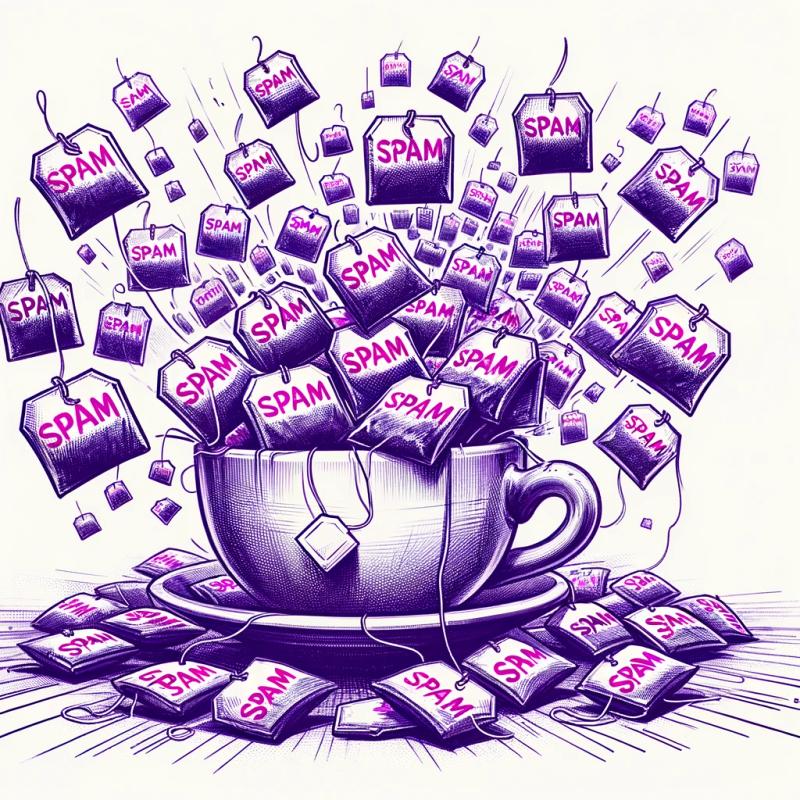
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
hicsail-mongo-models
Advanced tools
Readme
Map JavaScript classes to MongoDB collections. This project was forked from mongo-models. We also added additional function and features that is need for out team. Check them out in the release notes.
MongoDB's native driver for Node.js is pretty good. We just want a little sugar on top.
Mongoose is awesome, and big. It's built on top of MongoDB's native Node.js driver. It's a real deal ODM with tons of features. You should check it out.
We wanted something in between the MongoDB driver and Mongoose. A light weight abstraction where we can interact with collections via JavaScript classes and get document results as instances of those classes.
We're also big fans of the object schema validation library joi. Joi works well for defining a model's data schema.
$ npm install hicsail-mongo-models
You extend the MongoModels
class to create new model classes that map to
MongoDB collections. The base class also acts as a singleton so models share
one connection per process.
Let's create a Customer
model.
const Joi = require('joi');
const MongoModels = require('mongo-models');
class Customer extends MongoModels {
static create(name, email, phone, callback) {
const document = {
name,
email,
phone
};
this.insertOne(document, callback);
}
speak() {
console.log(`${this.name}: call me at ${this.phone}.`);
}
}
Customer.collection = 'customers'; // the mongodb collection name
Customer.schema = Joi.object().keys({
name: Joi.string().required(),
email: Joi.string().email(),
phone: Joi.string()
});
module.exports = Customer;
const Customer = require('./customer');
const Express = require('express');
const MongoModels = require('mongo-models');
const app = Express();
MongoModels.connect(process.env.MONGODB_URI, {}, (err, db) => {
if (err) {
// TODO: throw error or try reconnecting
return;
}
// optionally, we can keep a reference to db if we want
// access to the db connection outside of our models
app.db = db;
console.log('Models are now connected to mongodb.');
});
app.post('/customers', (req, res) => {
const name = req.body.name;
const email = req.body.email;
const phone = req.body.phone;
Customer.create(name, email, phone, (err, customers) => {
if (err) {
res.status(500).json({ error: 'something blew up' });
return;
}
res.json(customers[0]);
});
});
app.get('/customers', (req, res) => {
const filter = {
name: req.query.name
};
Customer.find(filter, (err, customers) => {
if (err) {
res.status(500).json({ error: 'something blew up' });
return;
}
res.json(customers);
});
});
app.server.listen(process.env.PORT, () => {
console.log(`Server is running on port ${process.env.PORT}`);
});
See the API reference.
Any issues or questions (no matter how basic), open an issue. Please take the initiative to read relevant documentation and be pro-active with debugging.
Contributions are welcome. If you're changing something non-trivial, you may want to submit an issue before creating a large pull request. Also Look at the original repo mongo-models and also contribute back to the original repo as well!
MIT
FAQs
Map JavaScript classes to MongoDB collections
The npm package hicsail-mongo-models receives a total of 0 weekly downloads. As such, hicsail-mongo-models popularity was classified as not popular.
We found that hicsail-mongo-models demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.