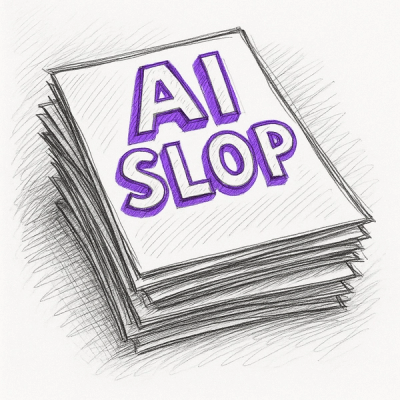
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
if-else-throw
Advanced tools
If X, return Y, else throw Z.
X can be a boolean or a function.
Requires Node.js 6.0.0 or above.
npm i if-else-throw
The module exports a single function.
test
(any): The “if” condition, evaluated for truthiness. If a function is provided, it is called with val
as its only argument and its return value is used.val
(any): The “then” value to return if test
is truthy. If omitted, test
is returned.error
(Error or string): The Error to throw if test
is falsely. If a string is provided, it is wrapped in a new Error
. If omitted, defaults to new Error()
.If test
is truthy, returns val
(or returns test
if val
is omitted). If test
is falsey, there is no return value because an error is thrown.
const ifElseThrow = require('if-else-throw')
const value = []
ifElseThrow(
Array.isArray(value), // The boolean condition (in this case, true)
value, // The value returned if true
new TypeError('Not an array') // The error thrown if false
) // []
ifElseThrow(
x => Array.isArray(x), // x will be the second argument ('this is a string')
'this is a string', // Given to the function and returned if the function returns true
new TypeError('Not an array') // The error thrown if false
) // Uncaught TypeError: Not an array
ifElseThrow(
'this is truthy',
'Must be truthy'
) // 'this is truthy'
ifElseThrow(
null,
'Must be truthy'
) // Uncaught Error: Must be truthy
FAQs
If X, return Y, else throw Z.
The npm package if-else-throw receives a total of 1,066 weekly downloads. As such, if-else-throw popularity was classified as popular.
We found that if-else-throw demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.