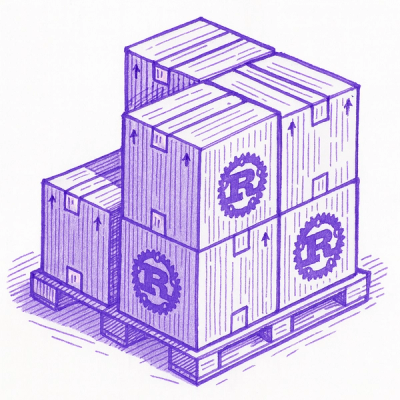
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
immutable-object-methods
Advanced tools
Update normal plain javascript object, immutable style. Simlar to how immutable.js, seamless-immutable etc does it but a lot smaller and simpler.
Update normal plain javascript object, immutable style. Simlar to how immutable.js, seamless-immutable etc does it but a lot smaller and simpler.
This is a Node.js module available through the
npm registry. It can be installed using the
npm
or
yarn
command line tools.
npm install immutable-object-methods --save
import {
setIn,
mergeDeep,
assign,
set,
without,
} from 'immutable-object-methods';
const input = { a: { b: 'c' } };
const updated = setIn(input, ['a', 'd'], 'e');
console.log(input);
console.log(updated);
const merged = mergeDeep({ foo: 'bar' }, { beep: { boop: 4711 }, foo: 'bas' });
console.log(merged);
// immutable assign
const assigned = assign({ foo: 'bar' }, { foz: 'baz' });
console.log(assigned);
const data = set({ beep: 'boop' }, 'foo', 'bar');
console.log(data);
const beep = without({ foo: 'bar' }, 'foo');
console.log(beep);
npm install
npm test
None
MIT
FAQs
Update normal plain javascript object, immutable style. Simlar to how immutable.js, seamless-immutable etc does it but a lot smaller and simpler.
The npm package immutable-object-methods receives a total of 2,145 weekly downloads. As such, immutable-object-methods popularity was classified as popular.
We found that immutable-object-methods demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.