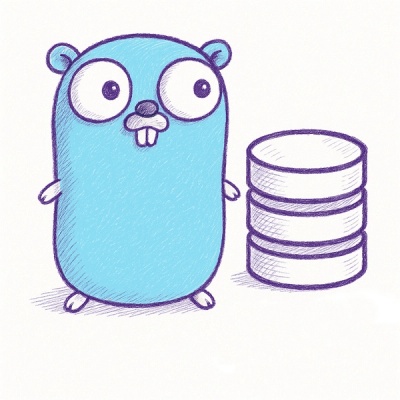
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
json-patch-extended
Advanced tools
Includes everything from Starcounter-Jack's JSON-Patch:
Plus addtional features:
JSON-Patch (RFC6902) is a standard format that allows you to update a JSON document by sending the changes rather than the whole document.
This repo deviates just a bit from that standard, but only with additions. The additions are as follows:
remove
ops include a value property and all replace
ops include a old property. In both cases, the additional property stores the old value at the path. This is an aide for the new reverse method.Install the current version (and save it as a dependency):
$ npm install json-patch-extended --save
$ bower install json-patch-extended --save
Include dist/json-patch-extended.min.js
Call require to get the instance:
var jsonpatch = require('json-patch-extended')
Or use import in ES6:
import jsonpatch from 'json-patch-extended'
Applying patches:
var myobj = { firstName:"Albert", contactDetails: { phoneNumbers: [ ] } };
var patches = [
{op:"replace", path:"/firstName", value:"Joachim" },
{op:"add", path:"/lastName", value:"Wester" },
{op:"add", path:"/contactDetails/phoneNumbers/0", value:{ number:"555-123" } }
];
jsonpatch.apply( myobj, patches );
// myobj == { firstName:"Joachim", lastName:"Wester", contactDetails:{ phoneNumbers[ {number:"555-123"} ] } };
Generating patches:
var myobj = { firstName:"Joachim", lastName:"Wester", contactDetails: { phoneNumbers: [ { number:"555-123" }] } };
var observer = jsonpatch.observe( myobj );
myobj.firstName = "Albert";
myobj.contactDetails.phoneNumbers[0].number = "123";
myobj.contactDetails.phoneNumbers.push({number:"456"});
var patches = jsonpatch.generate(observer);
// patches == [
// { op:"replace", path="/firstName", value:"Albert", old:"Joachim"},
// { op:"replace", path="/contactDetails/phoneNumbers/0/number", value:"123", old:"555-123"},
// { op:"add", path="/contactDetails/phoneNumbers/1", value:{number:"456"}}];
Comparing two object trees:
var objA = {user: {firstName: "Albert", lastName: "Einstein"}};
var objB = {user: {firstName: "Albert", lastName: "Collins"}};
var diff = jsonpatch.compare(objA, objB));
//diff == [{op: "replace", path: "/user/lastName", value: "Collins", old: "Einstein"}]
Validating a sequence of patches:
var obj = {user: {firstName: "Albert"}};
var patches = [{op: "replace", path: "/user/firstName", value: "Albert"}, {op: "replace", path: "/user/lastName", value: "Einstein"}];
var errors = jsonpatch.validate(patches, obj);
if (errors.length == 0) {
//there are no errors!
}
else {
for (var i=0; i < errors.length; i++) {
if (!errors[i]) {
console.log("Valid patch at index", i, patches[i]);
}
else {
console.error("Invalid patch at index", i, errors[i], patches[i]);
}
}
}
Reversing patches:
var patches = [
{op:"replace", path:"/firstName", value:"Joachim", old:"Albert" },
{op:"add", path:"/lastName", value:"Wester" },
{op:"remove", path:"/contactDetails/phoneNumbers/0", value:{ number:"555-123" } }
];
jsonpatch.reverse( patches );
// myobj == [
// {op:"replace", path:"/firstName", value:"Albert", old:"Joachim" },
// {op:"remove", path:"/lastName", value:"Wester" },
// {op:"add", path:"/contactDetails/phoneNumbers/0", value:{ number:"555-123" } }
// ];
obj
Object, patches
Array, validate
Boolean) : booleanApplies patches
array on obj
.
