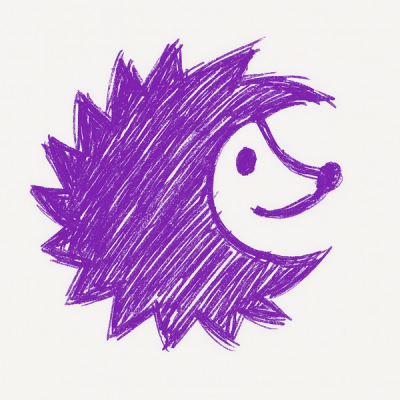
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
http-status-codes
Advanced tools
Constants enumerating the HTTP status codes. Based on the Java Apache HttpStatus API.
The http-status-codes npm package provides an easy-to-use collection of HTTP status codes and reason phrases, which are useful when writing server-side code. It helps developers to avoid hardcoding numeric status codes and instead use descriptive constants, improving code readability and maintainability.
Status Code Enumeration
Provides an enumeration of HTTP status codes, allowing developers to use descriptive constants instead of numeric codes.
const { StatusCodes } = require('http-status-codes');
console.log(StatusCodes.OK); // 200
console.log(StatusCodes.NOT_FOUND); // 404
console.log(StatusCodes.INTERNAL_SERVER_ERROR); // 500
Reason Phrase Lookup
Allows developers to get the standard reason phrase for a given HTTP status code.
const { getReasonPhrase } = require('http-status-codes');
console.log(getReasonPhrase(200)); // 'OK'
console.log(getReasonPhrase(404)); // 'Not Found'
console.log(getReasonPhrase(500)); // 'Internal Server Error'
Status Code Lookup
Enables developers to retrieve the numeric status code for a given reason phrase.
const { getStatusCode } = require('http-status-codes');
console.log(getStatusCode('OK')); // 200
console.log(getStatusCode('Not Found')); // 404
console.log(getStatusCode('Internal Server Error')); // 500
A utility to interact with HTTP status codes. It provides similar functionality to http-status-codes, allowing for both numeric code and text message retrieval. It also supports looking up codes based on the message.
Another simple package that provides HTTP status codes and messages. It is similar to http-status-codes but with a smaller footprint, offering a basic mapping between codes and their default messages.
Constants enumerating the HTTP status codes. Based on the Java Apache HttpStatus API.
All status codes defined in RFC1945 (HTTP/1.0, RFC2616 (HTTP/1.1), and RFC2518 (WebDAV) are supported.
####Installation
npm install http-status-codes
####Usage
HttpStatus = require('httpstatus');
response.send(HttpStatus.OK);
response.send(
HttpStatus.INTERNAL_SERVER_ERROR,
{ error: HttpStatus.getStatusText(HttpStatus.INTERNAL_SERVER_ERROR) }
);
FAQs
Constants enumerating the HTTP status codes. Based on the Java Apache HttpStatus API.
The npm package http-status-codes receives a total of 2,524,069 weekly downloads. As such, http-status-codes popularity was classified as popular.
We found that http-status-codes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.