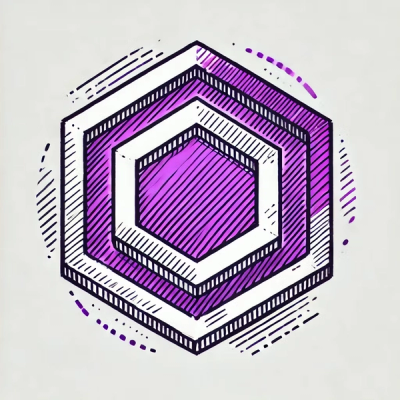
Security News
ESLint Adds Official Support for Linting HTML
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
iteration-typeguards
Advanced tools
typeguard functions to determine whether or not a value is iterable or an iterator in the ES6 sense
Install with npm
npm install iteration-typeguards
This module assumes a javascript environment implementing ES6, as it defines iterators and iterability based on the ES6 iteration protocols.
This module consists of two typeguard functions, isIterable
and isIterator
that accept
any javascript value as a single argument and return a boolean for whether or not that
value is a Iterable
or an Iterator
in the ES6 sense, respectively.
A typescript compiler --target
argument of es6
should be used. Both of the functions
isIterable
and isIterator
are defined as typescript typeguards for the built-in
iterfaces: Iterable<T>
and Iterator<T>
, respectively.
import {isIterable} from "iteration-typeguards";
isIterable(5); // false
isIterable<string>("oh hai"); // true
isIterable(null); // false
isIterable([1,2,3]); // true
isIterable(new Map<any,any>([
["hi", 3],
[{water: true}, "summer"]]
)); // true
isIterable({
[Symbol.iterator]: function() {
return {
next: function() {
return 47;
},
done: false
}
}
}); // true
import {isIterator} from "iteration-typeguards";
isIterator(5); // false
isIterator<string>("oh hai"); // false
isIterator<string>("oh hai"[Symbol.iterator]());// true
isIterator(null); // false
isIterator([1,2,3]); // false
isIterator([1,2,3][Symbol.iterator]); // true
isIterator({
next: function() {
return 47;
},
done: false
}); // true
Usage without typescript in nodejs is very similar to the typescript examples above without the type annotations. Since an ES6 environment is assumed, the import statements above should work. The more traditional nodejs require statements shown below also work.
var itGuards = require("iteration-typeguards");
itGuards.isIterable([1,2,3]) // true
itGuards.isIterator([1,2,3][Symbol.iterator]) // true
If you come across a case in which these typeguards do not perform as expected, please submit an issue (outlining the case) or a pull request (again, outlining the case and a possible solution).
MIT --- open source
FAQs
typeguard functions to determine whether or not a value is iterable or an iterator in the ES6 sense
The npm package iteration-typeguards receives a total of 0 weekly downloads. As such, iteration-typeguards popularity was classified as not popular.
We found that iteration-typeguards demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.