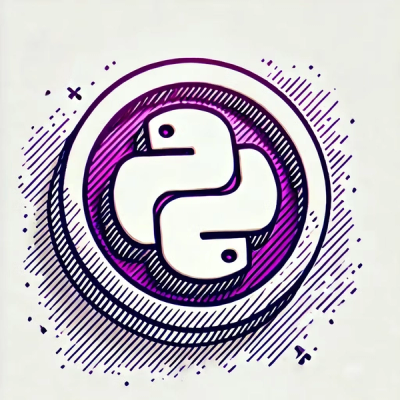
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
js13k-vite-plugins
Advanced tools
Collection of [Vite](https://vitejs.dev/) plugins and utilities for [js13k games](https://js13kgames.com/).
Collection of Vite plugins and utilities for js13k games.
Included tools:
Example project: https://github.com/codyebberson/js13k-starter
Go to the js13k-starter repository and click "Use this template" to create a new repository with the same files and folders as the template.
Clone the new repository and install the dependencies:
git clone git@github.com:your-username/js13k-starter.git
cd js13k-starter
npm install
Then run the development server:
npm run dev
First, setup a new Vite project: https://vitejs.dev/guide/#scaffolding-your-first-vite-project
npm create vite@latest
Next, add js13k-vite-plugins
:
npm install --save-dev js13k-vite-plugins
Then update your Vite configuration as needed. See below for example Vite configurations.
Example vite.config.ts
files
Use js13kViteConfig()
for a quick and easy default configuration (recommended).
// vite.config.ts
import { js13kViteConfig } from "js13k-vite-plugins";
import { defineConfig } from "vite";
export default defineConfig(js13kViteConfig());
Some plugins can be disabled individually by passing false
for the options.
roadrollerOptions: false
advzipOptions: false
For example, disable Roadroller:
// vite.config.ts
import { js13kViteConfig } from "js13k-vite-plugins";
import { defineConfig } from "vite";
export default defineConfig(
js13kViteConfig({
roadrollerOptions: false,
})
);
Pass in options to configure specific plugins.
For example, change the Advzip shrink level to "fast" (from default "insane").
// vite.config.ts
import { js13kViteConfig } from "js13k-vite-plugins";
import { defineConfig } from "vite";
export default defineConfig(
js13kViteConfig({
advzipOptions: {
shrinkLevel: "fast",
},
})
);
Use the individual plugins for more control over the build.
// vite.config.ts
import {
advzipPlugin,
ectPlugin,
getDefaultViteBuildOptions,
roadrollerPlugin,
} from "js13k-vite-plugins";
import { defineConfig } from "vite";
export default defineConfig({
build: getDefaultViteBuildOptions(),
plugins: [roadrollerPlugin(), ectPlugin(), advzipPlugin()],
});
The top level options is a collection of options for the various sub-plugins.
export interface JS13KOptions {
viteOptions?: BuildOptions;
terserOptions?: Terser.MinifyOptions;
rollupOptions?: RollupOptions;
htmlMinifyOptions?: HtmlMinifyOptions;
roadrollerOptions?: RoadrollerOptions;
ectOptions?: EctOptions;
advzipOptions?: AdvzipOptions;
}
Base package: vite
Full Vite documentation: https://vitejs.dev/config/build-options.html
Default options:
/**
* Returns recommended Vite build options for a JS13K game.
*
* Features:
* - Targets ESNext
* - Minifies with Terser
* - Disables CSS code splitting
* - Disables Vite polyfills
* - Uses recommended Terser options
* - Uses recommended Rollup options
*
* @returns The recommended Vite build options.
*/
export const defaultViteBuildOptions: BuildOptions = {
target: "esnext",
minify: "terser",
cssCodeSplit: false,
modulePreload: {
polyfill: false, // Don't add vite polyfills
},
terserOptions: defaultTerserOptions,
rollupOptions: defaultRollupOptions,
};
Base package: terser
Full Terser documentation: https://terser.org/docs/options/
Default options:
/**
* Returns recommended Terser options for a JS13K game.
