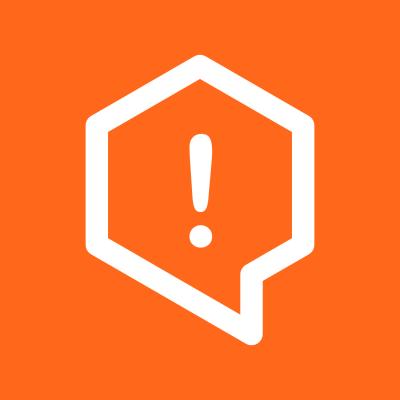
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
json-dup-key-validator
Advanced tools
A json validator that has an option to check for duplicated keys
The json-dup-key-validator npm package is used to validate JSON objects for duplicate keys. It helps ensure that JSON data structures do not contain duplicate keys, which can lead to unexpected behavior in applications.
Validate JSON String
This feature allows you to validate a JSON string to check for duplicate keys. The `validate` function returns an object indicating whether the JSON is valid and lists any duplicate keys found.
const { validate } = require('json-dup-key-validator');
const jsonString = '{ "key1": "value1", "key1": "value2" }';
const result = validate(jsonString);
console.log(result); // Output: { valid: false, duplicateKeys: ['key1'] }
Validate JSON Object
This feature allows you to validate a JSON object directly to check for duplicate keys. The `validateObject` function returns an object indicating whether the JSON object is valid and lists any duplicate keys found.
const { validateObject } = require('json-dup-key-validator');
const jsonObject = { "key1": "value1", "key1": "value2" };
const result = validateObject(jsonObject);
console.log(result); // Output: { valid: false, duplicateKeys: ['key1'] }
jsonlint is a JSON parser and validator with a CLI. It can be used to validate JSON data and provides detailed error messages. Unlike json-dup-key-validator, jsonlint focuses on general JSON validation and formatting rather than specifically identifying duplicate keys.
ajv is a JSON schema validator that supports JSON Schema draft-07 and other standards. It is highly configurable and can be used to validate JSON data against a schema. While ajv is more comprehensive and powerful, it requires defining schemas and is not specifically focused on detecting duplicate keys like json-dup-key-validator.
fast-json-stringify is a library for serializing JSON objects quickly. It uses JSON schema to generate code for fast serialization. Although it is not a validator, it can be used in conjunction with JSON schema validators like ajv to ensure data integrity. It does not specifically address duplicate key validation.
A json validator that has an option to check for duplicated keys
npm install json-dup-key-validator
var jsonValidator = require('json-dup-key-validator');
// Returns error or undefined if json is valid
jsonValidator.validate(jsonString, allowDuplicatedKeys);
// Returns the object and throws error if any
jsonValidator.parse(jsonString, allowDuplicatedKeys);
Validates a json string and returns error if any, undefined if the json string is valid.
Type: String
JSON string to parse
Type: Boolean
Default: false
Whether duplicated keys are allowed in an object or not
Parses a json string and returns the parsed result. Throws error if the json string is not valid.
Type: String
JSON string to parse
Type: Boolean
Default: false
Whether duplicated keys are allowed in an object or not
FAQs
A json validator that has an option to check for duplicated keys
The npm package json-dup-key-validator receives a total of 0 weekly downloads. As such, json-dup-key-validator popularity was classified as not popular.
We found that json-dup-key-validator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.