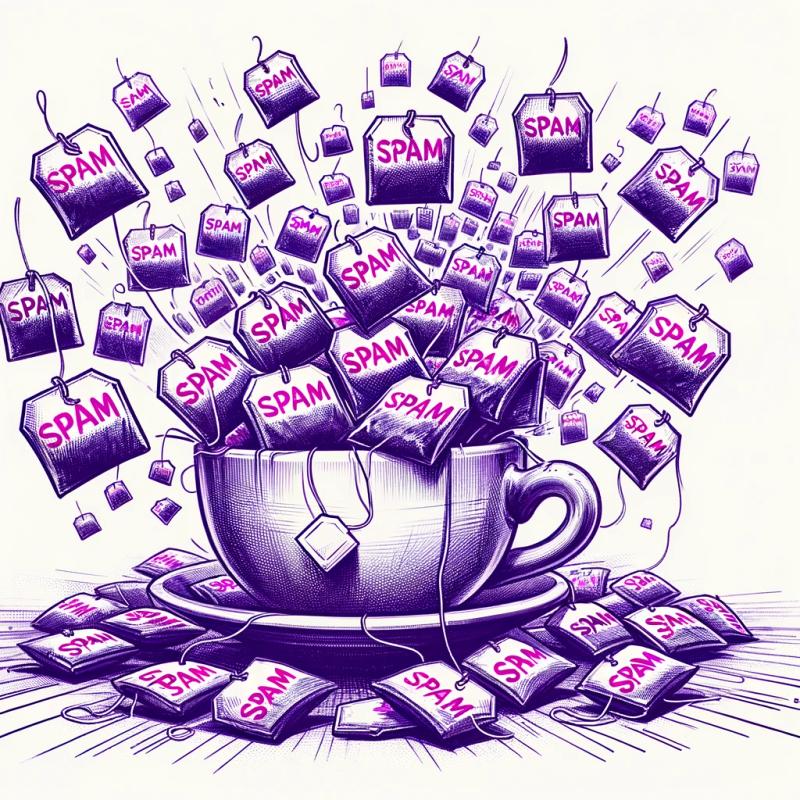
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
htmldom-latest
Advanced tools
Readme
const createHtmlDom = require('htmldom')
let $ = createHtmlDom('<div><button>1</button><a href="https">2</a></div>')
$('*').each((index, item) => {
let $item = $(item)
})
$('div button').addClass('title').attr('k', 'v')
$('a').attr('href')
$('div').find('a').attr('data-id', '5')
// Get last html code
$.html()
npm install htmldom --save
npm run test
Open test.html with browser
npm run umd
selector
w3c selector, support list
let $ = createHtmlDom('html code')
$('*').each((index, item) => {
// Origin dom data
console.log(item)
// Like jQuery object
let $el = $(item)
})
$('div .class a')
$('.item > *')
$('div + p')
$('.item ~ p')
$('.item > a')
$('.wrap .item')
$('#id li')
$('[title]').attr('key', 'value').addClass('cls').removeClass('cls2');
support jQuery method list
$('').length;
$('')[0] // first element
$('')[1] // second
$('').hasClass('cls');
$('').addClass('cls');
$('').addClass('cls1 cls2');
$('').removeClass() // remove all class
$('').removeClass('one') // remove one
$('').removeClass('one two') // remove multiple
$('').attr('key', 'value') // assign
$('').attr('key', function(index,oldValue) {});
$('').attr({
k: 'v',
'data-id': 'v2',
k3: null
}); // multiple assign
$('').attr('key', null) // remove attr
$('').parent()
$('').parent('.cls')
$('').html() // get html
$('').html('12') // set html
$('div').outerHTML()
$('#id').clone()
$('div').replaceWith('<view></view>')
$('').append('<h3>title');
$('').before('<h3>title');
$('#title').prev()
$('#title').prev('.selected')
$('#title').next().next('.selected')
$('').remove();
$('').css('height'); // get
$('').css('height', '200px'); // set
$('').css('height', null); // remove
$('').css({
});
$('div').find('.item > a')
$('').filter('[data-id=1]')
$('').filter(function(index) {
return $(this[index]).attr('data-id') == 1;
});
$('').eq(0) // first element
$('').eq(-1) // last element
$('').each(function(index, item) {
var $item = $(item);
});
Get a dom tree
/**
* <div id="test" class="title header" style="color:red;width:200px;"></div>
*/
{
type: 'tag',
name: 'div',
attributes: {
class: 'title header',
id: 'test',
style: 'color:red;width:200px;'
},
parent: null,
children: [],
classList: new Set(['title', 'header']),
style: {
color: 'red',
width: '200px'
}
}
/**
* raw tag (script, style, textarea)
* <script>alert(1)</script>
*/
{
type: 'tag',
name: 'script',
tagType: 'rawTag',
textContent: 'alert(1)'
}
/**
* selfClosingTag
* <image src="" />
*/
{
type: 'tag',
name: 'image',
attributes: { src: '' },
tagType: 'selfClosingTag'
}
/**
* voidTag
* <input>
*/
{
type: 'tag',
name: 'input',
tagType: 'voidTag',
}
/**
* text tag
*/
{
type: 'text',
data: 'text tag'
}
/**
* <!-- comemnt data -->
*/
{
type: 'comment',
data: ' comemnt data '
}
let $ = createHtmlDom('<div></div>')
$.root().prepend('<head></head>')
// true
$.root().find('div')[0] === $('div')[0]
// '<head></head><div></div>'
$.html()
If you want get html string fast, choose this api, it's only output origin html code
$.html()
It will return compressed html code
options
[options.removeAttributeQuotes=false]
<div id="test"></div> => <div id=test></div>
// Uglify inline script like this
$('script').each((index, item) => {
let $item = $(item)
let type = $item.attr('type')
let src = $item.attr('src')
if ((type && type !== 'text/javascript') || src) return
// Find a uglify plugin by yourself
item.textContent = uglifyJs(item.textContent)
})
// Uglify inline style like this
$('style').each((index, item) => {
let type = $(item).attr('type')
if (type && type !== 'text/css') return
// Find a uglify plugin by yourself
item.textContent = uglifyCss(item.textContent)
})
$.uglify()
$.uglify({
removeAttributeQuotes: true
})
It will return beauty html code
options
[options.indent=' ']
code indent$.beautify({
indent: ' '
});
FAQs
Simplified html handle in nodejs
The npm package htmldom-latest receives a total of 86 weekly downloads. As such, htmldom-latest popularity was classified as not popular.
We found that htmldom-latest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.