*
* Features:
* - Targets ESNext
* - Enables all unsafe options
* - Enables all passes
* - Enables all mangles
*/
export const defaultTerserOptions: Terser.MinifyOptions = {
compress: {
ecma: 2020 as ECMA,
module: true,
passes: 3,
unsafe_arrows: true,
unsafe_comps: true,
unsafe_math: true,
unsafe_methods: true,
unsafe_proto: true,
},
mangle: {
module: true,
toplevel: true,
},
format: {
comments: false,
ecma: 2020 as ECMA,
},
module: true,
toplevel: true,
};
Base package: rollup
Full Rollup documentation: https://rollupjs.org/configuration-options/
Default options:
/**
* Returns recommended Rollup options for a JS13K game.
*
* Features:
* - Enables inline dynamic imports
*
* @returns The recommended Rollup options.
*/
export const defaultRollupOptions: RollupOptions = {
output: {
inlineDynamicImports: true,
manualChunks: undefined,
},
};
Base package: html-minifier-terser
Full HTML Minify documentation: https://github.com/terser/html-minifier-terser
Default options:
export const defaultHtmlMinifyOptions: HtmlMinifyOptions = {
includeAutoGeneratedTags: true,
removeAttributeQuotes: true,
removeComments: true,
removeRedundantAttributes: true,
removeScriptTypeAttributes: true,
removeStyleLinkTypeAttributes: true,
sortClassName: true,
useShortDoctype: true,
collapseWhitespace: true,
collapseInlineTagWhitespace: true,
removeEmptyAttributes: true,
removeOptionalTags: true,
sortAttributes: true,
};
Base package: imagemin
params | type | default | default |
---|---|---|---|
verbose | boolean | true | Whether to output the compressed result in the console |
filter | RegExp or (file: string) => boolean | - | Specify which resources are not compressed |
disable | boolean | false | Whether to disable |
svgo | object or false | - | See Options |
gifsicle | object or false | - | See Options |
mozjpeg | object or false | - | See Options |
optipng | object or false | - | See Options |
pngquant | object or false | - | See Options |
webp | object or false | - | See Options |
Base package: roadroller
Full Roadroller documentation: https://lifthrasiir.github.io/roadroller/
This plugin uses the Roadroller defaults.
Base package: ect-bin
ECT documentation: https://github.com/fhanau/Efficient-Compression-Tool/blob/master/doc/Manual.docx (Google Docs)
export interface EctOptions {
/**
* Show no report when program is finished; print only warnings and errors.
*/
quiet?: boolean;
/**
* Select compression level [1-9] (ECT default: 3, JS13k default: 10009).
*
* For detailed information on performance read Performance.html.
*
* Advanced usage:
* A different syntax may be used to achieve even more compression for deflate compression
* if time (and efficiency) is not a concern.
* If the value is above 10000, the blocksplitting-compression cycle is repeated # / 10000 times.
* If # % 10000 is above 9, level 9 is used and the number of iterations of deflate compression
* per block is set to # % 10000. If # % 10000 is 9 or below, this number specifies the level.
*/
level?: number;
/**
* Discard metadata. (default true).
*/
strip?: boolean;
/**
* Keep the file modification time. (default false).
*/
keep?: boolean;
/**
* Enable strict losslessness. Without this, image data under fully transparent pixels can be
* modified to increase compression. This data is normally invisible and not needed.
* However, you may want to use this option if you are still going to edit the image.
*
* Also preserves rarely used GZIP metadata.
*
* (default false).
*/
strict?: boolean;
}
Default options:
/**
* Default Efficient Compression Tool (ECT) options.
*
* Level 10009 is the recommended value for JS13k games. This will be slow but will produce the smallest file size.
*
* Strip is true by default because metadata is not needed for JS13k games.
*/
export const defaultEctOptions: EctOptions = {
level: 10009,
strip: true,
};
Base package: advzip-bin
Full Advzip documentation: https://linux.die.net/man/1/advzip
TypeScript interface:
export interface AdvzipOptions {
pedantic?: boolean;
shrinkLevel?:
| 0
| 1
| 2
| 3
| 4
| "store"
| "fast"
| "normal"
| "extra"
| "insane";
}
Default options:
export const defaultAdvzipOptions: AdvzipOptions = {
shrinkLevel: "insane",
};
Rob Louie for Roadroller configuration recommendations
Salvatore Previti for Terser configuration recommendations
Andrzej Mazur for organizing js13k
MIT
FAQs
Collection of [Vite](https://vitejs.dev/) plugins and utilities for [js13k games](https://js13kgames.com/).
The npm package js13k-vite-plugins receives a total of 0 weekly downloads. As such, js13k-vite-plugins popularity was classified as not popular.
We found that js13k-vite-plugins demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.