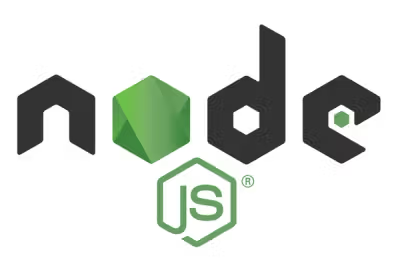
Security News
Node.js TSC Votes to Stop Distributing Corepack
Corepack will be phased out from future Node.js releases following a TSC vote.
injectivejs
Advanced tools
Injectivejs is a JavaScript library for interacting with injective sdk.
npm install injectivejs
import { createQueryRpc } from "@interchainjs/cosmos/utils";
import { createGetAllBalances } from "injectivejs/cosmos/bank/v1beta1/query.rpc.func";
import { createGetExchangeBalances } from "injectivejs/injective/exchange/v1beta1/query.rpc.func";
{ getRpcEndpoint } = useChain("injective");
const endpoint = await getRpcEndpoint();
const rpc = createQueryRpc(endpoint);
// get the tree shakable helper functions using the rpc client
const getAllBalances = createGetAllBalances(rpc);
// now you can query the cosmos modules
const balance = await getAllBalances({
address: "inj1addresshere",
});
// you can also query the injective modules
const getExchangeBalances = createGetExchangeBalances(rpc);
const exchangeBalance = await getExchangeBalances({});
For tx messages, there're helper functions to sign and broadcast messages:
For more detailed usage on how to use these functions, please see the starship tests in the networks/injective repo
There're also react and vue hooks for helper functions. Please see injective-react and injective-vue repos for more information.
import {
createDeposit,
createLiquidatePosition,
createActivateStakeGrant,
} from "injectivejs/injective/exchange/v1beta1/tx.rpc.func";
import { createBid } from "injectivejs/injective/auction/v1beta1/tx.rpc.func";
import {
createActivateStakeGrant,
createAdminUpdateBinaryOptionsMarket,
createAuthorizeStakeGrants,
createBatchCancelBinaryOptionsOrders,
createBatchCancelDerivativeOrders,
createBatchCancelSpotOrders,
createBatchCreateDerivativeLimitOrders,
createBatchCreateSpotLimitOrders,
createBatchUpdateOrders,
createCancelBinaryOptionsOrder,
createCancelDerivativeOrder,
createCancelSpotOrder,
createCreateBinaryOptionsLimitOrder,
createCreateBinaryOptionsMarketOrder,
createCreateDerivativeLimitOrder,
createCreateDerivativeMarketOrder,
createCreateSpotLimitOrder,
createCreateSpotMarketOrder,
createDecreasePositionMargin,
createDeposit,
createEmergencySettleMarket,
createExternalTransfer,
createIncreasePositionMargin,
createInstantBinaryOptionsMarketLaunch,
createInstantExpiryFuturesMarketLaunch,
createInstantPerpetualMarketLaunch,
createInstantSpotMarketLaunch,
createLiquidatePosition,
createPrivilegedExecuteContract,
createRewardsOptOut,
createSubaccountTransfer,
createUpdateDerivativeMarket,
createUpdateParams,
createUpdateSpotMarket,
createWithdraw,
} from "injectivejs/injective/exchange/v1beta1/tx.rpc.func";
import {
createCreateInsuranceFund,
createRequestRedemption,
createUnderwrite,
} from "injectivejs/injective/insurance/v1beta1/tx.rpc.func";
import {
createAcceptPayeeship,
createCreateFeed,
createFundFeedRewardPool,
createSetPayees,
createTransferPayeeship,
createTransmit,
createUpdateFeed,
createWithdrawFeedRewardPool,
} from "injectivejs/injective/ocr/v1beta1/tx.rpc.func";
import {
createRelayBandRates,
createRelayCoinbaseMessages,
createRelayPriceFeedPrice,
createRelayProviderPrices,
createRelayPythPrices,
createRelayStorkMessage,
createRequestBandIBCRates,
} from "injectivejs/injective/oracle/v1beta1/tx.rpc.func";
import {
createBlacklistEthereumAddresses,
createCancelSendToEth,
createConfirmBatch,
createDepositClaim,
createERC20DeployedClaim,
createRequestBatch,
createRevokeEthereumBlacklist,
createSendToEth,
createSetOrchestratorAddresses,
createSubmitBadSignatureEvidence,
createValsetConfirm,
createValsetUpdateClaim,
createWithdrawClaim,
} from "injectivejs/injective/peggy/v1/msgs.rpc.func";
import {
createExecuteContract,
createClearAdmin,
createInstantiateContract,
createInstantiateContract2,
createMigrateContract,
createPinCodes,
createRemoveCodeUploadParamsAddresses,
createStoreAndInstantiateContract,
createStoreCode,
createSudoContract,
createUnpinCodes,
createUpdateAdmin,
createUpdateContractLabel,
createUpdateInstantiateConfig,
createAddCodeUploadParamsAddresses,
createStoreAndMigrateContract,
} from "injectivejs/cosmwasm/wasm/v1/tx.rpc.func";
import { createTransfer } from "injectivejs/ibc/applications/transfer/v1/tx.rpc.func";
import {
createFundCommunityPool,
createCommunityPoolSpend,
createDepositValidatorRewardsPool,
} from "injectivejs/cosmos/distribution/v1beta1/tx.rpc.func";
import {
createSend,
createMultiSend,
} from "injectivejs/cosmos/bank/v1beta1/tx.rpc.func";
import {
createDelegate,
createUndelegate,
createCancelUnbondingDelegation,
createCreateValidator,
} from "injectivejs/cosmos/staking/v1beta1/tx.rpc.func";
import {
createDeposit,
createSubmitProposal,
createVote,
createVoteWeighted,
} from "injectivejs/cosmos/gov/v1beta1/tx.rpc.func";
Import the injective
object from injectivejs
.
