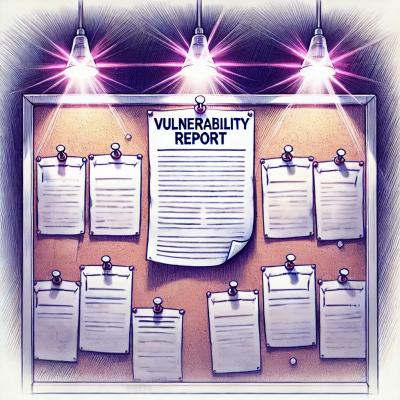
Security News
libxml2 Maintainer Ends Embargoed Vulnerability Reports, Citing Unsustainable Burden
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
imagemin-svgo
Advanced tools
The imagemin-svgo npm package is a plugin for Imagemin that uses SVGO (SVG Optimizer) to compress and optimize SVG files. It helps reduce the file size of SVG images by removing unnecessary data without affecting the visual quality.
Basic SVG Optimization
This code demonstrates how to use imagemin-svgo to optimize SVG files in the 'images' directory and save the optimized files to the 'output/images' directory.
const imagemin = require('imagemin');
const imageminSvgo = require('imagemin-svgo');
(async () => {
await imagemin(['images/*.svg'], {
destination: 'output/images',
plugins: [
imageminSvgo()
]
});
console.log('SVG images optimized');
})();
Custom SVGO Options
This code shows how to use imagemin-svgo with custom SVGO options. In this example, the 'removeViewBox' and 'cleanupIDs' plugins are configured to preserve the viewBox attribute and IDs in the SVG files.
const imagemin = require('imagemin');
const imageminSvgo = require('imagemin-svgo');
(async () => {
await imagemin(['images/*.svg'], {
destination: 'output/images',
plugins: [
imageminSvgo({
plugins: [
{ removeViewBox: false },
{ cleanupIDs: false }
]
})
]
});
console.log('SVG images optimized with custom options');
})();
SVGO (SVG Optimizer) is a standalone tool for optimizing SVG files. It provides a command-line interface and can be used as a library in Node.js applications. While imagemin-svgo is a plugin for Imagemin, svgo can be used independently for more direct control over SVG optimization.
svgmin is another tool for optimizing SVG files. It is similar to svgo but offers different optimization strategies and configurations. svgmin can be used as a command-line tool or integrated into build processes. It provides an alternative to imagemin-svgo with its own set of features and options.
SVGO imagemin plugin
npm install imagemin-svgo
import imagemin from 'imagemin';
import imageminSvgo from 'imagemin-svgo';
await imagemin(['images/*.svg'], {
destination: 'build/images',
plugins: [
imageminSvgo({
plugins: [{
name: 'removeViewBox',
active: false
}]
})
]
});
console.log('Images optimized');
Returns a Promise<Buffer>
.
Type: object
Pass options to SVGO.
Type: Buffer
The buffer to optimize.
FAQs
SVGO imagemin plugin
The npm package imagemin-svgo receives a total of 374,271 weekly downloads. As such, imagemin-svgo popularity was classified as popular.
We found that imagemin-svgo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Libxml2’s solo maintainer drops embargoed security fixes, highlighting the burden on unpaid volunteers who keep critical open source software secure.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.