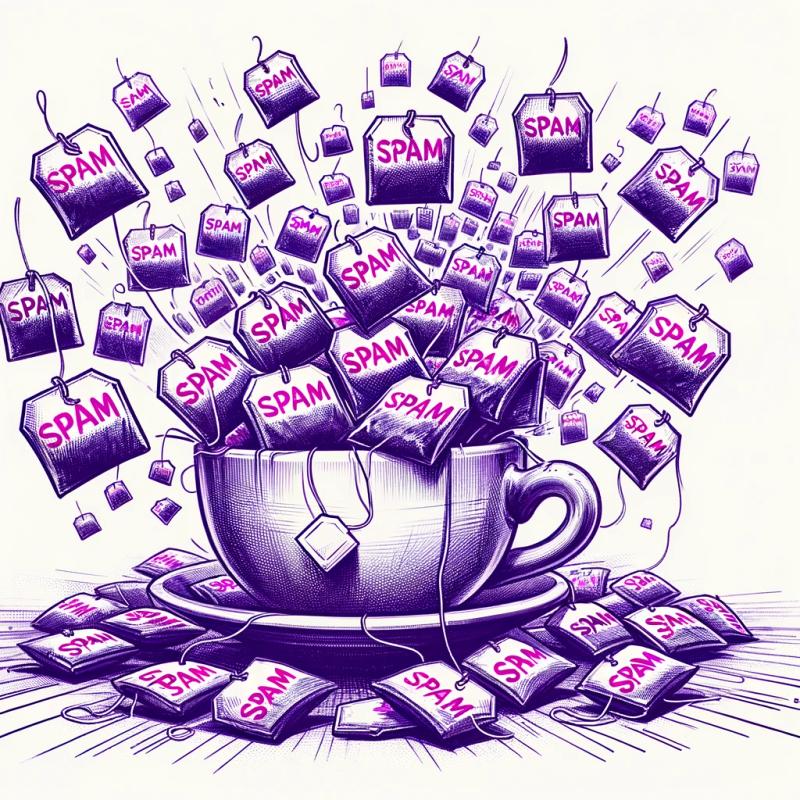
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
hermesjs
Advanced tools
Readme
HermesJS
Build message-driven APIs with ease.
npm install hermesjs
Create a simple app to receive messages from a MQTT broker:
const Hermes = require('hermesjs');
const MqttAdapter = require('hermesjs-mqtt');
const app = new Hermes();
app.add(MqttAdapter, {
host_url: 'mqtt://test.mosquitto.org',
topics: 'hello/#'
});
app.use((message, next) => {
console.log(message.payload);
try {
message.payload = JSON.parse(message.payload);
next(null, message);
} catch (e) {
next('Message payload must be in JSON format');
}
});
app.use((err, message, next) => {
console.error(err);
console.error('Received payload:');
console.error(message.payload);
next();
});
app.connect();
const Hermes = require('hermesjs');
const app = new Hermes();
Adds a connection adapter. Adapters are built independently, as Node.js packages.
For instance, using a MQTT example:
const MqttAdapter = require('hermesjs-mqtt');
app.add(MqttAdapter, {
host_url: 'mqtt://test.mosquitto.org',
topics: 'hello/#'
});
Use middlewares and routes. If you know how Connect/Express works, it's exactly the same, but instead of getting req
and res
, you get a message
object.
Middlewares:
app.use((message, next) => {
console.log(message.payload);
// > '{"key": "value", "key2": 5}'
message.payload = JSON.parse(message.payload);
// Pass the modified message as the second
// argument to forward it to the next middleware.
next(null, message);
});
app.use((message, next) => {
// Now `message.payload` is an object.
console.log(message.payload);
// > { key: 'value', key2: 5 }
next();
});
Routes:
app.use('hello/:name', (message, next) => {
console.log(`Hello ${message.params.name}!`);
next();
});
HermesRouter
index.js
const hello = require('./routes/hello');
app.use('hello', hello);
routes/hello.js
const Router = require('hermesjs/lib/router');
const router = new Router();
router.use(':name', (message, next) => {
console.log(`Hello ${message.params.name}!`);
next();
});
router.use('world', (message, next) => {
console.log(`Hello world!`);
next();
});
module.exports = router;
Catch Errors
app.use((err, message, next) => {
console.log('Handle error here...');
next(err); // Optionally forward error to next middleware
});
This is the same as app.use
but for the outbound communication. The middlewares you specify here will be used before sending a message to the server or broker.
app.use((message, next) => {
// Set `sentAt` attribute to every message before they are sent.
message.payload.sentAt = Date.now();
next(null, message);
});
It sends a message to the server. The message will go through all the outbound middlewares before it reaches the adapters.
app.send('Hello!', {}, 'hello/guest');
Starts the application and connects to the server using the adapters.
app.connect();
You can also use the
app.listen()
alias.
Replies back to the server.
app.use('hello/:name', (message, next) => {
message.reply('Hello server!', undefined, `hello/${message.params.name}/response`);
});
Object containing all the params in the message topic, i.e.:
app.in.broker.use('hello/:name/:surname', (message, next) => {
// Given the `hello/tim/burton` topic, the params will look like:
// message.params == {
// name: 'tim',
// surname: 'burton'
// }
}
});
Fran Méndez (fmvilas.com)
FAQs
Real-time messaging framework
The npm package hermesjs receives a total of 258 weekly downloads. As such, hermesjs popularity was classified as not popular.
We found that hermesjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.