If the validate
parameter is set to true
, the patch is extensively validated before applying.
An invalid patch results in throwing an error (see jsonpatch.validate
for more information about the error object).
Returns an array of results - one item for each item in patches
. The type of each item depends on type of operation applied
test
- boolean result of the testremove
, replace
and move
- original object that has been removedadd
(only when adding to an array) - index at which item has been inserted (useful when using -
alias)obj
Object, callback
Function (optional)) : observer
ObjectSets up an deep observer on obj
that listens for changes in object tree. When changes are detected, the optional
callback is called with the generated patches array as the parameter.
Returns observer
.
obj
Object, observer
Object) : patches
ArrayIf there are pending changes in obj
, returns them synchronously. If a callback
was defined in observe
method, it will be triggered synchronously as well.
If there are no pending changes in obj
, returns an empty array (length 0).
obj
Object, observer
Object) : voidDestroys the observer set up on obj
.
Any remaining changes are delivered synchronously (as in jsonpatch.generate
). Note: this is different that ES6/7 Object.unobserve
, which delivers remaining changes asynchronously.
obj1
Object, obj2
Object) : patches
ArrayCompares object trees obj1
and obj2
and returns the difference relative to obj1
as a patches array.
If there are no differences, returns an empty array (length 0).
patches
Array) : patches
ArrayReverses a patches
array. (Pass result to jsonpatch.apply to apply to an object).
Patches are reversed according to the following:
add
- becomes remove
remove
- becomes add
replace
- old and value are switchedcopy
, move
, and test
- ignoredpatches
Array, tree
Object (optional)) : error
JsonPatchErrorValidates a sequence of operations. If tree
parameter is provided, the sequence is additionally validated against the object tree.
If there are no errors, returns undefined. If there is an errors, returns a JsonPatchError object with the following properties:
name
String - short error codemessage
String - long human readable error messageindex
Number - index of the operation in the sequenceoperation
Object - reference to the operationtree
Object - reference to the treePossible errors:
Error name | Error message |
---|---|
SEQUENCE_NOT_AN_ARRAY | Patch sequence must be an array |
OPERATION_NOT_AN_OBJECT | Operation is not an object |
OPERATION_OP_INVALID | Operation op property is not one of operations defined in RFC-6902 |
OPERATION_PATH_INVALID | Operation path property is not a valid string |
OPERATION_FROM_REQUIRED | Operation from property is not present (applicable in move and copy operations) |
OPERATION_VALUE_REQUIRED | Operation value property is not present, or undefined (applicable in add , replace and test operations) |
OPERATION_VALUE_CANNOT_CONTAIN_UNDEFINED | Operation value property object has at least one undefined value (applicable in add , replace and test operations) |
OPERATION_PATH_CANNOT_ADD | Cannot perform an add operation at the desired path |
OPERATION_PATH_UNRESOLVABLE | Cannot perform the operation at a path that does not exist |
OPERATION_FROM_UNRESOLVABLE | Cannot perform the operation from a path that does not exist |
OPERATION_PATH_ILLEGAL_ARRAY_INDEX | Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index |
OPERATION_VALUE_OUT_OF_BOUNDS | The specified index MUST NOT be greater than the number of elements in the array |
undefined
s (JS to JSON projection)As undefined
type does not exist in JSON, it's also not a valid value of JSON Patch operation. Therefore jsonpatch
will not generate JSON Patches that sets anything to undefined
.
Whenever a value is set to undefined
in JS, JSON-Patch methods generate
and compare
will treat it similarly to how JavaScript method JSON.stringify
(MDN) treats them:
If
undefined
(...) is encountered during conversion it is either omitted (when it is found in an object) or censored tonull
(when it is found in an array).
See the ECMAScript spec for details.
MIT
FAQs
Starcounter-Jack's JSON-Patch with additional capabilities
We found that json-patch-extended demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.