatedAt: 'update_at',
tableName: 'tasks',
classMethods: {
associate: (models) => {
Tasks.belongsTo(models.User, {
foreignKey: 'userId'
});
}
}
});
...
server.route([
{
method: 'GET',
path: '/user',
config: {
handler: Controller.list
}
},
{
method: 'GET',
path: '/tasks',
config: {
handler: Controller.listTasks
}
}
]);
...
export const list = async (request, reply) => {
try {
const model = request.database.User;
const options = {
attributes: ['id', 'username', 'lastname', 'email'],
};
const values = await model.findAndCountAll(request.fieldsAll(options));
return reply(values).header('allowing-fields', request.fieldsHeaders(options));
} catch (err) {
return reply.badImplementation(err);
}
};
export const listTasks = async (request, reply) => {
try {
const model = request.database.Tasks;
const options = {
attributes: ['id', 'descriptions', 'observation'],
include: [{
model: request.database.User,
attributes: ['id', 'username', 'lastname', 'email']
}]
};
const values = await model.findAndCountAll(request.fieldsAll(options));
return reply(values).header('allowing-fields', request.fieldsHeaders(options));
} catch (err) {
return reply.badImplementation(err);
}
};
####request
curl -X GET --header 'Accept: application/json' --header 'fields: id' 'http://localhost:3000/user'
####SQL
SELECT 'id' FROM 'users' AS 'User';
curl -X GET --header 'Accept: application/json' --header 'fields: id, email' 'http://localhost:3000/user'
####SQL
SELECT 'id', 'email' FROM 'users' AS 'User';
####Response Headers { "allowing-fields": "username,firstName,lastName,email", ... }
curl -X GET --header 'Accept: application/json' 'http://localhost:3000/tasks'
####SQL
SELECT
'Tasks'.'id',
'Tasks'.'descriptions',
'Tasks'.'observation',
'User'.'id' AS 'User.id',
'User'.'username' AS 'User.username',
'User'.'first_name' AS 'User.firstName',
'User'.'last_name' AS 'User.lastName',
'User'.'email' AS 'User.email'
FROM 'tasks' AS 'Tasks'
LEFT OUTER JOIN 'users' AS 'User' ON 'Tasks'.'user_id' = 'User'.'id';
===
curl -X GET --header 'Accept: application/json' --header 'fields: id, User.id, User.username' 'http://localhost:3000/tasks'
####SQL
SELECT
'Tasks'.'id',
'User'.'id' AS 'User.id',
'User'.'username' AS 'User.username'
FROM 'tasks' AS 'Tasks'
LEFT OUTER JOIN 'users' AS 'User' ON 'Tasks'.'user_id' = 'User'.'id';
####Response Headers { "allowing-fields": "id,descriptions,observation,User.id,User.username,User.firstName,User.lastName,User.email", ... }
FAQs
Dynamic fields for query
The npm package hapi-sequelize-dynamic-fields receives a total of 2 weekly downloads. As such, hapi-sequelize-dynamic-fields popularity was classified as not popular.
We found that hapi-sequelize-dynamic-fields demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.