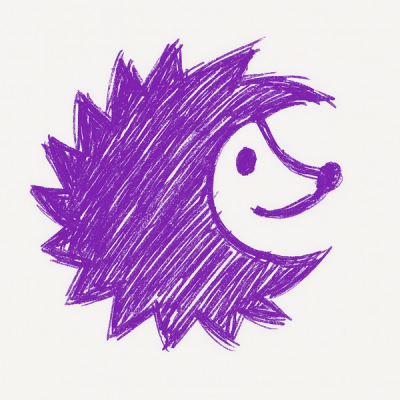
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Hydra is a runnable server implementation of the OAuth2 2.0 authorization framework and the OpenID Connect Core 1.0.
Hydra-js is a client library for javascript. It is currently available as an npm-module only. At this moment, Hydra-js primarily helps you with performing the consent validation. We welcome contributions that implement more of the Hydra HTTP REST API.
$ npm i --save hydra-js
var Hydra = require('hydra-js')
const config = {
client: {
id: process.env.HYDRA_CLIENT_ID, // id of the client you want to use, defaults to this env var
secret: process.env.HYDRA_CLIENT_SECRET, // secret of the client you want to use, defaults to this env var
},
auth: {
tokenHost: process.env.HYDRA_URL, // hydra url, defaults to this env var
authorizePath: '/oauth2/auth', // hydra authorization endpoint, defaults to '/oauth2/auth'
tokenPath: '/oauth2/token', // hydra token endpoint, defaults to '/oauth2/token'
},
scope: 'hydra.keys.get' // scope of the authorization, defaults to 'hydra.keys.get'
}
const hydra = new Hydra(config)
var Hydra = require('hydra-js')
const hydra = new Hydra(/* options */)
hydra.authenticate().then((token) => {
// ...
}).catch((error) => {
// ...
})
The following examples fetches the appropriate cryptographic keys and access tokens automatically, you basically need to do:
var Hydra = require('hydra-js')
const hydra = new Hydra(/* options */)
// verify consent challenge
hydra.verifyConsentChallenge(challenge).then(({ challenge: data }) => {
// consent challenge is valid, render the consent screen:
// w.render('consent', { data })
}).catch((error) => {
// error
})
// generate consent challenge
hydra.generateConsentResponse(challenge, subject, scopes, {}, data).then(({ consent }) => {
// success! redirect back to hydra:
// w.redirect(challenge.redir + '&consent=' + consent)
}).catch((error) => {
// error
})
FAQs
A client library for Hydra, an OAuth2 and OpenID Connect Provider
We found that hydra-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.