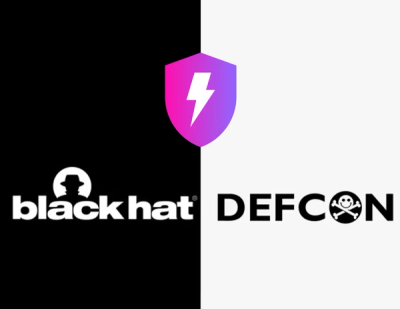
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
http-vue-loader
Advanced tools
Load .vue files directly from your html/js. No node.js environment, no build step.
Load .vue files directly from your html/js. No node.js environment, no build step.
my-component.vue
<template>
<div class="hello">Hello {{who}}</div>
</template>
<script>
module.exports = {
data: function() {
return {
who: 'world'
}
}
}
</script>
<style>
.hello {
background-color: #ffe;
}
</style>
myFile.html
using httpVueLoader()
...
<script type="text/javascript">
new Vue({
components: {
'my-component': httpVueLoader('my-component.vue')
},
...
or, using httpVueLoaderRegister()
...
<script type="text/javascript">
httpVueLoaderRegister(Vue, 'my-component.vue');
new Vue({
components: [
'my-component'
},
...
or, using httpVueLoader
as a plugin
...
<script type="text/javascript">
Vue.use(httpVueLoader);
new Vue({
components: {
'my-component': 'url:my-component.vue'
},
...
or, using an array
new Vue({
components: [
'url:my-component.vue'
},
...
![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|
Latest ✔ | Latest ✔ | ? | ? | Latest ✔ | 9+ ✔ |
url
)url
: any url to a .vue file
vue
, url
)vue
: a Vue instance
url
: any url to a .vue file
url
)This is the default httpLoader.
Use axios instead of the default http loader:
httpVueLoader.httpRequest = function(url) {
return axios.get(url)
.then(function(res) {
return res.data;
})
.catch(function(err) {
return Promise.reject(err.status);
});
}
The aim of http-vue-loader is to quickly test .vue components without any compilation step.
However, for production, I recommend to use webpack module bundler with vue-loader,
see also why Vue.js doesn't support templateURL.
Note also that http-vue-loader
only supports text/x-template for <template>
, text/javascript for <script>
and text/css for <style>
.
<template>
, <script>
and <style>
does not support the src
attribute yet.
<style scoped>
is functional.
Due to the lack of suitable API in Google Chrome and Internet Explorer, syntax errors in the <script>
section are only reported on FireFox.
FAQs
Load .vue files directly from your html/js. No node.js environment, no build step.
The npm package http-vue-loader receives a total of 426 weekly downloads. As such, http-vue-loader popularity was classified as not popular.
We found that http-vue-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.