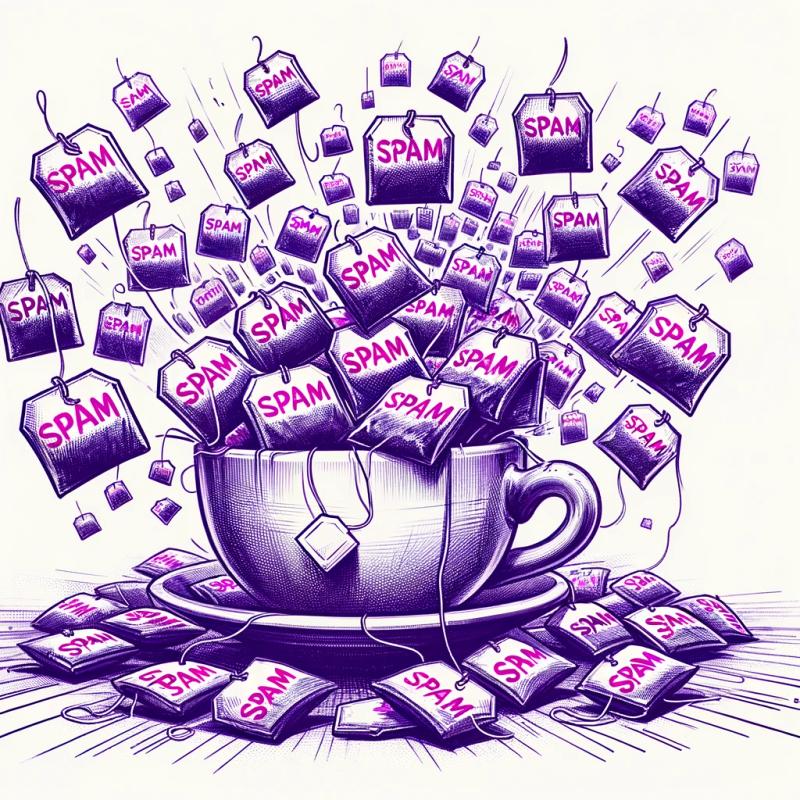
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
itemsapi-node
Advanced tools
Readme
Node.js client for ItemsAPI - node.js & elasticsearch search server for web and mobile. For more low level info go to documentation
$ npm install itemsapi-node --save
var ItemsAPI = require('itemsapi-node');
var client = new ItemsAPI('http://yourLocalOrHerokuUrl.com/api/v1', 'cities');
var facets = {
country:['Canada', 'India']
};
client.search({
sort: 'most_votes',
query: '',
page: 1,
aggs: facets,
per_page: 12
}).then(function(res) {
console.log(res);
//console.log(JSON.stringify(res, null, 2));
})
Example response:
{ meta: { query: '', sort: 'most_votes', search_time: 20 },
pagination: { page: '1', per_page: '12', total: 67 },
data:
{ items:
[ [Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object],
[Object] ],
aggregations: { country: [Object], distance_ranges: [Object] },
sortings: { country: [Object], distance: [Object], city: [Object] }
}
}
client.similar('item-id', {
fields: ['tags']
}).then(function(res) {
console.log(res);
})
client.getItem(id)
.then(function(result) {
console.log(result);
})
client.addItem(data)
.then(function(result) {
console.log(result);
})
client.deleteItem(id)
.then(function(result) {
console.log(result);
})
client.addBulkItems(cities)
.then(function(res) {
console.log('added ' + cities.length + 'elements');
})
client.getCollection()
.then(function(result) {
console.log(result);
})
Example response:
{
name: 'cities',
type: 'cities',
index: 'cities',
meta: { title: 'Cities' },
defaults: { sort: 'city' },
schema: {
city: { type: 'string', store: true, index: 'not_analyzed' },
province: { type: 'string', store: true },
province_icon: { type: 'string', display: 'image' },
country: { type: 'string', index: 'not_analyzed', store: true },
country_icon: { type: 'string', display: 'image' },
geo: { type: 'geo_point' }
},
sortings: {
country: {
title: 'Country',
type: 'normal',
order: 'asc',
field: 'country'
},
distance: { title: 'Distance', type: 'geo', order: 'asc', field: 'geo' },
city: { title: 'City', type: 'normal', order: 'asc', field: 'city' }
},
table: {},
aggregations: {
country: { type: 'terms', field: 'country', size: 10, title: 'Country' },
distance_ranges: {
type: 'geo_distance',
field: 'geo',
ranges: [Object],
unit: 'km',
title: 'Distance ranges [km]'
}
}
}
client.addCollection(collection)
.then(function(result) {
console.log(result);
})
client.getCollections()
.then(function(result) {
console.log(result);
})
client.partialUpdateCollection({
index: 'new-index-name',
type: 'new-type-name',
})
.then(function(result) {
console.log(result);
})
FAQs
Node.js client for ItemsAPI
The npm package itemsapi-node receives a total of 11 weekly downloads. As such, itemsapi-node popularity was classified as not popular.
We found that itemsapi-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.