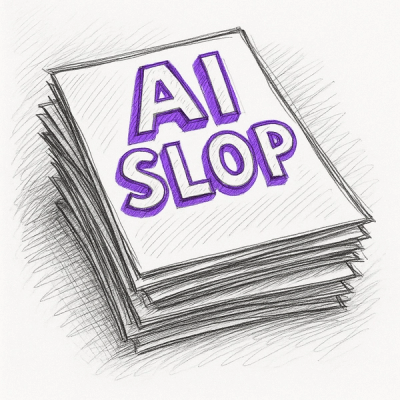
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Basic operations on synchronous + asynchronous iterables, strictly for JavaScript native types.
We do not use any synthetic types / wrappers here, like Observable
in RXJS, etc. It is strictly an iterable on the
input, and an iterable on the output, for maximum performance, simplicity and compatibility (see Rationale).
Related repo: iter-ops-extras - addition to the main API.
$ npm i iter-ops
import {pipe, filter, map} from 'iter-ops';
const input = [1, 2, 3, 4, 5];
const i = pipe(
input,
filter((a) => a % 2 === 0), // find even numbers
map((value) => ({value})) // remap into objects
);
console.log(...i); //=> {value: 2}, {value: 4}
import {pipe, toAsync, distinct, delay} from 'iter-ops';
const input = [1, 2, 2, 3, 3, 4];
const i = pipe(
toAsync(input), // make asynchronous
distinct(), // emit unique numbers
delay(1000) // delay each value by 1s
);
// or you can replace `pipe` + `toAsync` with just `pipeAsync`
(async function () {
for await (const a of i) {
console.log(a); //=> 1, 2, 3, 4 (with 1s delay)
}
})();
See also...
Function pipe takes any iterable, applies all specified operators to it, and returns an extended iterable. For strict type of iterables, there are also pipeSync and pipeAsync.
All standard operators implement the same logic as Array does:
See iter-ops-extras - a collection of custom operators (ones based on existing operators).
FAQs
Basic operations on iterables
The npm package iter-ops receives a total of 6,310 weekly downloads. As such, iter-ops popularity was classified as popular.
We found that iter-ops demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.