import { MessageComposer } from "injectivejs/injective/exchange/v1beta1/tx.registry";
const { createSpotLimitOrder, createSpotMarketOrder, deposit } =
MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/auction/v1beta1/tx.registry";
const { bid } = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/exchange/v1beta1/tx.registry";
const {
adminUpdateBinaryOptionsMarket,
batchCancelBinaryOptionsOrders,
batchCancelDerivativeOrders,
batchCancelSpotOrders,
batchCreateDerivativeLimitOrders,
batchCreateSpotLimitOrders,
batchUpdateOrders,
cancelBinaryOptionsOrder,
cancelDerivativeOrder,
cancelSpotOrder,
createBinaryOptionsLimitOrder,
createBinaryOptionsMarketOrder,
createDerivativeLimitOrder,
createDerivativeMarketOrder,
createSpotLimitOrder,
createSpotMarketOrder,
deposit,
exec,
externalTransfer,
increasePositionMargin,
instantBinaryOptionsMarketLaunch,
instantExpiryFuturesMarketLaunch,
instantPerpetualMarketLaunch,
instantSpotMarketLaunch,
liquidatePosition,
rewardsOptOut,
subaccountTransfer,
withdraw,
} = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/insurance/v1beta1/tx.registry";
const { createInsuranceFund, requestRedemption, underwrite } =
MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/ocr/v1beta1/tx.registry";
const {
acceptPayeeship,
createFeed,
fundFeedRewardPool,
setPayees,
transferPayeeship,
transmit,
updateFeed,
withdrawFeedRewardPool,
} = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/oracle/v1beta1/tx.registry";
const {
relayBandRates,
relayCoinbaseMessages,
relayPriceFeedPrice,
relayProviderPrices,
requestBandIBCRates,
} = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/injective/peggy/v1/tx.registry";
const {
cancelSendToEth,
confirmBatch,
depositClaim,
eRC20DeployedClaim,
requestBatch,
sendToEth,
setOrchestratorAddresses,
submitBadSignatureEvidence,
valsetConfirm,
valsetUpdateClaim,
withdrawClaim,
} = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/cosmwasm/wasm/v1/tx.registry";
const {
clearAdmin,
executeContract,
instantiateContract,
migrateContract,
storeCode,
updateAdmin,
} = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/ibc/applications/transfer/v1/tx.registry";
const { transfer } = MessageComposer.withTypeUrl;
import { MessageComposer } from "injectivejs/cosmos/distribution/v1beta1/tx.registry";
const {
fundCommunityPool,
setWithdrawAddress,
withdrawDelegatorReward,
withdrawValidatorCommission,
} = MessageComposer.fromPartial;
import { MessageComposer } from "injectivejs/cosmos/bank/v1beta1/tx.registry";
const { multiSend, send } = MessageComposer.fromPartial;
import { MessageComposer } from "injectivejs/cosmos/staking/v1beta1/tx.registry";
const {
beginRedelegate,
createValidator,
delegate,
editValidator,
undelegate,
} = MessageComposer.fromPartial;
import { MessageComposer } from "injectivejs/cosmos/gov/v1beta1/tx.registry";
const { deposit, submitProposal, vote, voteWeighted } =
cosmos.gov.v1beta1.MessageComposer.fromPartial;
⚡️ For web interfaces, we recommend using interchain-kit. Continue below to see how to manually construct signers and clients.
Here are the docs on creating signers in interchain-kit that can be used with Keplr and other wallets.
