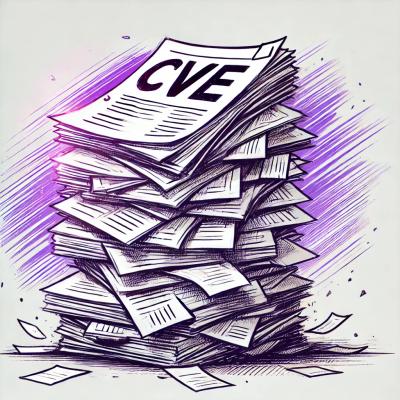
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
i18next-http-backend
Advanced tools
i18next-http-backend is a backend layer for i18next using in Node.js, in the browser and for Deno.
The i18next-http-backend package is a backend layer for the i18next internationalization framework, allowing you to load translations from a remote server via HTTP(S). It is particularly useful for applications that need to fetch translations dynamically from a server, making it easier to manage and update translations without redeploying the application.
Loading Translations from a Remote Server
This feature allows you to load translation files from a remote server. The `loadPath` option specifies the URL pattern to fetch the translation files, where `{{lng}}` and `{{ns}}` are placeholders for the language and namespace, respectively.
const i18next = require('i18next');
const HttpBackend = require('i18next-http-backend');
i18next
.use(HttpBackend)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json'
}
});
Customizing HTTP Requests
This feature allows you to customize the HTTP requests used to fetch the translation files. The `requestOptions` object can include any options that are valid for the Fetch API, such as `mode`, `credentials`, and `cache`.
const i18next = require('i18next');
const HttpBackend = require('i18next-http-backend');
i18next
.use(HttpBackend)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
requestOptions: {
mode: 'cors',
credentials: 'same-origin',
cache: 'default'
}
}
});
Handling Load Errors
This feature allows you to handle errors that occur during the loading of translation files. The `request` function can be customized to handle different types of errors and provide appropriate responses.
const i18next = require('i18next');
const HttpBackend = require('i18next-http-backend');
i18next
.use(HttpBackend)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
request: (options, url, payload, callback) => {
fetch(url, options)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => callback(null, { status: '200', data }))
.catch(error => callback(error, { status: '500' }));
}
}
});
The i18next-fs-backend package is another backend layer for i18next, but it loads translations from the file system instead of a remote server. This is useful for server-side applications where translations are stored locally. Compared to i18next-http-backend, it does not support dynamic loading from a remote server.
The i18next-localstorage-backend package allows you to cache translations in the browser's local storage. This can improve performance by reducing the number of HTTP requests needed to fetch translations. However, it does not provide the same level of dynamic loading from a remote server as i18next-http-backend.
The i18next-xhr-backend package is similar to i18next-http-backend but uses XMLHttpRequest instead of the Fetch API to load translations. It provides similar functionality but may be less modern and flexible compared to i18next-http-backend, which leverages the Fetch API.
This is a simple i18next backend to be used in Node.js, in the browser and for Deno. It will load resources from a backend server using the XMLHttpRequest or the fetch API.
Get a first idea on how it is used in this i18next crash course video.
It's based on the deprecated i18next-xhr-backend and can mostly be used as a drop-in replacement.
Why i18next-xhr-backend was deprecated?
If you don't like to manage your translation files manually or are simply looking for a better management solution, take a look at i18next-locize-backend. The i18next backed plugin for 🌐 locize ☁️.
To see i18next-locize-backend in a working app example, check out:
Make sure you set the debug
option of i18next to true
. This will maybe log more information in the developer console.
Seeing failed http requests, like 404?
Are you using a language detector plugin that detects region specific languages you are not providing? i.e. you provide 'en'
translations but you see a 'en-US'
request first?
This is because of the default load
option set to 'all'
.
Try to set the load
option to 'languageOnly'
i18next.init({
load: 'languageOnly',
// other options
})
Source can be loaded via npm or downloaded from this repo.
There's also the possibility to directly import it via a CDN like jsdelivr or unpkg or similar.
