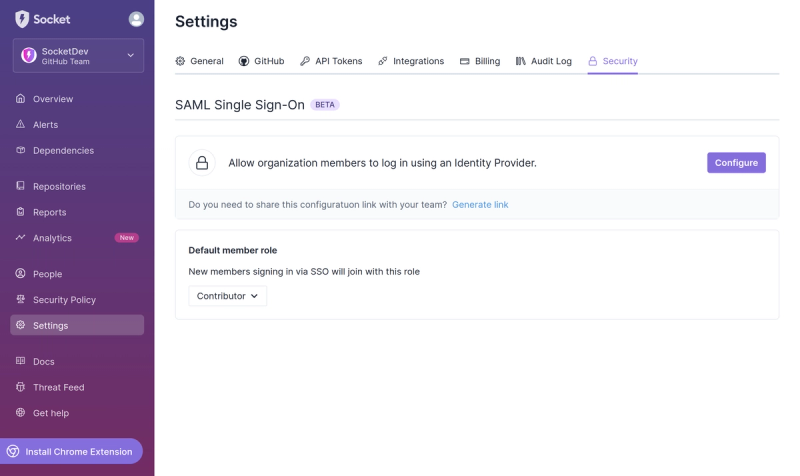
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
ip-location
Advanced tools
Readme
Fetch the location of an IP address, host name, or your own location.
Uses freegeoip.net to query for information. freegeoip.net uses MaxMind Geolite 2.
npm i --save ip-location
var ipLocation = require('ip-location')
ipLocation('github.com', function (err, data) {
console.log(data)
})
Outputs:
{ ip: '192.30.252.129',
country_code: 'US',
country_name: 'United States',
region_code: 'CA',
region_name: 'California',
city: 'San Francisco',
zip_code: '94107',
time_zone: 'America/Los_Angeles',
latitude: 37.7697,
longitude: -122.3933,
metro_code: 807 }
var ipLocation = require('ip-location')
ipLocation('github.com')
.then(function (data) {
console.dir(data)
})
.catch(function (err) {
console.error(err)
})
You can set your own Promise library if you want to use Bluebird or are using Node v0.10.
var ipLocation = require('ip-location')
ipLocation.Promise = require('bluebird')
If you want to use this in the browser, you must bring your own http GET library to the party. I'd recommend: xhr or fetch.
var ipLocation = require('ip-location')
ipLocation.httpGet = function (url, callback) {
fetch(url).then(function (resp) {
return resp.text() // don't use json()
}).then(function (text) {
resp.body = text // body should always be set to a string
callback(null, resp)
}).catch(function (err) {
callback(err)
})
}
Just pass in an empty string.
ipLocation('', function (err, myLocation) {
console.dir(myLocation)
})
MIT - Copyright (c) JP Richardson
FAQs
Get an IP or hostname location.
The npm package ip-location receives a total of 544 weekly downloads. As such, ip-location popularity was classified as not popular.
We found that ip-location demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.