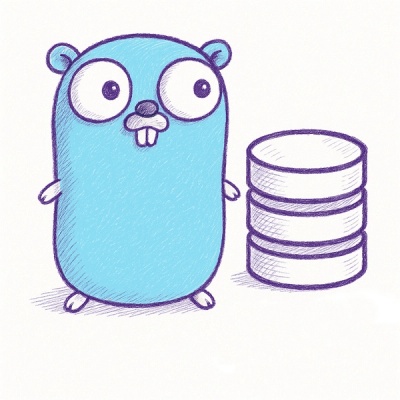
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Simple CSV export module that can export a rich JSON array of objects to CSV. (Formerly json-csv)
Moved to: @iwsio/json-csv
const jsoncsv = require('json-csv')
jsoncsv.buffered(data, options) //returns Promise
//optionally, you can use the callback
jsoncsv.buffered(data, options, (err, csv) => {...}))
let callback = function(err, csv) {
//csv contains Utf8 (or encoding of your choice) string of converted data in CSV format.
}
When using the streaming API, you can pipe data to it in object mode.
const jsoncsv = require('json-csv')
let readable = some_readable_source //<readable source in object mode>
readable
.pipe(jsoncsv.stream(options)) //transforms to Utf8 string and emits lines
.pipe(something_else_writable)
//optionally, you can use the callback
jsoncsv.stream(options, (err, streamToCsv) => {
readable
.pipe(streamToCsv) //transforms to Utf8 string and emits lines
.pipe(something_else_writable)
})
{
//field definitions for CSV export
fields :
[
{
//required: field name for source value
name : 'string',
//required: column label for CSV header
label : 'string',
//optional: filter to transform value before exporting
filter : function(value) { return value; }
}
],
// Other default options:
fieldSeparator: ","
,ignoreHeader: false
,buffered: true
,encoding: "utf8"
}
Simple structure with basic CSV conversion.
const jsoncsv = require('json-csv')
let items = [
{
name : 'fred',
email : 'fred@somewhere',
amount : 1.02
},
{
name : 'jo',
email : 'jo@somewhere',
amount : 1.02
},
{
name : 'jo with a comma,',
email : 'jo@somewhere',
amount : 1.02
},
{
name : 'jo with a quote"',
email : 'jo@somewhere',
amount : 1.02
}]
jsoncsv.buffered(items, {
fields : [
{
name : 'name',
label : 'Name',
quoted : true
},
{
name : 'email',
label : 'Email'
},
{
name : 'amount',
label : 'Amount'
}
]},
(err, csv) => {
console.log(csv);
});
//OR Streaming
let options = {
fields : [
{
name : 'name',
label : 'Name',
quoted : true
},
{
name : 'email',
label : 'Email'
},
{
name : 'amount',
label : 'Amount'
}
]}
Readable.from(items) //node 12
.pipe(jsoncsv.stream(options))
.pipe(process.stdout)
Generates Output:
Name,Email,Amount
"fred",fred@somewhere,1.02
"jo",jo@somewhere,1.02
"jo with a comma,",jo@somewhere,1.02
"jo with a quote""",jo@somewhere,1.02
Here's a little more advanced sample that uses sub-structures and a filter for manipulating output for individual columns.
const jsoncsv = require('json-csv')
let items = [
{
contact : {
company : 'Widgets, LLC',
name : 'John Doe',
email : 'john@widgets.somewhere'
},
registration : {
year : 2013,
level : 3
}
},
{
contact : {
company : 'Sprockets, LLC',
name : 'Jane Doe',
email : 'jane@sprockets.somewhere'
},
registration : {
year : 2013,
level : 2
}
}
];
jsoncsv.buffered(items, {
fields : [
{
name : 'contact.company',
label : 'Company'
},
{
name : 'contact.name',
label : 'Name'
},
{
name : 'contact.email',
label : 'Email'
},
{
name : 'registration.year',
label : 'Year'
},
{
name : 'registration.level',
label : 'Level',
filter : function(value) {
switch(value) {
case 1 : return 'Test 1'
case 2 : return 'Test 2'
default : return 'Unknown'
}
}
}]
},
function(err, csv) {
console.log(csv);
});
Generates Output:
Company,Name,Email,Year,Level
"Widgets, LLC",John Doe,john@widgets.somewhere,2013,Unknown
"Sprockets, LLC",Jane Doe,jane@sprockets.somewhere,2013,Test 2
Pipe to File (Using example above):
const fs = require("fs")
let options = {
fields : [
{
name : 'contact.company',
label : 'Company'
},
{
name : 'contact.name',
label : 'Name'
},
{
name : 'contact.email',
label : 'Email'
},
{
name : 'registration.year',
label : 'Year'
},
{
name : 'registration.level',
label : 'Level',
filter : function(value) {
switch(value) {
case 1 : return 'Test 1'
case 2 : return 'Test 2'
default : return 'Unknown'
}
}
}]
}
let out = fs.createWriteStream("output.csv", {encoding: 'utf8'})
let readable = Readable.from(items) //node 12
readable
.pipe(jsoncsv.stream(options))
.pipe(out)
Example using "OR" || operator to combine two object attributes at once column.
const jsoncsv = require("json-csv");
let items = [
{
name: "White Shoes",
price: 12.10,
category1: "Apparel",
category2: "Bottom Apparel",
category3: "Shoes",
},
{
name: "Grey Pants",
price: 50.30,
category1: "Apparel",
category2: "Bottom Apparel",
category3: "Pants",
},
{
name: "Black Belt",
price: 5.30,
category1: "Apparel",
category2: "Belts",
},
{
name: "Normal Glasses",
price: 10.20,
category1: "Glasses",
},
{
name: "Dark Glasses",
price: 20.30,
category1: "",
category2: "Sunglasses",
},
]
jsoncsv.buffered(
items,
{
fields: [
{
name: "name",
label: "Name",
},
{
name: "price",
label: "Price",
},
{
name: "category1||category2||category3",
label: "Category",
},
],
}, (err, csv) => {
console.log(csv);
}
)
Generates Output:
Name, Price, Category
"White Shoes", 12.10, Shoes
"Grey Pants", 50.30, Pants
"Black Belt", 10.30, Belts
"Normal Glasses", 10.20, Glasses
"Dark Glasses", 20.30, Sunglasses
FAQs
Easily convert JSON array to CSV in Node.JS via buffered or streaming.
The npm package json-csv receives a total of 5,067 weekly downloads. As such, json-csv popularity was classified as popular.
We found that json-csv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.