# npm package
$ npm install i18next-http-backend
Wiring up:
import i18next from 'i18next';
import HttpApi from 'i18next-http-backend';
i18next.use(HttpApi).init(i18nextOptions);
for Deno:
import i18next from 'https://deno.land/x/i18next/index.js'
import Backend from 'https://deno.land/x/i18next_http_backend/index.js'
i18next.use(Backend).init(i18nextOptions);
for plain browser:
<script src="https://cdn.jsdelivr.net/npm/i18next-http-backend@1.3.1/i18nextHttpBackend.min.js"></script>
<!-- an example can be found in example/jquery/index.html -->
i18next.use(i18nextHttpBackend).init(i18nextOptions);
window.i18nextHttpBackend
{
// path where resources get loaded from, or a function
// returning a path:
// function(lngs, namespaces) { return customPath; }
// the returned path will interpolate lng, ns if provided like giving a static path
// the function might return a promise
// returning falsy will abort the download
//
// If not used with i18next-multiload-backend-adapter, lngs and namespaces will have only one element each,
// If used with i18next-multiload-backend-adapter, lngs and namespaces can have multiple elements
// and also your server needs to support multiloading
// /locales/resources.json?lng=de+en&ns=ns1+ns2
// Adapter is needed to enable MultiLoading https://github.com/i18next/i18next-multiload-backend-adapter
// Returned JSON structure in this case is
// {
// lang : {
// namespaceA: {},
// namespaceB: {},
// ...etc
// }
// }
loadPath: '/locales/{{lng}}/{{ns}}.json',
// path to post missing resources, or a function
// function(lng, namespace) { return customPath; }
// the returned path will interpolate lng, ns if provided like giving a static path
//
// note that this only works when initialized with { saveMissing: true }
// (see https://www.i18next.com/overview/configuration-options)
addPath: '/locales/add/{{lng}}/{{ns}}',
// parse data after it has been fetched
// in example use https://www.npmjs.com/package/json5 or https://www.npmjs.com/package/jsonc-parser
// here it removes the letter a from the json (bad idea)
parse: function(data) { return data.replace(/a/g, ''); },
// parse data before it has been sent by addPath
parsePayload: function(namespace, key, fallbackValue) { return { key: fallbackValue || "" } },
// parse data before it has been sent by loadPath
// if value returned it will send a POST request
parseLoadPayload: function(languages, namespaces) { return undefined },
// allow cross domain requests
crossDomain: false,
// allow credentials on cross domain requests
withCredentials: false,
// overrideMimeType sets request.overrideMimeType("application/json")
overrideMimeType: false,
// custom request headers sets request.setRequestHeader(key, value)
customHeaders: {
authorization: 'foo',
// ...
},
// can also be a function, that returns the headers
customHeaders: () => ({
authorization: 'foo',
// ...
}),
requestOptions: { // used for fetch, can also be a function (payload) => ({ method: 'GET' })
mode: 'cors',
credentials: 'same-origin',
cache: 'default'
},
// define a custom request function
// can be used to support XDomainRequest in IE 8 and 9
//
// 'options' will be this entire options object
// 'url' will be passed the value of 'loadPath'
// 'payload' will be a key:value object used when saving missing translations
// 'callback' is a function that takes two parameters, 'err' and 'res'.
// 'err' should be an error
// 'res' should be an object with a 'status' property and a 'data' property containing a stringified object instance beeing the key:value translation pairs for the
// requested language and namespace, or null in case of an error.
request: function (options, url, payload, callback) {},
// adds parameters to resource URL. 'example.com' -> 'example.com?v=1.3.5'
queryStringParams: { v: '1.3.5' },
reloadInterval: false // can be used to reload resources in a specific interval (milliseconds) (useful in server environments)
}
Options can be passed in:
preferred - by setting options.backend in i18next.init:
import i18next from 'i18next';
import HttpApi from 'i18next-http-backend';
i18next.use(HttpApi).init({
backend: options,
});
on construction:
import HttpApi from 'i18next-http-backend';
const HttpApi = new HttpApi(null, options);
via calling init:
import HttpApi from 'i18next-http-backend';
const HttpApi = new HttpApi();
HttpApi.init(null, options);
To properly type the backend options, you can import the HttpBackendOptions
interface and use it as a generic type parameter to the i18next's init
method, e.g.:
import i18n from 'i18next'
import HttpBackend, { HttpBackendOptions } from 'i18next-http-backend'
i18n
.use(HttpBackend)
.init<HttpBackendOptions>({
backend: {
// http backend options
},
// other i18next options
})
From the creators of i18next: localization as a service - locize.com
A translation management system built around the i18next ecosystem - locize.com.
With using locize you directly support the future of i18next.
FAQs
i18next-http-backend is a backend layer for i18next using in Node.js, in the browser and for Deno.
The npm package i18next-http-backend receives a total of 771,781 weekly downloads. As such, i18next-http-backend popularity was classified as popular.
We found that i18next-http-backend demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.