Use SigningClient.connectWithSigner and pass in the signer options for injective to get your SigningClient
:
import { SigningClient } from "@interchainjs/cosmos/signing-client";
import { defaultSignerOptions } from "@interchainjs/injective/defaults";
const signingClient = await SigningClient.connectWithSigner(
await getRpcEndpoint(),
new AminoGenericOfflineSigner(aminoOfflineSigner),
{
signerOptions: defaultSignerOptions.Cosmos,
}
);
To broadcast messages, you can create signers with a variety of options:
When you have your signing client
, you can broadcast messages:
const msg = {
typeUrl: MsgSend.typeUrl,
value: MsgSend.fromPartial({
amount: [
{
denom: "inj",
amount: "1000",
},
],
toAddress: address,
fromAddress: address,
}),
};
const fee: StdFee = {
amount: [
{
denom: "inj",
amount: "864",
},
],
gas: "86364",
};
const response = await signingClient.signAndBroadcast(address, [msg], fee);
For a comprehensive example of how to use InjectiveJS to send messages, please see the example here. This example demonstrates how to:
The example provides a complete walkthrough of setting up the client, creating a message for sending tokens, and broadcasting the transaction to the Injective blockchain.
Follow the instructions in the example to set up your InjectiveJS client and start sending messages to the Injective blockchain.
If you want to manually construct a signing client, you can do so by following the example below:
import {
cosmosAminoConverters,
cosmosProtoRegistry,
cosmwasmAminoConverters,
cosmwasmProtoRegistry,
ibcProtoRegistry,
ibcAminoConverters,
injectiveAminoConverters,
injectiveProtoRegistry
} from 'injectivejs';
const signer: OfflineSigner = /* create your signer (see above) */
const rpcEndpoint = 'https://rpc.cosmos.directory/injective'; // or another URL
const protoRegistry: ReadonlyArray<[string, GeneratedType]> = [
...cosmosProtoRegistry,
...cosmwasmProtoRegistry,
...ibcProtoRegistry,
...injectiveProtoRegistry
];
const aminoConverters = {
...cosmosAminoConverters,
...cosmwasmAminoConverters,
...ibcAminoConverters,
...injectiveAminoConverters
};
const registry = new Registry(protoRegistry);
const aminoTypes = new AminoTypes(aminoConverters);
const signingClient = await SigningClient.connectWithSigner(rpcEndpoint, signer);
signingClient.addEncoders(registry);
signingClient.addConverters(aminoTypes);
When first cloning the repo:
yarn
yarn build:dev
Contract schemas live in ./contracts
, and protos in ./proto
. Look inside of scripts/inj.telescope.json
and configure the settings for bundling your SDK and contracts into injectivejs
:
yarn codegen
Build the types and then publish:
yarn build
yarn publish
A unified toolkit for building applications and smart contracts in the Interchain ecosystem
Category | Tools | Description |
---|---|---|
Chain Information | Chain Registry, Utils, Client | Everything from token symbols, logos, and IBC denominations for all assets you want to support in your application. |
Wallet Connectors | Interchain Kitbeta, Cosmos Kit | Experience the convenience of connecting with a variety of web3 wallets through a single, streamlined interface. |
Signing Clients | InterchainJSbeta, CosmJS | A single, universal signing interface for any network |
SDK Clients | Telescope | Your Frontend Companion for Building with TypeScript with Cosmos SDK Modules. |
Starter Kits | Create Interchain Appbeta, Create Cosmos App | Set up a modern Interchain app by running one command. |
UI Kits | Interchain UI | The Interchain Design System, empowering developers with a flexible, easy-to-use UI kit. |
Testing Frameworks | Starship | Unified Testing and Development for the Interchain. |
TypeScript Smart Contracts | Create Hyperweb App | Build and deploy full-stack blockchain applications with TypeScript |
CosmWasm Contracts | CosmWasm TS Codegen | Convert your CosmWasm smart contracts into dev-friendly TypeScript classes. |
🛠 Built by Hyperweb (formerly Cosmology) — if you like our tools, please checkout and contribute to our github ⚛️
AS DESCRIBED IN THE LICENSES, THE SOFTWARE IS PROVIDED “AS IS”, AT YOUR OWN RISK, AND WITHOUT WARRANTIES OF ANY KIND.
No developer or entity involved in creating this software will be liable for any claims or damages whatsoever associated with your use, inability to use, or your interaction with other users of the code, including any direct, indirect, incidental, special, exemplary, punitive or consequential damages, or loss of profits, cryptocurrencies, tokens, or anything else of value.
FAQs
Injectivejs is a JavaScript library for interacting with injective sdk.
The npm package injectivejs receives a total of 34 weekly downloads. As such, injectivejs popularity was classified as not popular.
We found that injectivejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.
Research
Security News
Research uncovers Black Basta's plans to exploit package registries for ransomware delivery alongside evidence of similar attacks already targeting open source ecosystems.
Security News
Oxlint's beta release introduces 500+ built-in linting rules while delivering twice the speed of previous versions, with future support planned for custom plugins and improved IDE integration.