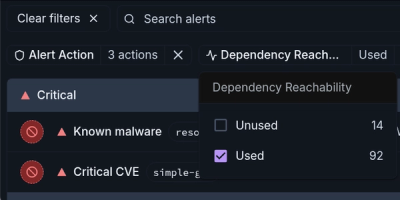
Product
Introducing Module Reachability: Focus on the Vulnerabilities That Matter
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
html-form-validation
Advanced tools
This module is to validate HTML forms. Text fields, emails, phones, checkobxes etc.
Install validator module
npm i -save html-form-validation
Add validator to your project
AMD
require(['html-form-validation'], function (Validator) {});
CommonJS
var Validator = require('html-form-validation');
ES6
import Validator from 'html-form-validation';
Inline
<script src="html-form-validation.js"></script>
Also, include CSS file
<link href="validator.css" rel="stylesheet">
Validator module needs proper HTML-markup (more in example section)
<form>
<!--Email field-->
<label class="form-input" data-validation="required" data-validation-type="email">
<input type="email">
<div class="error"></div>
</label>
<!--Text field. With min and max length (can be only 'min' or only 'max')-->
<label class="form-input" data-validation="required" data-validation-type="text">
<textarea data-validation-condition="length" data-min-length="50" data-max-length="200"></textarea>
<div class="error"></div>
</label>
<!--Text field. With custom error message, 'equal' codition-->
<label class="form-input" data-validation="required" data-validation-type="text" data-validation-text="Incorrect data">
<input type="text" data-validation-condition="equal" data-equal="dataToCompare">
<div class="error"></div>
</label>
<!--Validate form button-->
<button class="validate-form-button" type="submit">Validate form</button>
</form>
Initialize validator module
/**
* First param. Form to validate.
*
* @type {jQuery|HTMLElement|String}
*/
var form = $('form');
/**
* Second param. Function performed after validation (if the form is valid).
* Should return options for ajax request performed after.
*
* @type {Function|Object}
* @return {Object} options for ajax request
*/
var onSuccess = function (context) {
//this code runs before ajax request
var data = getDataFunc();
return {
url: 'ajax/example.json',
method: 'get',
data: data,
success: function () {
console.log('ajax success callback');
}
}
};
/**
* Third param. User-specified options. Unnecessary.
*
* @type {Object}
*/
var options = {
// If the form is situated in bootstrap's modal (e.g. login form),
// incorrect state will be removed from fields when modal is closed.
// DEFAULT: false
modal: false,
// Form fields selector.
// When changing this, you should also change CSS styles.
// DEFAULT: '.form-input'.
fieldsSelector: '.form-input',
// Remove fields incorrect state, when clicked outside the form.
// DEFAULT: false.
removeOnFocusOut: false,
// Perform AJAX request with specified options (if the form is valid).
// DEFAULT: true.
ajax: true,
// Language support. (en/ru)
// DEFAULT: 'en'
lang: 'en'
};
/** Initialize Validator */
new Validator(form, onSuccess, options);
/** Or initialize with jQuery */
form.validator(onSuccess, options);
On success function can perform async actions before returning ajax options object (only ES6 version)
const onSuccess = context => {
// function should return Promise
return new Promise((resolve, reject) => {
// perform async actions. main ajax request will be performed when promise resolve
$.ajax({
url: 'path/to/async/action',
method: 'get',
// remember, function must return options for AJAX request
success: data => resolve({
url: 'path/to/main/ajax',
method: 'post',
data,
success: res => console.log(res)
}),
error: xhr => reject(xhr)
});
})
};
Option name | Possible values | Description |
---|---|---|
data-validation | required / false | Validates the field when set to true. |
data-validation-type | text / phone / email / checkbox / radio / select | Which method used to validate field. Each type has its own. |
data-validation-text | any string | Text used as error message. Otherwise validator will use its own messages for every field type. |
Type | Description | Available input types |
---|---|---|
text | Validates text field. Input can have additional attribute data-validation-condition with available length and equal values. If set to length - Validator will look for data-min-length, data-max-length or data-length attributes. If set to equal - Validator will look for data-equal attribute. Then validator will match value with values from attributes. | input / textarea |
phone | Under development. No additional options are available. | input |
Validates email field. No additional options are available. | input | |
checkbox | At least one checkbox in field should be checked and visible. No additional options are available. | input[type="checkbox"] |
radio | At lease one radio should be selected. No additional options are available. | input[type="radio"] |
select | Checks for selected option. Its value should not equal 0 or false. No additional options are available. | select |
Run tests
npm run-script runTest
Current version is 0.1.61
FAQs
HTML forms validation plugin
The npm package html-form-validation receives a total of 3 weekly downloads. As such, html-form-validation popularity was classified as not popular.
We found that html-form-validation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
Company News
Socket is bringing best-in-class reachability analysis into the platform — cutting false positives, accelerating triage, and cementing our place as the leader in software supply chain security.
Product
Socket is introducing a new way to organize repositories and apply repository-specific security policies.